What Are the Best Practices for Writing React Js Code?
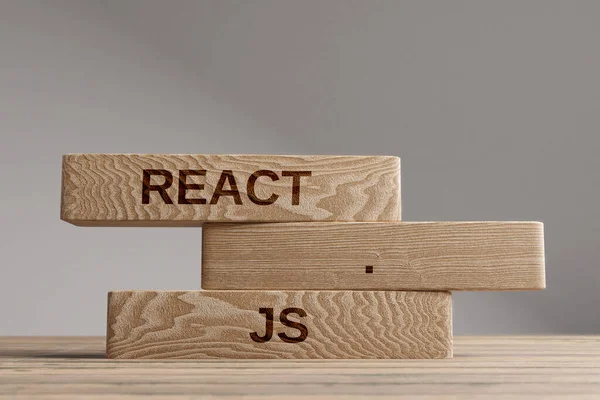
What Are the Best Practices for Writing React Js Code?
In the fast-evolving world of web development, React.js has emerged as a go-to library for building user interfaces. Its flexibility and efficiency have made it a popular choice among developers. However, to make the most of React.js, it’s essential to follow best practices when writing code. In this comprehensive guide, we will explore the key principles and techniques for writing clean and efficient React.js code.
Before we dive into the best practices, let’s start with a brief introduction to React.js for those who might be new to it.
React.js is an open-source JavaScript library for building user interfaces. Developed and maintained by Facebook, it allows developers to create interactive and dynamic web applications with ease. React.js is known for its component-based architecture, which promotes code reusability and maintainability.
Now, let’s explore the best practices for writing React.js code that will help you create robust and scalable applications.
The Fundamentals
1. Understand React’s Component Lifecycle
To become proficient in React.js, it’s crucial to grasp the component lifecycle. Understanding the lifecycle methods such as componentDidMount
and componentWillUnmount
will enable you to manage your components efficiently.
2. Component Structure
Organize your code by creating smaller, reusable components. Keep each component focused on a specific task or feature, following the Single Responsibility Principle (SRP).
3. Use Functional Components
With the introduction of React Hooks, functional components have become the preferred choice. They are more concise and easier to test than class components.
4. Props and State
Maintain a clear distinction between props and state. Props are used for passing data to child components, while state should be reserved for managing component-specific data.
5. Avoid Direct State Mutations
To prevent unexpected side effects, always use the setState
method to update state instead of modifying it directly.
Writing Clean and Maintainable Code
6. Consistent Code Formatting
Adopt a consistent code formatting style, such as Airbnb’s JavaScript Style Guide. Tools like ESLint and Prettier can help enforce coding standards.
7. Code Comments
Document your code using meaningful comments. Explain complex logic or any non-obvious decisions to make your codebase more accessible to other developers.
8. DRY (Don’t Repeat Yourself) Principle
Identify duplicated code and refactor it into reusable functions or components to reduce redundancy and improve maintainability.
9. PropTypes
Utilize PropTypes to define the expected data types of props, helping catch bugs during development.
10. Error Boundaries
Implement error boundaries to gracefully handle and display errors without crashing the entire application.
Performance Optimization
11. Virtual DOM
Leverage React’s Virtual DOM to minimize DOM manipulation, resulting in improved performance.
12. PureComponent and React.memo
Optimize rendering by using PureComponent for class components and React.memo for functional components when unnecessary renders can be avoided.
13. Key Prop
When rendering lists, always provide a unique key
prop to help React identify which items have changed, improving list rendering efficiency.
Testing
14. Unit Testing
Write unit tests for your components using testing libraries like Jest and React Testing Library. Test-driven development (TDD) can help catch issues early.
15. Integration Testing
Perform integration tests to ensure that different components work together seamlessly.
State Management
16. Redux
Consider using Redux for state management in larger applications to maintain a single source of truth.
17. Context API
For smaller applications, the Context API can be a simpler alternative to manage global state.
Security
18. Avoiding XSS Attacks
Sanitize user input and avoid rendering raw HTML to prevent cross-site scripting (XSS) attacks.
Accessibility
19. ARIA Roles and Labels
Use ARIA roles and labels to enhance the accessibility of your application for users with disabilities.
SEO Optimization
20. Server-Side Rendering (SSR)
Implement SSR for better SEO rankings by ensuring search engines can crawl and index your content.
21. Meta Tags
Include relevant meta tags in your HTML to improve the visibility of your web pages in search engine results.
Error Handling
22. Error Logging
Implement error logging to monitor and track issues in your application, making it easier to identify and resolve problems.
Code Reviews
23. Conduct Regular Code Reviews
Encourage a culture of code reviews within your team to identify and address issues early in the development process.
Performance Monitoring
24. Use Profiling Tools
Leverage profiling tools like React DevTools to identify performance bottlenecks and optimize your application.
Stay Updated
25. Keep Learning
The world of web development is constantly evolving. Stay updated with the latest React.js features and best practices by following official documentation, blogs, and online courses.
FAQs
Q: What Are the Best Practices for Writing React Js Code?
A: The best practices for writing React.js code include understanding the component lifecycle, using functional components, maintaining clean code formatting, and optimizing performance, among others. This guide covers these practices in detail.
Q: Why is Code Formatting Important in React.js?
A: Consistent code formatting is essential for code readability and maintainability. It helps developers understand the codebase and ensures that coding standards are followed throughout the project.
Q: When Should I Use Redux for State Management?
A: Redux is suitable for managing state in larger applications with complex data flow and interactions. For smaller projects, the Context API may be a more straightforward choice.
Q: How Can I Improve the Accessibility of My React Application?
A: To improve accessibility, use ARIA roles and labels, provide alt text for images, and ensure keyboard navigation is seamless. Conduct accessibility audits to identify and address issues.
Q: What Is Server-Side Rendering (SSR) in React?
A: Server-Side Rendering (SSR) is a technique that renders a React component on the server and sends the fully-rendered HTML to the client. SSR can improve SEO and initial page load times.
Q: What Are Some Common Performance Bottlenecks in React Applications?
A: Common performance bottlenecks in React applications include excessive re-renders, unnecessary component updates, and inefficient data fetching. Profiling tools like React DevTools can help identify and address these issues.
Conclusion
Mastering React.js requires a commitment to learning and following best practices. By understanding the component lifecycle, writing clean and maintainable code, optimizing performance, and staying updated, you can become a proficient React developer. Remember, practice makes perfect, so keep building and refining your React.js skills.
READ MORE: HIRE REACT DEVELOPER