How to Make a Calculator with JavaScript?
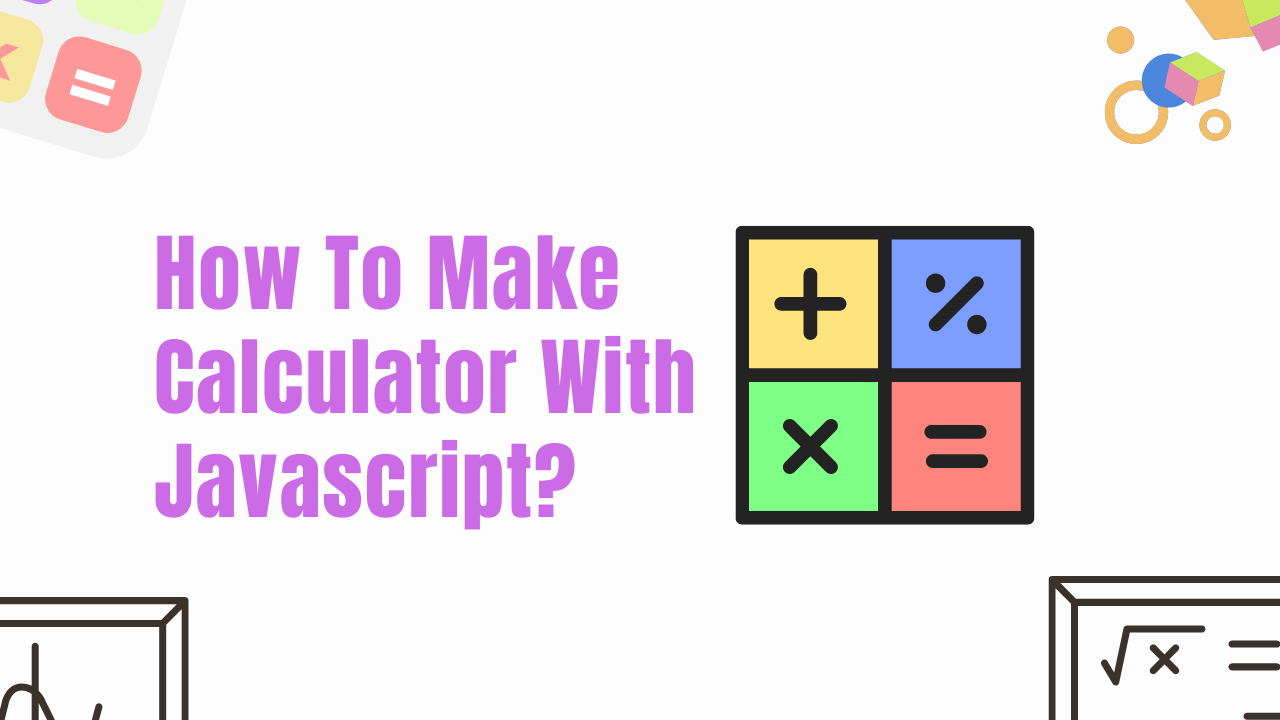
Are you tired of using the same old calculator that comes with your computer or phone? Building your own calculator with JavaScript can be a fun and rewarding experience. Not only will you learn about JavaScript, but you’ll also have a personalized calculator that you can use for all your calculations. In this article, we’ll guide you through the steps of building a calculator with JavaScript.
HTML for Calculator Design
First, let’s start with the HTML code for the calculator. We’ll create a form that contains input fields for the numbers and buttons for the operations.
hsp
<input type="text" id="num1">
<input type="text" id="num2">
<br>
<button onclick="add()">+</button>
<button onclick="subtract()">-</button>
<button onclick="multiply()">*</button>
<button onclick="divide()">/</button>
<button onclick="clear()">C</button>
</form>
As you can see, we’ve added input fields for two numbers and buttons for addition, subtraction, multiplication, division, and clear.
