How to Create a Sidebar in React JS?
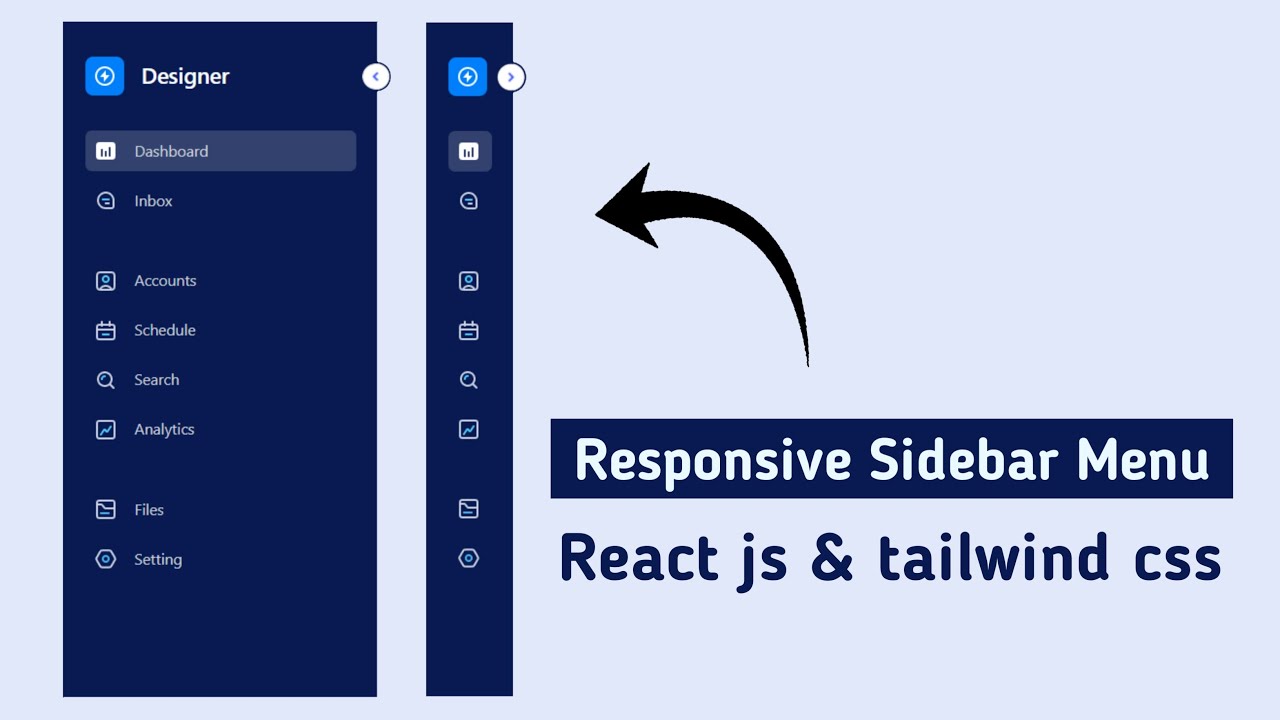
Before we delve into the technical details, let’s understand the role of sidebars in web design. A sidebar is a vertical column typically placed on the left or right side of a web page. It contains links to various sections of the website, allowing users to quickly navigate to different pages or access important features. Sidebars enhance user experience by providing easy access to information without the need for extensive navigation.
Setting Up Your React Project
To begin creating a sidebar in React JS, you first need to set up your React project. If you already have a project in progress, you can skip this step. Otherwise, follow these steps to create a new React application:
- Open your terminal and navigate to the directory where you want to create your project.
- Run the following command to create a new React application:
npx create-react-app sidebar-app
This will create a new directory named “sidebar-app” with all the necessary files.
Designing the Sidebar Component
Now that you have your React project set up, it’s time to design the sidebar component. Here’s how you can do it:
- In your project directory, navigate to the “src” folder and create a new folder named “components” if it doesn’t exist.
- Inside the “components” folder, create a new file called “Sidebar.js”.
- Open “Sidebar.js” and start by importing React:
import React from 'react';
- Create a functional component named “Sidebar”:
function Sidebar() {
return (
<div className="sidebar">
{/* Sidebar content */}
</div>
);
}
export default Sidebar;
In this example, we’ve used a CSS class “sidebar” to style the sidebar component. You can customize the styling according to your project’s design.
Adding Content to the Sidebar
With the basic structure of the sidebar component in place, let’s add some content to it. Typically, a sidebar contains navigation links, icons, and labels. Here’s how you can add content to your React sidebar:
- Inside the
<div className="sidebar">
element, add the navigation links using the<a>
tag:<div className="sidebar">
<a href="#">Home</a>
<a href="#">About</a>
<a href="#">Services</a>
{/* Add more links */}
</div>
- You can also include icons for each link using popular icon libraries like Font Awesome or Material Icons.
Styling the Sidebar
To make your sidebar visually appealing, you’ll need to apply CSS styles. You can do this by creating a separate CSS file or using inline styles. Here’s an example of how you can style your React sidebar using inline styles:
<div className="sidebar" style={{ backgroundColor: '#222', color: '#fff' }}>
{/* Sidebar content */}
</div>
Feel free to experiment with different styles to match your project’s design.
Implementing State for Sidebar Interaction
To enhance the functionality of your sidebar, you can implement state to manage its interaction, such as opening and closing. Here’s a simple example using React hooks:
- Import the
useState
hook at the beginning of your “Sidebar.js” file:import React, { useState } from 'react';
- Declare a state variable to track whether the sidebar is open or closed: