What is the Concept of a Class in Java?
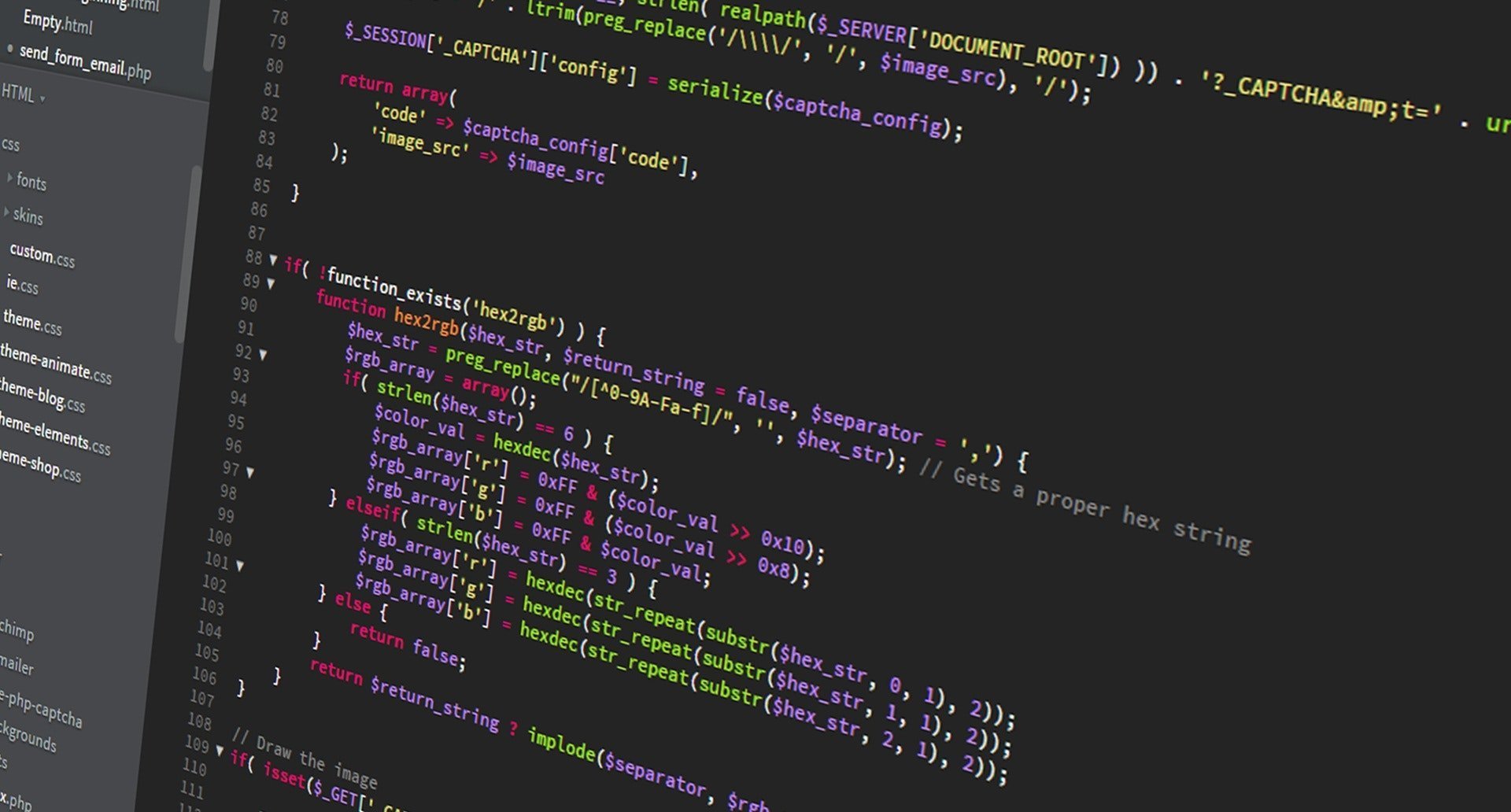
What is the Concept of a Class in Java?
