Get Familiar With AWS SDK In .NET Core
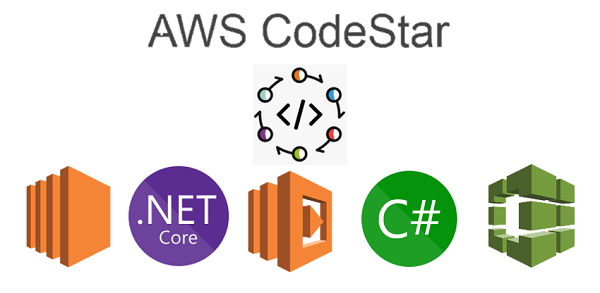
Get Familiar With AWS SDK In .NET Core
In the rapidly evolving landscape of cloud computing, Amazon Web Services (AWS) stands as a leader, providing a wide array of services and tools for developers. For .NET developers, AWS SDK in .NET Core offers a seamless integration between your applications and AWS services. Whether you’re new to AWS or looking to expand your expertise, this guide will take you through the essentials, techniques, and best practices. Let’s embark on a journey to Get Familiar With AWS SDK In .NET Core!
Get Familiar With AWS SDK In .NET Core
AWS SDK in .NET Core is a set of libraries, tools, and resources that enable .NET developers to interact with AWS services efficiently. This integration empowers developers to harness the capabilities of AWS cloud services directly within their .NET applications. By utilizing AWS SDK in .NET Core, developers can seamlessly manage resources, implement security, and utilize AWS services without leaving their familiar .NET environment.
Setting Up AWS SDK in .NET Core
Installing and configuring the AWS SDK in .NET Core is the first step towards leveraging its capabilities. To start, you need to install the necessary NuGet packages that correspond to the AWS services you intend to use. These packages include AWS SDK libraries that provide methods and classes to interact with AWS services seamlessly.
To configure AWS credentials, you’ll need to set up access keys, which consist of an access key ID and a secret access key. These credentials authenticate your application with AWS services. It’s crucial to manage these credentials securely, avoiding hardcoding them within your codebase. Instead, use AWS Identity and Access Management (IAM) roles and policies to grant your application the necessary permissions.
Basic Operations with AWS SDK
Once you’ve set up the AWS SDK in .NET Core and configured your credentials, you’re ready to perform basic operations with AWS services. Let’s take a look at creating an S3 bucket and performing object uploads and downloads.
Creating an S3 Bucket
Amazon Simple Storage Service (S3) provides scalable storage in the cloud. To create an S3 bucket using the AWS SDK in .NET Core, you can use the AmazonS3Client
class. Simply instantiate the client, and then call the PutBucketAsync
method to create a new bucket.
var s3Client = new AmazonS3Client();
var bucketName = "my-unique-bucket-name";
var request = new PutBucketRequest
{
BucketName = bucketName,
UseClientRegion = true
};
await s3Client.PutBucketAsync(request);
Uploading and Downloading Objects
Uploading and downloading objects to and from an S3 bucket is a common task. You can achieve this using the TransferUtility
class provided by the AWS SDK in .NET Core.
var transferUtility = new TransferUtility(s3Client);
// Uploading an object
var filePath = "path/to/myfile.txt";
var key = "myfile.txt";
await transferUtility.UploadAsync(filePath, bucketName, key);
// Downloading an object
var downloadPath = "downloaded/myfile.txt";
await transferUtility.DownloadAsync(downloadPath, bucketName, key);
Working with AWS Services
AWS SDK in .NET Core supports a wide range of AWS services, each tailored to specific use cases. Let’s explore how to integrate with Amazon DynamoDB and utilize Amazon Simple Queue Service (SQS).
Integrating with Amazon DynamoDB
Amazon DynamoDB is a managed NoSQL database service. Using AWS SDK in .NET Core, you can interact with DynamoDB tables using the AmazonDynamoDBClient
class. Here’s an example of inserting an item into a DynamoDB table:
var dynamoDBClient = new AmazonDynamoDBClient();
var request = new PutItemRequest
{
TableName = "MyTable",
Item = new Dictionary<string, AttributeValue>
{
{ "Id", new AttributeValue { S = "123" } },
{ "Name", new AttributeValue { S = "John Doe" } }
}
};
await dynamoDBClient.PutItemAsync(request);
Utilizing Amazon SQS
Amazon SQS is a reliable and scalable message queue service. With the AWS SDK in .NET Core, you can use the AmazonSQSClient
class to send and receive messages from SQS queues.
