How to Enable CORS in React JS?
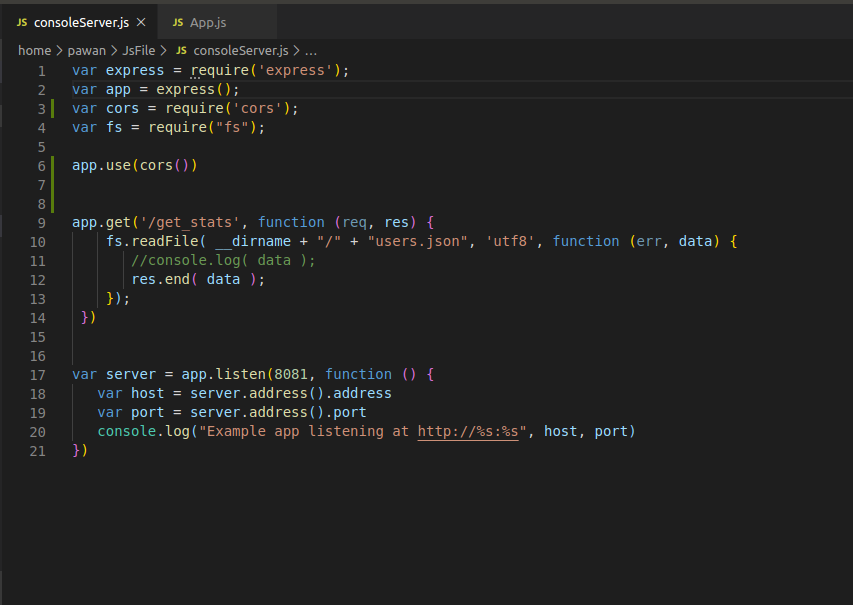
How to Enable CORS in React JS?
In the world of web development, building dynamic and interactive applications has become the norm. React JS, a popular JavaScript library, empowers developers to create responsive user interfaces with ease. However, when it comes to making requests to different servers, you might encounter Cross-Origin Resource Sharing (CORS) issues. In this comprehensive guide, we will walk you through the process of enabling CORS in React JS applications, ensuring seamless communication between your app and external servers.
Cross-Origin Resource Sharing (CORS) is a security feature implemented by web browsers to control and restrict web page access to resources from different origins. When your React app tries to access resources from a different domain, CORS policies may block the request. Here’s how you can enable CORS in your React JS application:
Enabling Cross-Origin Resource Sharing (CORS) in a React.js application involves making changes on the server side, rather than within your React code. CORS is a security feature implemented on the server to control which origins are allowed to access resources.
Here are the general steps to enable CORS for a React.js application:
- Server Configuration:
CORS is a server-side setting. You need to configure your server to allow requests from different origins. This is typically done by adding appropriate HTTP headers to the server’s responses. The exact steps vary depending on the server technology you are using.
For example, if you’re using Express.js in Node.js for your server, you can use the
cors
middleware to enable CORS. Here’s how you might set it up:const express = require('express');
const cors = require('cors');const app = express();
// Allow requests from any origin
app.use(cors());// ... other middleware and routes ...
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
This code allows requests from any origin. In a production scenario, you might want to configure CORS to allow only specific origins.
- Client-Side:
In your React.js application, you don’t need to do anything special to enable CORS. However, you might need to ensure that you’re making requests from the correct origin and that your server is configured to respond correctly to CORS-related headers.
- Testing:
After you’ve made the necessary changes to your server’s CORS configuration, you can test whether CORS is working by making requests from your React app to the server. If CORS is not configured correctly, you might see browser console errors like “Cross-Origin Request Blocked” or similar.
FAQs
How does CORS impact web security?
CORS plays a vital role in web security by preventing unauthorized cross-origin requests. It ensures that only trusted domains can access specific resources, mitigating the risk of data breaches and unauthorized access.
Can I enable CORS for specific HTTP methods?
Yes, you can configure CORS to allow specific HTTP methods for cross-origin requests. This granular control enhances security and restricts potential vulnerabilities.
Are there security risks associated with enabling CORS?
While CORS itself is a security feature, improper configuration can lead to security risks. Allowing unrestricted access from any origin can expose your app to Cross-Site Request Forgery (CSRF) attacks. Always configure CORS carefully.
Is it recommended to use proxy servers for CORS?
Using proxy servers can be an effective solution for handling CORS issues during development. However, in production, it’s essential to ensure proper server-side CORS configuration to maintain security and stability.
How do I troubleshoot CORS errors?
CORS errors can be tricky to troubleshoot. Start by checking your server’s response headers, ensuring they include the necessary CORS headers. Also, inspect browser console logs for detailed error messages.
Can I enable CORS for specific routes?
Yes, you can enable CORS for specific routes by configuring your server to include the appropriate CORS headers based on the route. This selective approach provides flexibility and security.
Conclusion
Enabling CORS in your React JS application is a crucial step to ensure smooth communication between your app and external servers. By understanding CORS policies, implementing proper configuration, and using effective debugging techniques, you can overcome cross-origin issues and build secure, high-performing web applications. Remember to stay up-to-date with best practices in web security and regularly review your CORS settings to maintain a robust and protected application.
READ MORE | HIRE REACT DEVELOPER