Career Planning as an Individual Contributor Software Engineer
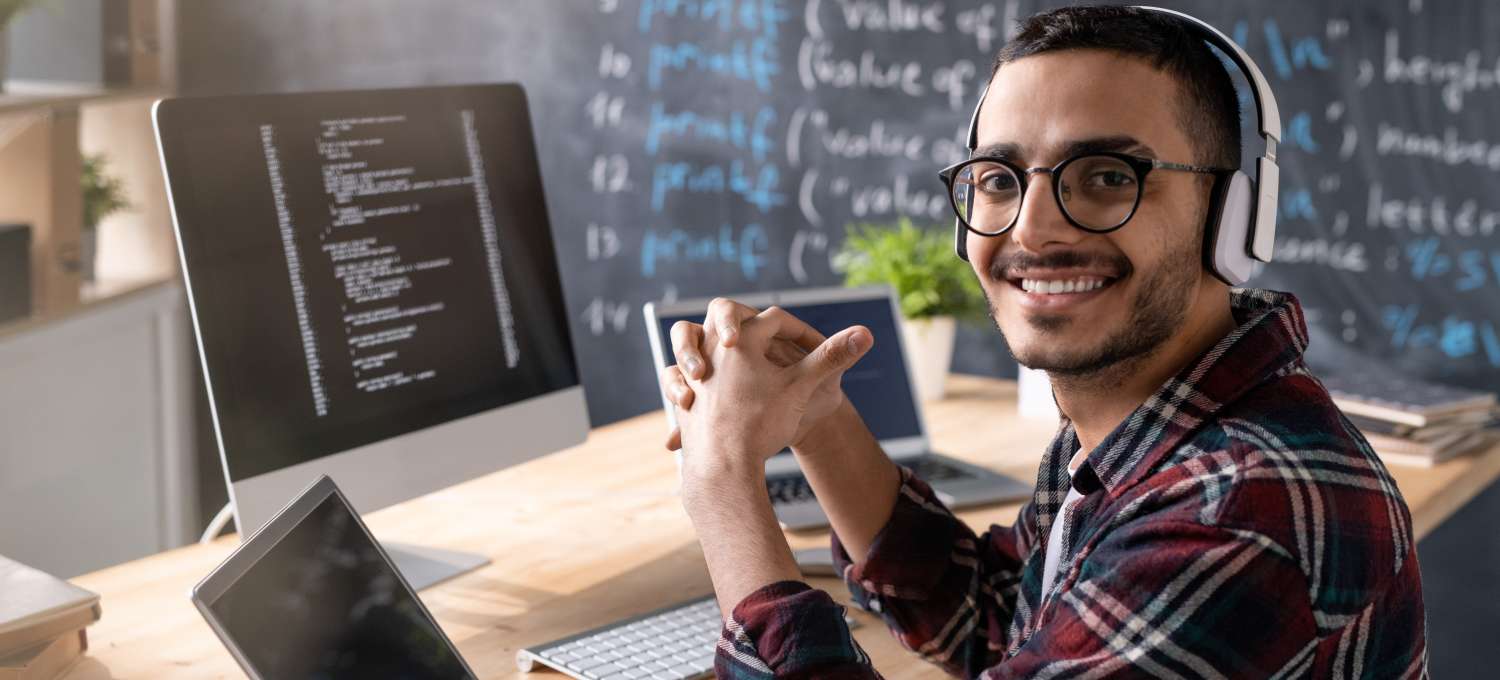
Career Planning as an Individual Contributor Software Engineer
Career planning is a crucial aspect of professional growth for individual contributor software engineers. It involves setting long-term goals, acquiring new skills, and making strategic decisions to advance in your software engineering career. In this article, we will explore the best practices and insights to help you succeed in your journey as an individual contributor software engineer.
As an individual contributor software engineer, your career growth relies heavily on your technical expertise and ability to solve complex problems. To achieve long-term success in this role, consider the following strategies:
1. Assessing Your Skills and Interests
Before embarking on any career plan, it’s essential to evaluate your current skill set and interests. Identify your strengths and weaknesses in various programming languages, tools, and frameworks. Determine the areas that excite you the most and align with your passion. This introspection will help you focus on a specialized path within software engineering that suits your interests.
2. Setting Clear Career Goals
Defining clear and achievable career goals is vital for your growth as an individual contributor software engineer. Set short-term and long-term objectives that align with your skills and interests. Whether it’s becoming an expert in a particular technology or taking on a leadership role in software development, having well-defined goals will guide your career decisions.
3. Creating a Development Plan
Once you’ve identified your career goals, create a development plan to reach them. Outline the necessary steps, including acquiring new technical skills, attending workshops, obtaining certifications, or pursuing higher education. Break down your plan into manageable milestones to measure your progress effectively.
4. Building a Strong Professional Network
Networking plays a significant role in career growth. Connect with fellow software engineers, attend industry events, and participate in online forums to expand your professional network. Building strong relationships with colleagues, mentors, and industry experts can open doors to new opportunities and valuable insights.
5. Staying Updated with Industry Trends
The field of software engineering is dynamic, with continuous advancements in technology. Stay updated with the latest industry trends, best practices, and emerging technologies. Regularly read tech blogs, watch webinars, and participate in online communities to keep your knowledge up-to-date.
6. Seeking Challenging Projects
Taking on challenging projects can significantly contribute to your career growth. Volunteering for complex assignments or working on cross-functional teams can help you enhance your problem-solving abilities and showcase your skills to higher-ups in the organization.
7. Embracing Learning Opportunities
As a software engineer, there are numerous learning opportunities available, such as online courses, workshops, and bootcamps. Embrace these opportunities to acquire new skills or improve existing ones. Continuous learning is essential for staying competitive in the ever-evolving tech industry.