var sqsClient = new AmazonSQSClient();
// Sending a message
var sendMessageRequest = new SendMessageRequest
{
QueueUrl = "my-queue-url",
MessageBody = "Hello from AWS SDK in .NET Core"
};
await sqsClient.SendMessageAsync(sendMessageRequest);
// Receiving a message
var receiveMessageRequest = new ReceiveMessageRequest
{
QueueUrl = "my-queue-url"
};
var messages = await sqsClient.ReceiveMessageAsync(receiveMessageRequest);
foreach (var message in messages.Messages)
{
Console.WriteLine("Received message: " + message.Body);
}
Security Best Practices
Ensuring the security of your AWS resources and data is of paramount importance. AWS SDK in .NET Core provides tools and practices to enhance security. Let’s delve into Identity and Access Management (IAM) and encryption best practices.
Identity and Access Management (IAM)
IAM allows you to control access to AWS services and resources securely. By creating IAM roles, you can assign permissions to your .NET applications without exposing sensitive credentials. This follows the principle of least privilege, ensuring that applications have only the permissions they absolutely need.
Encryption and Key Management
Encrypting data at rest and in transit is a crucial security measure. AWS SDK in .NET Core offers encryption options for various services. Amazon Key Management Service (KMS) provides centralized management of encryption keys. You can use KMS to encrypt data within your application and manage encryption keys securely.
Advanced Techniques with AWS SDK
As you become more proficient with AWS SDK in .NET Core, you can explore advanced techniques to optimize performance and achieve complex scenarios. Let’s examine asynchronous programming and cross-region replication.
Asynchronous Programming with AWS SDK
Utilizing asynchronous programming techniques can significantly enhance the responsiveness and efficiency of your .NET applications. AWS SDK in .NET Core supports asynchronous methods, enabling your application to perform non-blocking operations.
var s3Client = new AmazonS3Client();
// Asynchronous upload
await s3Client.UploadAsync(filePath, bucketName, key);
// Asynchronous download
await s3Client.DownloadAsync(downloadPath, bucketName, key);
Cross-Region Replication
Cross-region replication allows you to replicate data across different AWS regions. This provides redundancy and disaster recovery capabilities. With AWS SDK in .NET Core, you can configure cross-region replication for S3 buckets.
var s3Client = new AmazonS3Client();
var request = new PutBucketReplicationRequest
{
BucketName = sourceBucketName,
Role = "arn:aws:iam::123456789012:role/replication-role",
ReplicationConfiguration = new ReplicationConfiguration
{
Rules = new List<ReplicationRule>
{
new ReplicationRule
{
Prefix = "prefix/",
Destination = new ReplicationDestination
{
BucketArn = "arn:aws:s3:::destination-bucket"
}
}
}
}
};
await s3Client.PutBucketReplicationAsync(request);
Monitoring and Debugging
Effective monitoring and debugging are essential to maintaining the health and performance of your AWS-integrated .NET applications. AWS SDK in .NET Core offers integration with Amazon CloudWatch for monitoring and troubleshooting.
AWS CloudWatch Integration
Amazon CloudWatch provides insights into the operational health of your AWS resources. With AWS SDK in .NET Core, you can publish custom metrics, set up alarms, and retrieve metrics data programmatically.
var cloudWatchClient = new AmazonCloudWatchClient();
var request = new PutMetricDataRequest
{
Namespace = "MyApp",
MetricData = new List<MetricDatum>
{
new MetricDatum
{
MetricName = "Requests",
Unit = StandardUnit.Count,
Value = 1
}
}
};
await cloudWatchClient.PutMetricDataAsync(request);
Troubleshooting Common Issues
While working with AWS SDK in .NET Core, you might encounter challenges such as connectivity issues or authentication problems. AWS provides detailed documentation and troubleshooting guides to help you address these issues effectively.
Scaling and Performance Optimization
Scalability and performance are vital considerations when developing applications with AWS SDK in .NET Core. By following best practices, you can ensure your applications can handle increased workloads and deliver optimal performance.
Autoscaling Applications on AWS
Autoscaling allows your application to automatically adjust its capacity based on demand. AWS SDK in .NET Core supports autoscaling with Amazon EC2 instances. You can define scaling policies to add or remove instances based on predefined conditions.
