How to Use For Loop in React JS
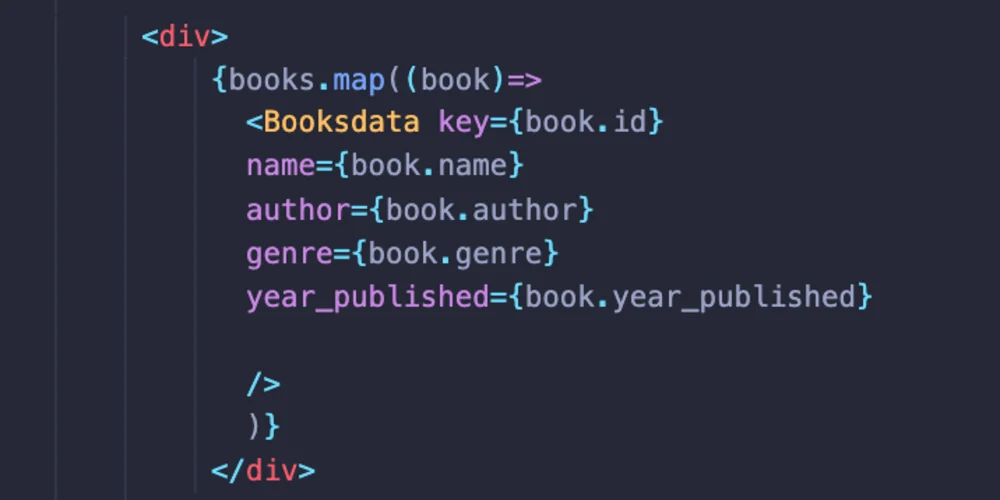
How to Use For Loop in React JS
React JS, a popular JavaScript library, offers powerful tools for building dynamic and interactive user interfaces. One fundamental technique is the use of for loops, which allow developers to iterate through arrays and execute specific tasks for each element. In this guide, we will delve into the various ways of using for loops in React JS, explore best practices, and provide practical examples to enhance your understanding.
For loops are essential constructs in programming that enable you to iterate over arrays and perform actions on each item. In React JS, for loops can be used to render lists of components, manipulate data, or execute other logic for each element in an array.
Rendering Lists of Components
One common use case for for loops in React JS is rendering lists of components. This is particularly useful when you have an array of data and you want to display a React component for each item in the array.
const items = ['Apple', 'Banana', 'Orange'];
const FruitList = () => {
return (
<ul>
{items.map((fruit, index) => (
<li key={index}>{fruit}</li>
))}
</ul>
);
};
Manipulating Data
For loops can also be employed to manipulate data within an array. Suppose you have an array of numbers and you want to double each value using a for loop.
const numbers = [1, 2, 3, 4, 5];
for (let i = 0; i < numbers.length; i++) {
numbers[i] *= 2;
}
Performing Conditional Actions
Using for loops, you can perform conditional actions based on certain criteria. Let’s say you have an array of scores and you want to determine whether each student passed or failed based on a threshold.
const scores = [85, 90, 72, 60, 95];
const passingThreshold = 70;
for (let i = 0; i < scores.length; i++) {
const status = scores[i] >= passingThreshold ? 'Passed' : 'Failed';
console.log(`Student ${i + 1}: ${status}`);
}
Best Practices for Using For Loops in React JS
While for loops offer flexibility, it’s important to follow best practices to ensure efficient and maintainable code.
- Use
map
andforEach
: Instead of traditional for loops, consider using themap
andforEach
methods for arrays. They offer more concise syntax and can enhance code readability. - Key Prop for Rendering: When rendering components using for loops, provide a unique
key
prop to each component. This helps React efficiently update the DOM when the array changes. - Avoid Direct DOM Manipulation: While using for loops to update elements, avoid direct manipulation of the DOM. Instead, modify the state or props of components to trigger re-renders.
- Extract Logic: If the logic within the for loop becomes complex, consider extracting it into separate functions or components. This improves code organization and maintainability.
FAQs (Frequently Asked Questions)
How can I iterate over an object’s properties using a for loop in React JS?
In React JS, objects don’t have a built-in map
method like arrays. To iterate over an object’s properties, you can use a for…in loop. Here’s an example:
const student = {
name: 'John',
age: 20,
grade: 'A'
};
for (const key in student) {
console.log(`${key}: ${student[key]}`);
}
Can I use a for loop to fetch data from an API in React JS?
Yes, you can use a for loop to fetch data from an API in React JS. However, it’s recommended to use asynchronous operations like fetch
or axios
within a useEffect
hook to avoid blocking the main thread.
How do I break out of a for loop if a certain condition is met?
You can use the break
statement to exit a for loop prematurely when a certain condition is met. For example:
const numbers = [10, 20, 30, 40, 50];
for (let i = 0; i < numbers.length; i++) {
if (numbers[i] === 30) {
console.log('Number found!');
break;
}
}
What’s the difference between map
and forEach
in React JS?
Both map
and forEach
are array methods used to iterate over arrays. However, map
returns a new array with the results of applying a provided function to each element, while forEach
only executes the provided function for each element without creating a new array.
Is it possible to nest for loops in React JS?
Yes, you can nest for loops in React JS. However, be cautious when doing so, as nested loops can lead to performance issues. Consider alternative approaches like using nested map
or forEach
methods when working with nested data structures.
Can I use for loops to create animations in React JS?
While for loops are not typically used for creating animations in React JS, you can utilize libraries like React Spring or CSS animations to achieve animations more effectively and with smoother performance.
Conclusion
In this guide, we’ve explored the versatile applications of for loops in React JS. Whether you’re rendering components, manipulating data, or performing conditional actions, for loops provide a powerful mechanism for iterating over arrays. By following best practices and leveraging the strengths of React, you can enhance the efficiency and maintainability of your code. Incorporate the insights from this guide into your React projects to build dynamic and engaging user interfaces.
READ MORE | HIRE REACT DEVELOPER