8. Balancing Technical and Soft Skills
While technical skills are crucial, soft skills are equally important for career advancement. Develop strong communication, teamwork, and leadership skills to excel as an individual contributor software engineer. Effective collaboration and communication can lead to successful project outcomes and open doors to leadership roles.
9. Seeking Mentorship and Guidance
Having a mentor can significantly impact your career growth. Seek out experienced software engineers who can provide guidance, offer advice, and share their own career insights. A mentor can help you navigate challenges, identify blind spots, and accelerate your career progression.
10. Creating a Personal Brand
Establishing a personal brand within the software engineering community can set you apart from others. Contribute to open-source projects, write technical blogs, and participate in conferences to showcase your expertise. A strong personal brand can attract exciting career opportunities and recognition within the industry.
11. Evaluating Job Opportunities Wisely
When considering job opportunities, evaluate them carefully based on how well they align with your career goals and values. Look beyond monetary benefits and consider factors like company culture, work-life balance, and growth potential.
12. Taking Risks and Embracing Change
Successful career planning often involves taking calculated risks and embracing change. Be open to new challenges and don’t shy away from stepping out of your comfort zone. Embracing change can lead to significant breakthroughs in your career.
13. Being Adaptable and Resilient
The tech industry is known for its volatility. Being adaptable and resilient in the face of change is crucial. Embrace failure as a learning opportunity and bounce back stronger from setbacks.
14. Giving Back to the Community
Contributing to the software engineering community can be fulfilling and rewarding. Mentor junior engineers, participate in hackathons, or engage in outreach programs. Giving back not only benefits others but also enhances your professional reputation.
15. Recognizing Burnout and Prioritizing Self-Care
Software engineering can be demanding and stressful at times. Recognize signs of burnout and prioritize self-care. Take breaks, practice mindfulness, and maintain a healthy work-life balance to ensure long-term career sustainability.
16. Tracking Your Achievements
Keep a record of your accomplishments, successful projects, and recognition received. This documentation can serve as valuable evidence of your impact during performance reviews or when seeking new opportunities.
17. Seeking Feedback and Continuous Improvement
Request feedback from colleagues, managers, or mentors to understand areas where you can improve. Continuous improvement is essential for professional growth, and constructive criticism can help you identify blind spots and make necessary adjustments.
18. Exploring Different Specializations
Software engineering offers a wide array of specializations, such as web development, machine learning, cybersecurity, and more. Explore different areas to discover your true passion and expertise.
19. Building a Diverse Portfolio
A diverse portfolio showcasing your various projects and accomplishments can strengthen your job applications and professional image. Demonstrate your versatility and adaptability through your portfolio.
20. Networking Beyond Your Industry
Don’t limit your networking to only those within the software engineering industry. Expand your horizons by connecting with professionals from other fields. Cross-industry collaborations can lead to unique opportunities and perspectives.
21. Seeking Leadership Opportunities
Even as an individual contributor software engineer, you can still seek leadership opportunities within projects or teams. Demonstrating leadership qualities can position you for future managerial roles.
22. Negotiating Your Worth
When presented with new job offers or promotions, don’t be afraid to negotiate your salary and benefits. Knowing your worth and advocating for fair compensation is crucial for long-term career satisfaction.
23. Understanding Company Culture
Company culture plays a significant role in your job satisfaction. Understand the values and work environment of potential employers to ensure a good fit for your personality and career goals.
24. Contributing to Open-Source Projects
Participating in open-source projects not only benefits the software community but also enhances your skills and visibility. It can be a stepping stone to new opportunities and collaborations.
25. Balancing Work and Side Projects
If you’re passionate about personal side projects, find a balance between your regular job and these endeavors. Side projects can demonstrate your creativity and entrepreneurial spirit.
FAQs
FAQ 1: What is the significance of career planning for individual contributor software engineers?
Career planning is essential for individual contributor software engineers as it helps them set clear goals, identify their strengths and interests, and make informed career decisions. It provides a roadmap for professional growth and ensures they stay competitive in the dynamic tech industry.
FAQ 2: How can I create a development plan for my software engineering career?
To create a development plan, assess your current skills, define your career goals, and identify the steps required to achieve them. This may involve acquiring new technical skills, attending workshops, obtaining certifications, and participating in online learning platforms.
FAQ 3: Can networking really impact my career as an individual contributor software engineer?
Yes, networking is a powerful tool for career growth. Building a strong professional network can lead to new opportunities, mentorship, and exposure to different perspectives within the industry.
FAQ 4: How can I maintain a healthy work-life balance in the demanding field of software engineering?
Maintaining a work-life balance is crucial for long-term career sustainability. Set boundaries, prioritize self-care, and take regular breaks to prevent burnout and maintain productivity.
FAQ 5: Is it necessary to specialize in a particular area of software engineering?
While specializing in a particular area can enhance your expertise, it’s not always necessary. Exploring different specializations allows you to discover your true passion and expertise, making you a well-rounded software engineer.
FAQ 6: How can I contribute to open-source projects as an individual contributor software engineer?
Contributing to open-source projects can be as simple as finding a project that interests you and starting to contribute through code, documentation, or community engagement. It’s a great way to enhance your skills and give back to the software community.
Conclusion
Career planning as an individual contributor software engineer is a transformative process that requires self-awareness, determination, and a commitment to continuous improvement. By following the strategies outlined in this article, you can chart a successful and fulfilling career path in the dynamic world of software engineering. Remember to stay curious, embrace challenges, and always strive for excellence in your professional journey.
READ MORE: 10 Full Stack Project Ideas for 2023