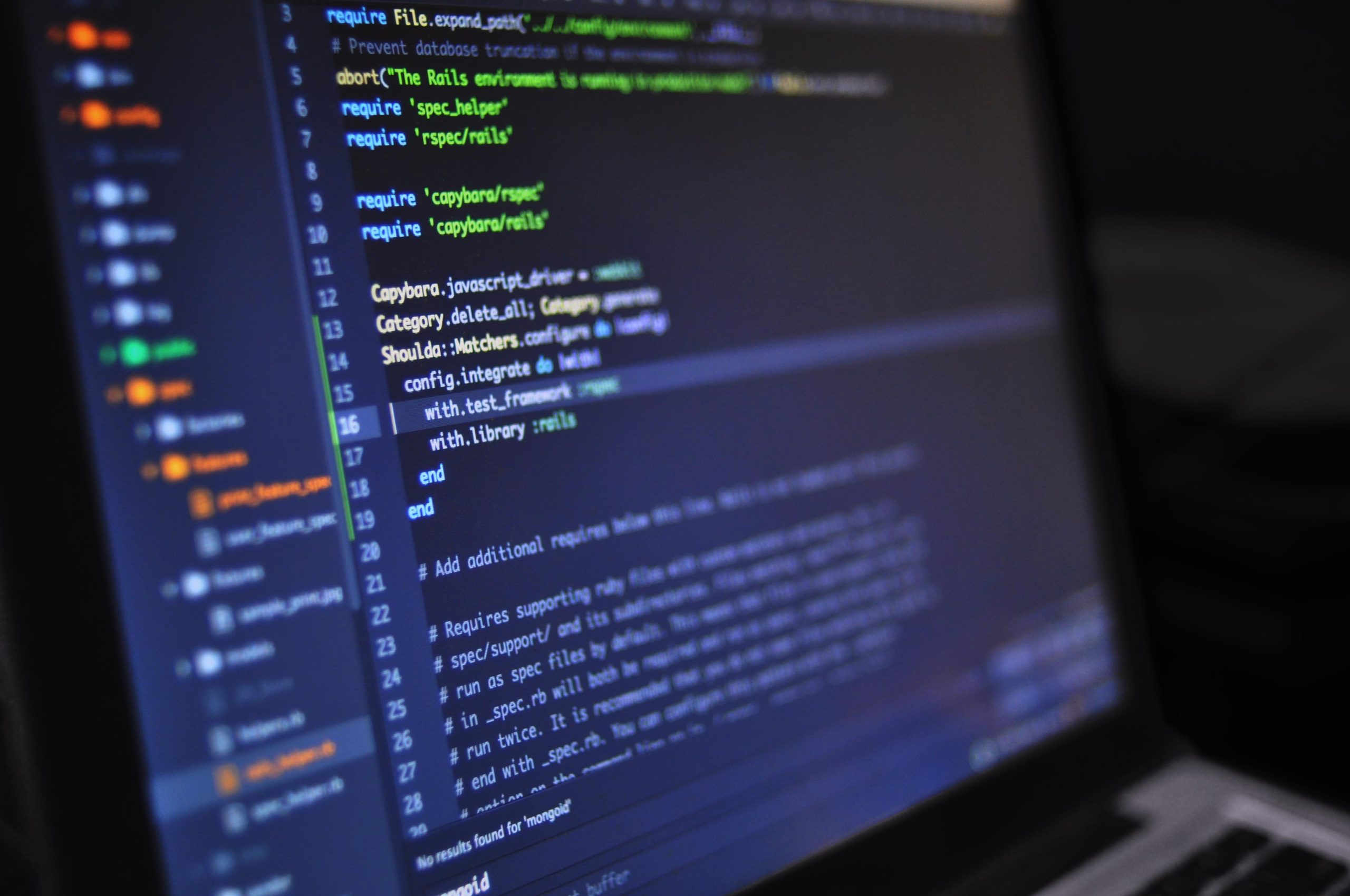
What Is a Single Dimensional Array in Java?
