How to Fetch API in JavaScript?
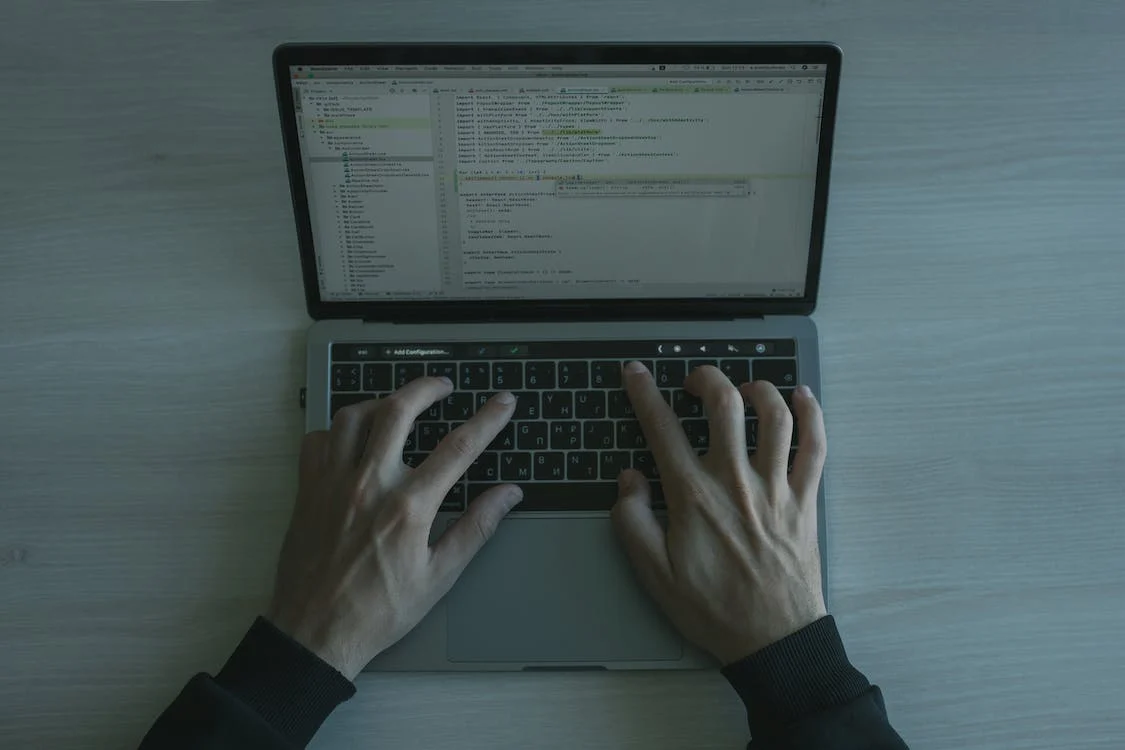
How to Fetch API in JavaScript?
When it comes to building dynamic and interactive web applications, integrating external data through APIs (Application Programming Interfaces) is a common practice. JavaScript, being a versatile and widely used programming language, offers a straightforward and powerful way to fetch data from APIs. In this guide, we will dive deep into the process of how to fetch API in JavaScript. Whether you’re a beginner or an experienced developer, this article will equip you with the knowledge and skills needed to effectively retrieve data from APIs and incorporate it into your web projects.
Understanding Fetch API
In the world of JavaScript, the Fetch API is your go-to tool for making network requests to retrieve resources, often data, from a server. It’s built into modern browsers and provides a more flexible and powerful alternative to the traditional XMLHttpRequest. Fetch API uses Promises, making it easier to work with asynchronous code and manage responses.
Making GET Requests with Fetch
To fetch data from an API, you start with making a GET request. The basic structure of a fetch request involves specifying the URL you want to fetch data from. For instance:
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Here, we use the fetch()
function to initiate the request. The response is then processed as JSON using .json()
, and we handle the data or errors accordingly.
Handling Response Data
Once you receive a response from the API, you can extract and utilize the data as needed. This could involve populating your webpage with content, updating UI elements, or performing calculations. Remember that response data might come in different formats such as JSON, plain text, HTML, or even images.
Error Handling
Error handling is crucial when working with APIs. Network errors, server errors, or incorrect data can all lead to unexpected behavior in your application. By using .catch()
as shown earlier, you can gracefully handle errors and provide feedback to users.