Caching Strategies for Improved Performance
Implementing caching strategies can significantly enhance the performance of your applications. AWS SDK in .NET Core integrates seamlessly with Amazon ElastiCache, which is a managed in-memory data store. By caching frequently accessed data, you can reduce database load and improve response times.
Serverless Computing with AWS SDK
Serverless computing allows you to build and run applications without managing server infrastructure. AWS SDK in .NET Core enables you to create serverless APIs using AWS Lambda and implement event-driven architectures.
Building Serverless APIs with AWS Lambda
AWS Lambda lets you run code in response to events without provisioning or managing servers. You can use AWS SDK in .NET Core to create Lambda functions and expose them as APIs using Amazon API Gateway.
Event-Driven Architecture
Event-driven architecture promotes loose coupling between components and enables scalable and resilient applications. AWS SDK in .NET Core supports integrating AWS Lambda functions with various event sources, including Amazon S3, Amazon DynamoDB, and Amazon SNS.
Machine Learning Integration with AWS SDK
Leveraging machine learning capabilities in your applications can lead to intelligent insights and predictions. AWS SDK in .NET Core offers integration with Amazon SageMaker, a fully managed machine learning service.
Utilizing Amazon SageMaker for Machine Learning
Amazon SageMaker simplifies the process of building, training, and deploying machine learning models. With AWS SDK in .NET Core, you can use the SageMaker API to create, train, and deploy models without leaving your .NET environment.
Real-time Inference with AWS SDK
Real-time inference involves using trained machine learning models to make predictions on new data as it arrives. AWS SDK in .NET Core enables you to invoke SageMaker endpoints for real-time inference, allowing your application to provide intelligent responses.
CI/CD for AWS SDK in .NET Core
Implementing continuous integration and continuous deployment (CI/CD) practices ensures that your application development and deployment processes are streamlined and automated. AWS SDK in .NET Core integrates seamlessly with AWS CodePipeline for CI/CD workflows.
Automating Deployments with AWS CodePipeline
AWS CodePipeline is a fully managed CI/CD service that automates the build, test, and deployment phases of your application release process. By utilizing AWS SDK in .NET Core, you can define pipelines that automatically deploy changes to your AWS-integrated .NET applications.
Continuous Deployment Best Practices
To ensure successful and reliable deployments, follow best practices such as version control, automated testing, and environment isolation. With AWS SDK in .NET Core and AWS CodePipeline, you can implement these practices to achieve a smooth and efficient deployment process.
Community and Resources
As you explore and expand your knowledge of AWS SDK in .NET Core, you’ll find a vibrant community and various resources to support your learning journey.
AWS Documentation and Tutorials
The official AWS documentation provides comprehensive guides, tutorials, and reference materials for AWS SDK in .NET Core. Whether you’re a beginner or an advanced developer, you can find valuable insights and examples to enhance your skills.
Developer Forums and Support
Engaging with the AWS developer community can provide you with solutions to challenges, tips, and best practices. AWS offers developer forums and support channels where you can ask questions, share experiences, and collaborate with fellow developers.
FAQs
Is AWS SDK in .NET Core suitable for beginners?
Absolutely! AWS SDK in .NET Core provides a user-friendly interface and extensive documentation, making it accessible for developers at all skill levels.
Can I use AWS SDK in .NET Core for mobile app development?
Yes, AWS SDK in .NET Core can be used to integrate AWS services into mobile applications built using .NET technologies.
How does AWS SDK in .NET Core handle security?
AWS SDK in .NET Core offers features like IAM roles and encryption to ensure secure interactions with AWS services.
What are some common use cases for AWS SDK in .NET Core?
Common use cases include building scalable web applications, integrating machine learning, implementing serverless APIs, and more.
Are there any costs associated with using AWS SDK in .NET Core?
While AWS SDK itself is free to use, you may incur charges for the AWS services you utilize within your applications.
How can I stay up-to-date with the latest AWS SDK updates?
Keep an eye on the official AWS SDK for .NET GitHub repository and AWS announcements for updates and new features.
Conclusion
Embarking on the journey to Get Familiar With AWS SDK In .NET Core opens up a world of possibilities for your .NET applications. From seamless integration with AWS services to advanced techniques and best practices, AWS SDK in .NET Core empowers you to create powerful and innovative solutions. As you continue to explore and experiment, remember that the AWS developer community and resources are there to support your growth. So, dive in, experiment, and unlock the full potential of cloud computing for your .NET projects.