Java, a versatile and widely-used programming language, offers various data structures to developers. One of the fundamental structures is the single dimensional array. In this article, we will delve into the intricacies of single dimensional arrays in Java. From its definition to practical applications, you will gain a comprehensive understanding of this crucial concept. So, let’s embark on this Java journey and uncover the power of single dimensional arrays!
In Java, a single dimensional array is a linear collection of elements, all of the same data type. These elements are stored in contiguous memory locations, making it easy to access and manipulate them. To fully grasp the concept, let’s break it down:
Elements
A single dimensional array consists of elements, which are individual data values. These elements can be of any data type, such as integers, floats, or even objects.
Indexing
Each element in the array is assigned a unique index, starting from 0 for the first element, 1 for the second, and so on. This index is crucial for accessing and modifying array elements.
Fixed Size
Unlike some other data structures, the size of a single dimensional array in Java is fixed upon declaration. You cannot change the size once it’s defined.
Homogeneous
All elements in a single dimensional array must be of the same data type. This homogeneity ensures consistency and efficient storage.
Declaration
To declare a single dimensional array in Java, you specify the data type, followed by the array name and square brackets. For example, to declare an integer array named “myArray,” you would write:
int[] myArray;
Initialization
After declaring an array, you need to initialize it by specifying its size and assigning values to its elements. Here’s an example:
int[] myArray = new int[5];
myArray[0] = 10;
myArray[1] = 20;
myArray[2] = 30;
myArray[3] = 40;
myArray[4] = 50;
Now that we have a foundational understanding of single dimensional arrays, let’s explore their practical applications.
Practical Applications of Single Dimensional Arrays
Single dimensional arrays in Java are incredibly versatile and find applications in various domains:
Storing Lists of Items
Arrays are perfect for storing lists of items of the same data type, such as a list of students’ ages, scores, or product prices.
Iteration
Arrays simplify the process of iterating through a collection of data. You can use loops to access and manipulate each element efficiently.
Sorting
Sorting algorithms often rely on arrays. You can arrange elements in ascending or descending order, making searching and retrieval more efficient.
Mathematical Operations
Arrays are indispensable for mathematical operations. You can perform calculations on elements of an array, such as finding the sum, average, or maximum value.
Data Structures
Arrays are the building blocks for more complex data structures, like lists and queues. They provide a foundation for creating custom data structures.
FAQs (Frequently Asked Questions)
Q: How do I declare and initialize a single dimensional array in Java?
To declare a single dimensional array, specify the data type, followed by the array name and square brackets. For initialization, use the “new” keyword, followed by the data type and the array size. Here’s an example:
int[] myArray = new int[5];
Q: Can I change the size of a single dimensional array after it’s declared?
No, the size of a single dimensional array in Java is fixed upon declaration and cannot be changed.
Q: What is the purpose of indexing in an array?
Indexing in an array allows you to uniquely identify and access each element. It starts from 0 for the first element, 1 for the second, and so on.
Q: Are all elements in a single dimensional array required to be of the same data type?
Yes, all elements in a single dimensional array must be of the same data type. This ensures homogeneity and consistency.
Q: What are some common applications of single dimensional arrays in Java?
Single dimensional arrays are used for storing lists of items, iteration, sorting, mathematical operations, and as building blocks for more complex data structures.
Q: Can I store objects in a single dimensional array?
Yes, you can store objects of the same class in a single dimensional array, provided they share a common data type.
Conclusion
In this comprehensive guide, we’ve explored the world of single dimensional arrays in Java. We’ve covered their definition, key characteristics, practical applications, and answered common questions. As a fundamental data structure in Java, single dimensional arrays play a crucial role in programming tasks. Now that you have a solid understanding of this concept, you can leverage it in your Java projects and write more efficient and organized code.
So, whether you’re a novice programmer or an experienced developer, mastering single dimensional arrays is a step toward becoming proficient in Java programming. Start incorporating them into your projects and unlock the potential of this essential data structure.
READ MORE: Hire React Js Developers