What is Promise in React JS?
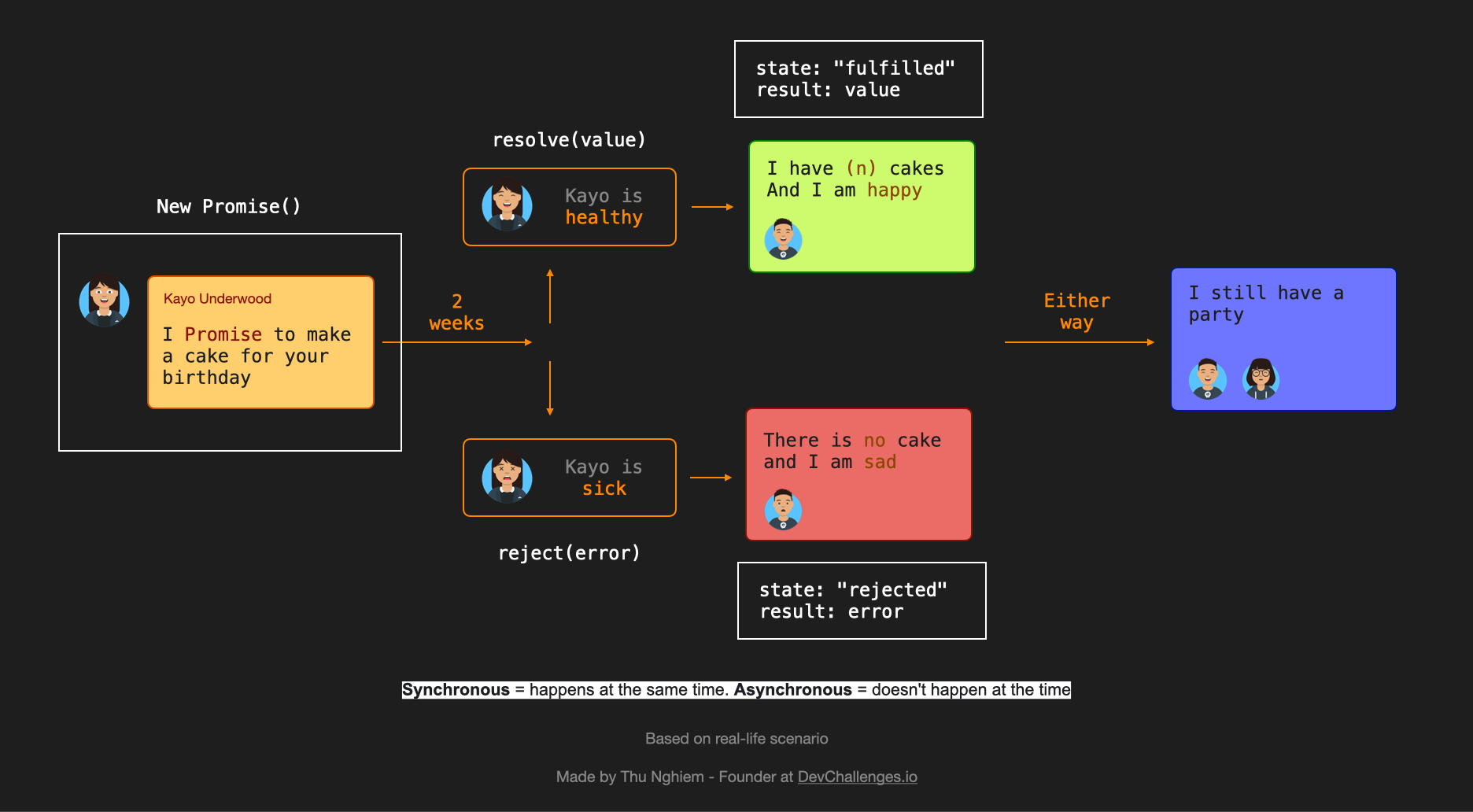
A promise in React JS is an object that represents the eventual completion or failure of an asynchronous operation. It’s a way to deal with operations that don’t necessarily happen immediately but rather at some point in the future. Promises provide a cleaner and more structured approach to handling asynchronous code compared to traditional callback functions.
Promises have three distinct states:
- Pending: The initial state of a promise when it’s created. This is the state where the asynchronous operation hasn’t completed yet.
- Fulfilled: The state of a promise when the asynchronous operation is successfully completed. This is also known as “resolved.”
- Rejected: The state of a promise when the asynchronous operation encounters an error or fails.
Using promises in React JS helps developers avoid callback hell, a situation where deeply nested callbacks can lead to code that’s hard to read, understand, and maintain. Instead, promises enable a more linear and organized flow of asynchronous operations, making the codebase cleaner and more manageable.
How Promises Work in React JS
Promises in React JS work by providing a set of methods that allow you to attach functions to be executed when the promise is fulfilled or rejected. The most common methods used with promises are then()
and catch()
:
- The
then()
method is used to specify what should happen when the promise is fulfilled. It takes a callback function that will be executed when the promise is resolved successfully. - The
catch()
method is used to handle errors that might occur during the promise’s lifecycle. It takes a callback function that will be executed if the promise is rejected.
Let’s take a look at an example to better understand how promises work in React JS:
const fetchData = () => {
return new Promise((resolve, reject) => {
// Simulate an asynchronous operation
setTimeout(() => {
const data = { id: 1, name: 'Example' };
resolve(data); // Fulfill the promise
// reject(new Error('Failed to fetch data')); // Reject the promise
}, 1000);
});
};