Building RESTful APIs with Java Spring Boot: A Step-by-Step Tutorial
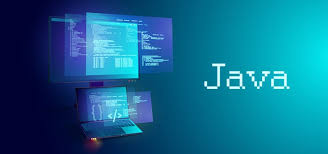
Building RESTful APIs with Java Spring Boot: A Step-by-Step Tutorial
In today’s fast-paced digital world, building RESTful APIs has become an essential skill for developers. Whether you’re creating a web application, mobile app, or any other software that communicates over the web, understanding how to design and implement RESTful APIs is crucial. This tutorial will guide you through the process of building RESTful APIs using Java Spring Boot, a powerful and popular framework that simplifies API development.
Java Spring Boot is a framework that streamlines the development of Java applications, particularly web-based applications. It provides a robust foundation for building scalable and maintainable APIs. The framework embraces the principles of convention over configuration, allowing developers to focus on writing business logic rather than spending time on configuration details.
Setting Up Your Development Environment
Before diving into API development, it’s important to set up your development environment. Follow these steps to ensure a smooth development process:
- Install Java Development Kit (JDK): Download and install the latest version of JDK compatible with Java Spring Boot.
- Install Integrated Development Environment (IDE): Choose a reliable IDE like IntelliJ IDEA or Eclipse to facilitate coding and debugging.
- Install Spring Boot: Use tools like Spring Initializr or command-line tools to create a new Spring Boot project.
- Dependencies and Libraries: Spring Boot offers a wide range of dependencies and libraries that you can easily include in your project to enhance functionality. Research and include the ones that suit your project’s needs.
Creating Your First RESTful Endpoint
Let’s start by creating a simple RESTful endpoint to understand the basics of Spring Boot API development.
- Defining a Controller: Create a new Java class and annotate it with
@RestController
. This annotation indicates that the class will handle incoming HTTP requests and produce HTTP responses. - Mapping Endpoints: Use the
@RequestMapping
annotation to map the URL path to the appropriate method in your controller class. - Implementing Endpoint Logic: Inside the mapped method, write the logic to process the request and generate a response.
public class ApiController {
public String helloWorld() {
return "Hello, world!";
}
}
Designing Effective Endpoints
When designing endpoints, consider these best practices:
- Use Nouns in URLs: Use nouns instead of verbs in your URLs to represent resources. For example, use
/users
instead of/getUsers
. - HTTP Methods: Utilize appropriate HTTP methods like GET, POST, PUT, and DELETE to perform different operations on resources.
- Versioning: Include version numbers in your API URLs to ensure backward compatibility as your API evolves.
Handling Data with Spring Boot
To create dynamic APIs, you’ll often need to handle data. Spring Boot makes this process straightforward:
- Creating Data Models: Define Java classes that represent your data entities, and annotate them with
@Entity
. - Setting Up Repositories: Use Spring Data JPA to create repositories for your data models, allowing you to perform database operations effortlessly.
- CRUD Operations: Spring Boot provides built-in support for CRUD (Create, Read, Update, Delete) operations using repository methods.
Error Handling and Validation
Robust APIs handle errors gracefully and validate incoming data. Spring Boot offers tools to manage these aspects:
- Global Exception Handling: Implement a global exception handler to capture and process exceptions, providing meaningful error responses.
- Input Validation: Utilize validation annotations like
@NotNull
and@Valid
to ensure that incoming data meets the required criteria.
Security and Authentication
Securing your APIs is paramount. Spring Boot simplifies security implementation:
- Spring Security: Integrate Spring Security to manage authentication, authorization, and protection against common security threats.
- Token-based Authentication: Implement token-based authentication using JSON Web Tokens (JWT) to secure your endpoints.
Performance Optimization
To ensure optimal performance, consider these strategies:
- Caching: Implement caching mechanisms to store frequently accessed data and reduce database queries.
- Pagination: Use pagination to limit the amount of data returned in each response, enhancing API performance.
FAQs
Q: Can I use other Java frameworks to build RESTful APIs?
A: Yes, while Spring Boot is a popular choice, other frameworks like Jersey and Play Framework can also be used.
Q: Is Spring Boot suitable for microservices architecture?
A: Absolutely. Spring Boot’s modular structure and cloud integration make it an excellent choice for building microservices.
Q: How can I secure my Spring Boot APIs against common attacks?
A: Implement security measures like input validation, authentication, and authorization using Spring Security.
Q: What’s the role of Maven or Gradle in Spring Boot projects?
A: Maven and Gradle are build automation tools that manage project dependencies and simplify the build process.
Q: Can I deploy Spring Boot applications to the cloud?
A: Yes, Spring Boot provides seamless integration with cloud platforms like AWS, Azure, and Google Cloud.
Q: Is Spring Boot suitable for beginners in API development?
A: Absolutely. Spring Boot’s intuitive design and extensive documentation make it beginner-friendly.
Conclusion
Building RESTful APIs with Java Spring Boot empowers developers to create robust and efficient web services. This tutorial has provided a comprehensive overview, from setting up the development environment to implementing advanced features like security and optimization. By following these step-by-step instructions, you’re well on your way to becoming proficient in RESTful API development. Keep experimenting, learning, and building to enhance your skills and create innovative APIs that cater to modern web application needs.
============================================
SOURCEBAE: Hire Java Developers