JavaScript for Calculator Functionality
Now, let’s move on to the JavaScript code that will make our calculator functional. We’ll create functions for basic operations and display the result in a separate input field.
ini
function add() {
var num1 = parseFloat(document.getElementById("num1").value);
var num2 = parseFloat(document.getElementById("num2").value);
var result = num1 + num2;
document.getElementById("result").value = result;
}
function subtract() {
var num1 = parseFloat(document.getElementById("num1").value);
var num2 = parseFloat(document.getElementById("num2").value);
var result = num1 - num2;
document.getElementById("result").value = result;
}
function multiply() {
var num1 = parseFloat(document.getElementById("num1").value);
var num2 = parseFloat(document.getElementById("num2").value);
var result = num1 * num2;
document.getElementById("result").value = result;
}
function divide() {
var num1 = parseFloat(document.getElementById("num1").value);
var num2 = parseFloat(document.getElementById("num2").value);
var result = num1 / num2;
document.getElementById("result").value = result;
}
function clear() {
document.getElementById("num1").value = "";
document.getElementById("num2").value = "";
document.getElementById("result").value = "";
}
In these functions, we’re retrieving the values of the input fields and performing the basic operations. We’re also displaying the result in a separate input field with the id “result”.
Additional Features
To make our calculator even more useful, we can add some additional features. For example, we can add a decimal point button that allows us to input decimal values. We can also handle errors such as dividing by zero or inputting non-numeric values. We can even add a backspace button to delete the last input.
clojure
function decimal() {
var num1 = document.getElementById("num1").value;
var num2 = document.getElementById("num2").value;
if (num1.indexOf(".") == -1) {
document.getElementById("num1").value += ".";
}
if (num2.indexOf(".") == -1) {
document.getElementById("num2").value += ".";
}
}
function error() {
var num1 =parseFloat(document.getElementById("num1").value);
var num2 = parseFloat(document.getElementById("num2").value);
if (isNaN(num1) || isNaN(num2)) {
alert("Please enter valid numbers");
}
else if (num2 == 0) {
alert("Cannot divide by zero");
}
}
function backspace() {
var num1 = document.getElementById("num1").value;
var num2 = document.getElementById("num2").value;
document.getElementById("num1").value = num1.slice(0, -1);
document.getElementById("num2").value = num2.slice(0, -1);
}
Styling the Calculator
To make our calculator look more attractive, we can add some CSS styles. We can customize the colors, fonts, and sizes of the buttons and input fields.
cssform {
background-color: #f2f2f2;
border: 1px solid #ccc;
padding: 10px;
max-width: 300px;
margin: 0 auto;
}
input[type="text"], button {
font-size: 20px;
margin: 5px;
padding: 5px;
border: 1px solid #ccc;
border-radius: 5px;
}
button {
background-color: #4CAF50;
color: white;
}
button:hover {
background-color: #3e7e3;
cursor: pointer;
}
Testing and Debugging
Once we’ve completed the JavaScript code and CSS styles, it’s time to test our calculator. We can input various numbers and operations and check if the result is correct. If we encounter any errors, we can use the browser’s console to debug the code and fix the errors.
FAQs
What is JavaScript?
JavaScript is a programming language that is used to create interactive websites and web applications. It is commonly used for adding dynamic effects, validating forms, and creating animations.
Do I need any special software to build a calculator with JavaScript?
No, you can use any text editor such as Notepad or Sublime Text to write the code. You can also use online code editors such as CodePen or JSFiddle.
Can I add more operations to the calculator?
Yes, you can add more operations such as square root, exponent, or percentage. You just need to create new functions for these operations and add buttons tothe HTML code.
Can I customize the design of the calculator?
Yes, you can customize the design with CSS styles. You can change the colors, fonts, and sizes of the buttons and input fields.
Why should I build my own calculator with JavaScript?
Building your own calculator with JavaScript is a great way to learn about the language and also have a personalized calculator that you can use for all your calculations. It also allows you to add additional features and customize the design according to your preferences.
Conclusion
In this article, we’ve learned how to build a calculator with JavaScript. We started with the HTML code for the calculator design and then moved on to the JavaScript code for the functionality. We also added additional features such as decimal point, error handling, and a backspace button. Finally, we styled the calculator with CSS and tested it for any errors. By following these steps, you can create your own personalized calculator that you can use for all your calculations.