How to Create Route in React JS?
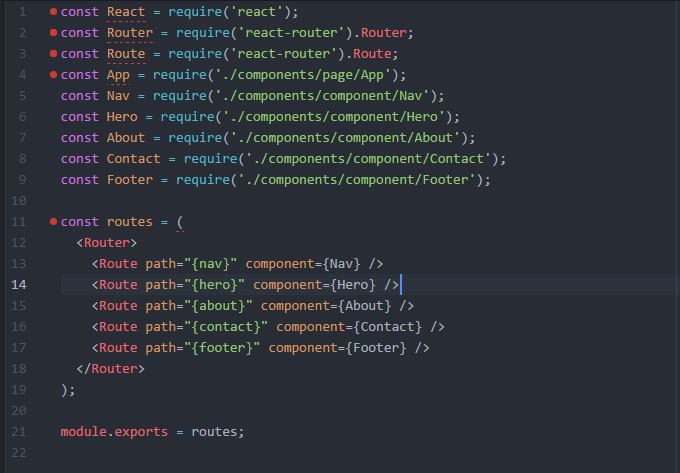
How to Create Route in React JS?
Are you eager to create a dynamic and user-friendly web application using React JS? Routing is a fundamental aspect of web development that allows users to navigate through different pages seamlessly. In this guide, we will explore how to create routes in React JS, providing you with a clear understanding of the process and enabling you to enhance the user experience of your web applications.