Java, one of the most popular programming languages, is celebrated for its object-oriented programming (OOP) approach. At the core of this methodology lies the concept of a class. To comprehend Java and OOP, it’s pivotal to grasp what a class is and how it functions within the language.
Java is an object-oriented programming language, which means it structures code around objects, combining data and functionality into a single unit known as a class.
Object-oriented programming emphasizes the creation of objects that contain both data and behavior.
Class in Java
In Java, a class is a fundamental concept that serves as a blueprint or template for creating objects. It defines the structure and behavior of objects, specifying their attributes (fields or member variables) and methods (functions or member functions). Classes are a key component of object-oriented programming (OOP), a programming paradigm that promotes the organization of code into objects, each of which encapsulates data and functionality.
Important Aspects of a Class in Java:
- Attributes/Fields: A class can have fields or member variables, which represent the attributes or properties of objects created from that class. Fields can have different data types, including primitive types (e.g., int, double) and reference types (e.g., other classes or objects).
- Methods: A class contains methods, which define the behavior or actions that objects of the class can perform. Methods can manipulate the class’s fields and perform various operations.
- Constructor: Every class can have at least one constructor, which is a special method used to initialize objects when they are created. Constructors have the same name as the class and can have parameters to set initial values for the object’s fields.
- Encapsulation: Java supports encapsulation, which means that you can control access to a class’s fields and methods using access modifiers (e.g., public, private, protected). This helps hide the internal details of a class and expose only what’s necessary for external interaction.
- Inheritance: Java supports inheritance, allowing you to create a new class (subclass or derived class) that inherits fields and methods from an existing class (superclass or base class). Inheritance promotes code reuse and the creation of class hierarchies.
- Polymorphism: Polymorphism is another OOP concept in Java that allows objects of different classes to be treated as objects of a common superclass. This enables more flexible and dynamic coding, often achieved through method overriding and interfaces.
- Abstraction: Abstraction involves simplifying complex systems by modeling classes based on their essential features and ignoring unnecessary details. It helps manage the complexity of large software systems.
Here’s a simplified example of a Java class definition:
public class Car {
// Fields
private String make;
private String model;
private int year;
// Constructor
public Car(String make, String model, int year) {
this.make = make;
this.model = model;
this.year = year;
}
// Method
public void start() {
System.out.println("The car is starting.");
}
// Getter methods (for encapsulation)
public String getMake() {
return make;
}
public String getModel() {
return model;
}
public int getYear() {
return year;
}
}
In this example, the Car
class defines the structure of a car object, including its attributes (make, model, year) and a method (start) to perform a specific action. This class can be used as a blueprint to create individual car objects with their unique characteristics.
Examples of Class Implementation
Let’s create a simple Java class to better understand its structure and usage:
class MyClass {
private String name;
public MyClass(String name) {
this.name = name;
}
public void display() {
System.out.println("Hello, " + name + "!");
}
}
Best Practices for Defining Classes
To ensure effective class implementation, follow best practices like naming conventions, encapsulation, and meaningful abstraction.
Frequently Asked Questions (FAQs)
Q1: What is the main purpose of a class in Java?
A: The primary purpose of a class in Java is to define a blueprint for creating objects, encapsulating data and behavior.
Q2: Can a Java class inherit from multiple classes?
A: No, Java supports single inheritance, meaning a class can inherit from only one superclass.
Q3: How are class constructors different from regular methods?
A: Constructors have the same name as the class, no return type, and are used to initialize object properties during object creation.
Q4: What is the significance of access modifiers in a class?
A: Access modifiers control the visibility and accessibility of class members, ensuring proper encapsulation and security.
Q5: Can you provide an example of polymorphism using Java classes?
A: Polymorphism in Java can be demonstrated through method overriding, where a subclass provides a specific implementation of a method defined in its superclass.
Conclusion
Understanding the concept of a class in Java is foundational to mastering the language and embracing object-oriented principles. Classes provide the structure, organization, and reusability that are vital for efficient and scalable software development.
READ MORE: What is the Introductory Web Development Cost in India?