Using Flask to Build RESTful APIs with Python
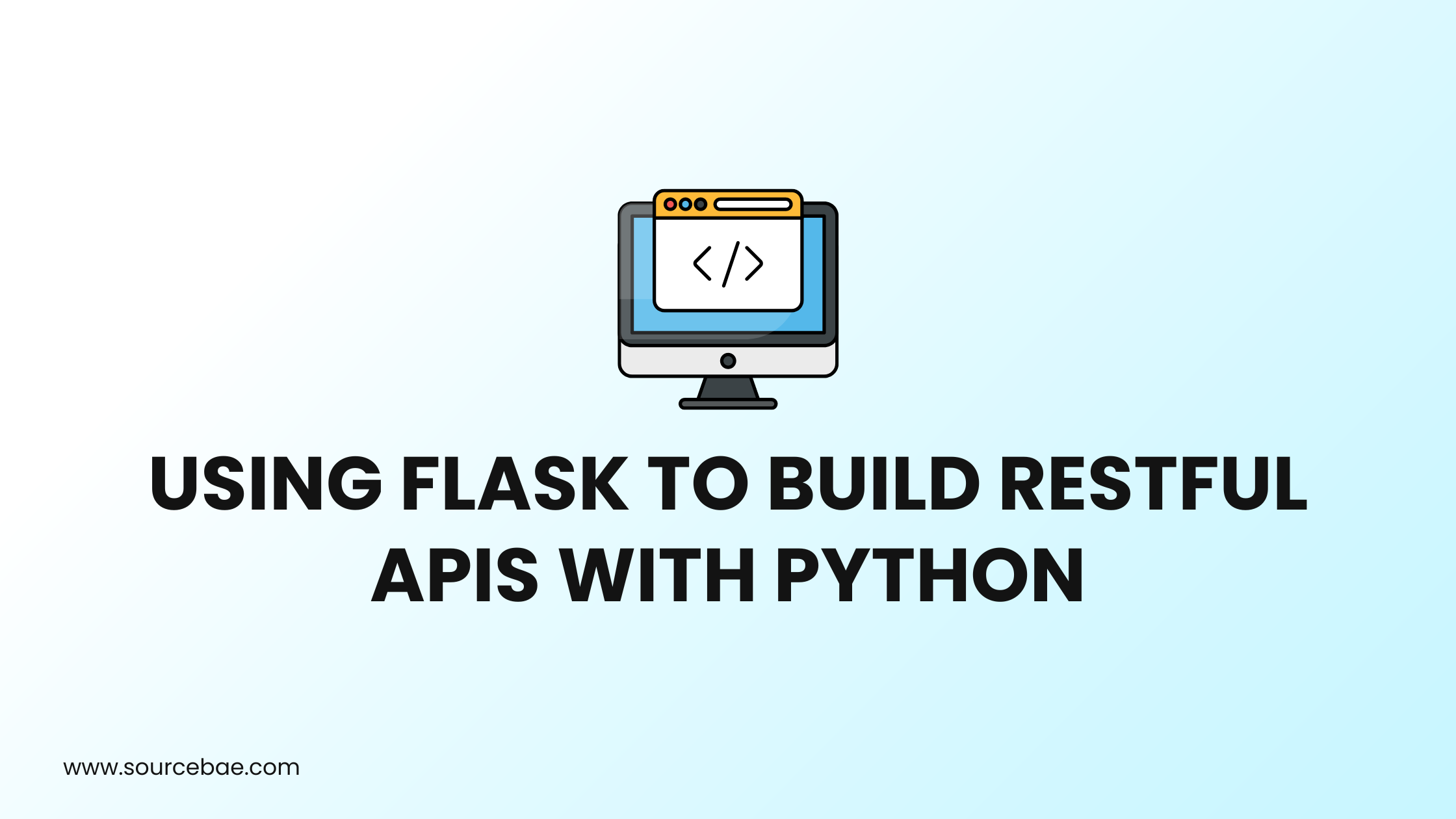
Flask is a lightweight and flexible web framework in Python that allows you to build web applications, including RESTful APIs.
RESTful APIs follow the principles of Representational State Transfer (REST), which is an architectural style for designing networked applications.
This style focuses on using standard HTTP methods (GET, POST, PUT, DELETE) to perform CRUD (Create, Read, Update, Delete) operations on resources.
Here’s a step-by-step guide to building RESTful APIs using Flask:
Step 1: Installation
Make sure you have Python installed on your system. You can install Flask using pip, a package manager for Python. Open your terminal and run:
pip install Flask
Step 2: Setting Up the Flask App
Create a new directory for your project and navigate to it in the terminal. Inside the directory, create a new Python file (e.g., app.py
). Import Flask and create an instance of it:
from flask import Flask
app = Flask(__name__)
Step 3: Defining Routes
Routes define the URLs at which your API will be accessible. You can use decorators to associate functions with specific routes and HTTP methods. For example:
@app.route('/api/resource', methods=['GET'])
def get_resource():
# Logic to fetch and return the resource
return jsonify({"message": "Resource retrieved successfully"})
Step 4: Handling HTTP Methods
Define functions for each HTTP method you want to support. Here’s an example for creating a resource using the POST method:
@app.route('/api/resource', methods=['POST'])
def create_resource():
# Logic to create a new resource
return jsonify({"message": "Resource created successfully"})
Step 5: URL Parameters
You can include parameters in your URLs to provide additional information. Here’s an example of using URL parameters to retrieve a specific resource:
@app.route('/api/resource/<int:resource_id>', methods=['GET'])
def get_specific_resource(resource_id):
# Logic to retrieve the specified resource
return jsonify({"message": f"Retrieved resource {resource_id}"})
Step 6: Running the App
At the end of your app.py
file, add the following lines to run the Flask app:
if __name__ == '__main__':
app.run(debug=True)
Step 7: Testing the API
Start the Flask development server by running python app.py
in your terminal. Your API will be accessible at http://127.0.0.1:5000/
.
You can test your API using tools like curl
, Postman, or even a web browser.
Remember, this is just a basic overview of building RESTful APIs with Flask. Depending on your project’s complexity, you might need to implement authentication, database integration, error handling, and more.
Flask’s simplicity and flexibility make it a great choice for building RESTful APIs in Python. Good luck with your API development!