How to Make a Calculator with JavaScript?
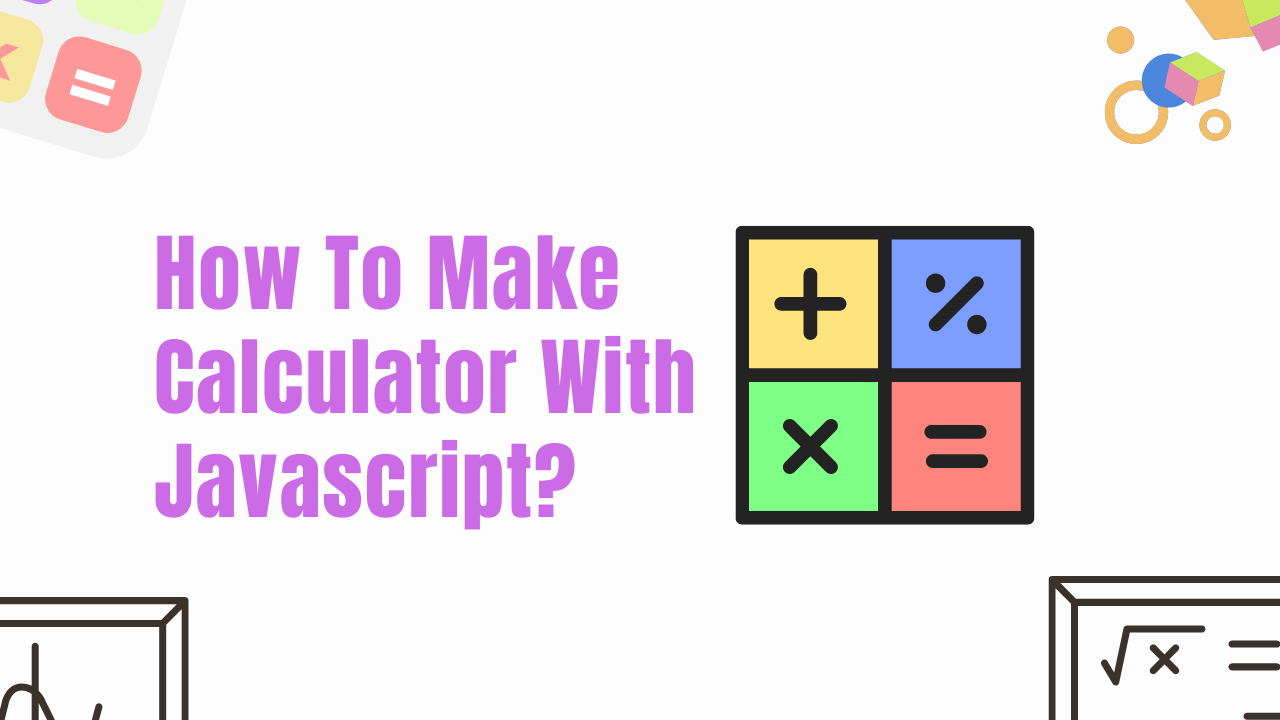
Now, let’s move on to the JavaScript code that will make our calculator functional. We’ll create functions for basic operations and display the result in a separate input field.
ini
function add() {
var num1 = parseFloat(document.getElementById("num1").value);
var num2 = parseFloat(document.getElementById("num2").value);
var result = num1 + num2;
document.getElementById("result").value = result;
}
function subtract() {
var num1 = parseFloat(document.getElementById("num1").value);
var num2 = parseFloat(document.getElementById("num2").value);
var result = num1 - num2;
document.getElementById("result").value = result;
}
function multiply() {
var num1 = parseFloat(document.getElementById("num1").value);
var num2 = parseFloat(document.getElementById("num2").value);
var result = num1 * num2;
document.getElementById("result").value = result;
}
function divide() {
var num1 = parseFloat(document.getElementById("num1").value);
var num2 = parseFloat(document.getElementById("num2").value);
var result = num1 / num2;
document.getElementById("result").value = result;
}
function clear() {
document.getElementById("num1").value = "";
document.getElementById("num2").value = "";
document.getElementById("result").value = "";
}
In these functions, we’re retrieving the values of the input fields and performing the basic operations. We’re also displaying the result in a separate input field with the id “result”.