Garbage Collection in Java: Understanding and Optimizing Memory Management
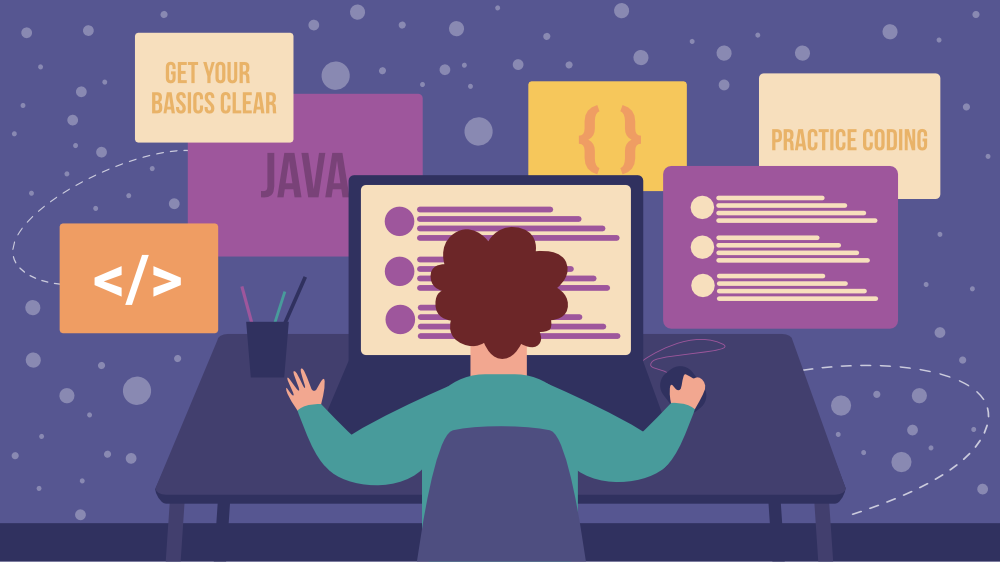
Garbage Collection in Java: Understanding and Optimizing Memory Management
Welcome to a comprehensive guide on “Garbage Collection in Java: Understanding and Optimizing Memory Management.” As a Java programmer, it’s crucial to have a deep understanding of how memory management works, especially in a language like Java, which handles memory automatically through its garbage collection mechanism. In this article, we will delve into the core concepts of garbage collection, explore memory optimization techniques, and provide you with invaluable insights to enhance your Java programming skills.
In the world of Java programming, efficient memory management is essential to prevent memory leaks and optimize application performance. Garbage collection, often referred to as GC, is the process of automatically identifying and reclaiming memory that is no longer in use by the program. This prevents memory leaks and allows developers to focus on coding rather than manual memory management.
Key Concepts in Garbage Collection
1. Memory Management in Java
Memory management is a critical aspect of Java programming. The Java Virtual Machine (JVM) is responsible for allocating and deallocating memory to different objects. Understanding the memory structure and how objects are stored and managed is fundamental to efficient Java programming.
2. Garbage Collection Algorithms
Java employs various garbage collection algorithms, each with its strengths and weaknesses. These include:
- Serial Garbage Collector: Suitable for single-threaded applications.
- Parallel Garbage Collector: Utilizes multiple threads for improved performance.
- Concurrent Mark-Sweep (CMS) Collector: Minimizes application pause times.
- G1 Garbage Collector: Optimized for large heap sizes and reduced pause times.
3. Object Reachability
Garbage collection identifies unreachable objects that can be safely deleted. Objects are categorized into four levels of reachability: strongly reachable, softly reachable, weakly reachable, and phantom reachable. Understanding these levels helps in managing memory more effectively.
4. Finalization
Java allows objects to perform cleanup operations before they are garbage collected through the “finalize()” method. However, relying solely on finalization is discouraged due to its unpredictable nature.
Memory Optimization Techniques
1. Object Reuse
Creating new objects consumes memory and impacts performance. Reusing objects whenever possible by resetting their states can significantly reduce memory overhead.
2. Local Variables and Scopes
Minimize the scope and lifetime of variables. Local variables are automatically deallocated when they go out of scope, aiding in efficient memory management.
3. Nullify References
Manually setting object references to null when they are no longer needed can help the garbage collector identify and clean up memory more effectively.
4. Use of Data Structures
Choosing the right data structures is vital. Some structures, like weak references and reference queues, can assist in better memory management.
FAQs
How does Garbage Collection work in Java?
Garbage Collection in Java involves identifying objects that are no longer reachable and freeing up the memory they occupy. The JVM utilizes various algorithms to perform this task efficiently.
Can I force the Garbage Collection process?
While Java allows you to request garbage collection using “System.gc()”, there’s no guarantee that the JVM will immediately perform it. The JVM decides when to initiate garbage collection based on factors like memory usage.
Is manual memory management possible in Java?
Java emphasizes automatic memory management through garbage collection to prevent common memory-related issues. Manual memory management is not a standard practice in Java and is error-prone.
What is the impact of inefficient garbage collection?
Inefficient garbage collection can lead to memory leaks, where unused objects accumulate and consume memory over time. This can result in application crashes or performance degradation.
How can I measure the performance of Garbage Collection?
Tools like Java VisualVM and Java Mission Control provide insights into garbage collection performance, helping you identify bottlenecks and optimize memory usage.
Can I choose a specific Garbage Collector for my application?
Yes, Java allows you to select a garbage collector based on your application’s requirements. Different garbage collectors are suitable for various scenarios, such as low-latency applications or throughput-focused systems.
Conclusion
Mastering the art of Garbage Collection in Java is essential for creating robust and high-performance applications. By understanding the inner workings of memory management, exploring various garbage collection algorithms, and implementing memory optimization techniques, you can elevate your Java programming skills to new heights. Embrace the power of automatic memory management and let Java’s garbage collection mechanism work its magic while you focus on creating exceptional software.
============================================
SOURCEBAE: Hire Java Developers