Customizing Headers
Headers provide additional information about your request, such as the format you want the response in or authorization tokens. You can customize headers by passing an object in the fetch()
options. For example:
fetch('https://api.example.com/data', {
headers: {
'Authorization': 'Bearer YOUR_TOKEN'
}
})
Asynchronous Nature of Fetch
Fetching data from APIs is an asynchronous operation, meaning the code execution doesn’t block while waiting for a response. This allows your application to remain responsive and continue executing other tasks.
Dealing with Cross-Origin Requests
Due to security restrictions, web pages can’t make requests to a different domain by default. Fetch API handles Cross-Origin Resource Sharing (CORS) by sending additional headers and options to the server. Servers can also include specific headers to allow or deny requests from certain origins.
Using Promises
Fetch API’s use of Promises simplifies the management of asynchronous operations. Promises help avoid callback hell and allow you to write cleaner and more readable code. The .then()
and .catch()
methods are used to handle resolved and rejected Promises, respectively.
Async/Await
Async/await is a modern syntax that makes asynchronous code look more synchronous and easier to understand. You can rewrite the previous example using async/await like this:
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error:', error);
}
}
Fetching JSON Data
JSON (JavaScript Object Notation) is a common format for API responses. Fetching JSON data is straightforward using the .json()
method, as demonstrated in earlier examples.
Fetching Text Data
If you expect plain text from an API, you can use the .text()
method to extract the response data:
fetch('https://api.example.com/text')
.then(response => response.text())
.then(textData => console.log(textData))
.catch(error => console.error('Error:', error));
Fetching Image Data
Fetching images from APIs is similar to other data types. You can display the fetched image by creating an <img>
element and setting its src
attribute to the fetched URL.
const imgElement = document.createElement('img');
fetch('https://api.example.com/image')
.then(response => response.blob())
.then(imageBlob => {
const imageURL = URL.createObjectURL(imageBlob);
imgElement.src = imageURL;
document.body.appendChild(imgElement);
})
.catch(error => console.error('Error:', error));
Fetching Data from Third-Party APIs
Many APIs, such as weather data or social media feeds, allow you to access their data to enhance your application. To fetch data from third-party APIs, you’ll need to read their documentation and understand how to structure your requests.
Rate Limiting and Throttling
When working with APIs, you may encounter rate limiting, which restricts the number of requests you can make in a given time period. Throttling is a similar concept that limits the rate at which requests are processed. Always respect API usage limits to prevent disruptions in service.
Caching Data for Performance
Caching fetched data can significantly improve the performance of your application. By storing data locally, you can avoid making redundant requests to the server and reduce latency. However, ensure that your cached data stays up to date.
Best Practices for Fetching API Data
- Minimize Requests: Make efficient requests by only fetching the data you need.
- Error Handling: Always handle errors to prevent crashes and provide a smooth user experience.
- Use HTTPS: Ensure your requests and responses are secure by using HTTPS.
- Consistent Data Format: Follow a consistent data format for responses, whether it’s JSON or other types.
- Testing and Monitoring: Regularly test your API calls and monitor for any changes in the API.
Security Considerations
When fetching data from APIs, security is paramount. Avoid exposing sensitive information in your requests and always validate and sanitize the data you receive. Additionally, keep your API keys and tokens secure to prevent unauthorized access.
FAQs
Q: Can I fetch data from any API using JavaScript?
A: In general, yes. However, some APIs may require authentication or have specific usage restrictions.
Q: Is Fetch API supported in all browsers?
A: Fetch API is supported in most modern browsers, including Chrome, Firefox, Safari, and Edge. However, for older browsers, you might need to use a polyfill.
Q: What is the advantage of using async/await with Fetch API?
A: Async/await makes asynchronous code more readable and easier to manage, especially when dealing with multiple API calls.
Q: How do I handle pagination when fetching large amounts of data?
A: APIs often implement pagination by providing next
and previous
links in the response. You can use these links to fetch additional data as needed.
Q: Can I fetch data from APIs that require API keys?
A: Yes, you can include your API key in the headers of your fetch request to access APIs that require authentication.
Q: Are there any performance considerations when fetching data from multiple APIs?
A: Fetching data from multiple APIs can impact performance. Consider using techniques like batching and caching to optimize performance.
Conclusion
Mastering the art of fetching APIs in JavaScript opens up endless possibilities for creating dynamic and data-driven web applications. In this guide, we’ve covered the fundamentals of using Fetch API, making requests, handling responses, and dealing with various data formats. By following best practices and considering security aspects, you can confidently integrate external data into your web projects. Remember, practice makes perfect. So, roll up your sleeves, experiment with different APIs, and watch your applications come to life with real-time data.
READ MORE | How to Become a React Developer?