Mastering Binary Search Trees in Data Structures
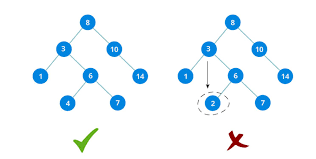
Mastering Binary Search Trees in Data Structures
Are you ready to delve into the realm of data structures and unlock the potential of binary search trees? This comprehensive guide will take you on a journey through the intricacies of mastering binary search trees, providing you with a solid foundation and expert insights into this fundamental topic. Whether you’re a novice programmer or a seasoned developer, by the end of this article, you’ll have a clear understanding of how to leverage binary search trees to optimize search operations and enhance your coding skills.
Binary search trees (BSTs) are a crucial data structure that plays a pivotal role in various computer science applications. At their core, BSTs are hierarchical structures composed of nodes, each containing a value and pointers to its left and right children. The arrangement of nodes in a BST follows a simple yet powerful property: for each node, all values in its left subtree are less than its value, while all values in its right subtree are greater. This property enables efficient search, insertion, and deletion operations, making BSTs an indispensable tool in algorithm design.
The Key Advantages of Binary Search Trees
Binary search trees offer a plethora of advantages that make them an attractive choice for implementing search and retrieval operations:
- Fast Search Operations: Thanks to the hierarchical nature of BSTs, searching for a specific value is significantly faster compared to linear search methods.
- Efficient Insertion and Deletion: Inserting and deleting nodes in a BST can be accomplished with logarithmic time complexity, ensuring optimal performance.
- Ordered Data Storage: The arrangement of values in a BST allows for easy traversal in ascending or descending order, making it suitable for tasks like in-order traversal.
- Dynamic Structure: BSTs can dynamically adapt to changing data, adjusting their shape while maintaining their balanced properties.
- Application Diversity: Binary search trees find applications in databases, compiler design, file systems, and more, showcasing their versatility.
Understanding Binary Tree Traversal Techniques
Traversal is an essential operation when working with binary search trees. It involves visiting each node in the tree exactly once, and there are three primary traversal techniques:
In-Order Traversal: Exploring Sorted Order
In the in-order traversal, nodes are visited in the following sequence: left child, current node, right child. This technique results in values being visited in ascending order, making it ideal for tasks like printing values in sorted order.
Pre-Order Traversal: Capturing Hierarchy
The pre-order traversal starts with the current node, followed by its left and right children. This technique is useful for duplicating the structure of the tree and capturing its hierarchy.
Post-Order Traversal: Deletion and Cleanup
In the post-order traversal, nodes are visited in the order of left child, right child, current node. This technique is commonly used for resource deallocation and memory cleanup.
Implementing Binary Search Trees: Step by Step
Building a binary search tree involves careful consideration of insertion and balancing strategies. Let’s walk through the step-by-step process:
- Insertion: To insert a new value into a BST, begin at the root and traverse the tree based on the value’s comparison with each node’s value. If the value is smaller, move to the left child; if larger, move to the right child. Continue this process until an empty spot is found, then insert the new node.
- Balancing: While binary search trees can offer efficient operations, they can also become unbalanced, leading to degraded performance. Techniques like AVL trees and Red-Black trees are employed to maintain balance, ensuring logarithmic time complexity for search operations.
- Deletion: Deleting a node from a binary search tree requires careful consideration of its children and position in the tree. Several cases need to be handled, including nodes with no children, nodes with one child, and nodes with two children.
Advanced Techniques for Optimal Binary Search Trees
As you master the basics of binary search trees, consider exploring advanced techniques to further enhance your understanding and proficiency:
Self-Balancing Trees: AVL and Red-Black Trees
Self-balancing trees like AVL and Red-Black trees automatically adjust their structure during insertions and deletions to maintain balance. These structures ensure that the height of the tree remains logarithmic, resulting in consistently efficient operations.
B-Trees: Handling Large Datasets
B-Trees are versatile data structures used to manage large datasets, commonly found in databases and file systems. B-Trees maintain balance and ensure efficient access to data, even in scenarios with a high volume of records.
Splay Trees: Optimizing Frequently Accessed Nodes
Splay trees are designed to bring frequently accessed nodes closer to the root, optimizing access times for those nodes. While they may not guarantee balanced structures, their focus on frequently accessed nodes can lead to improved performance.
FAQs about Mastering Binary Search Trees
How can I determine if a given binary tree is a binary search tree?
To determine if a binary tree is a binary search tree, you can perform an in-order traversal and check if the visited values are in ascending order. If the values satisfy this condition, the tree is a binary search tree.
Can a binary search tree have duplicate values?
Yes, a binary search tree can have duplicate values. In such cases, it’s common to decide whether duplicates should be stored in the left subtree, right subtree, or a combination of both, depending on the insertion strategy.
What is the time complexity of searching in a binary search tree?
The time complexity of searching in a binary search tree is O(h), where h is the height of the tree. In a balanced tree, the height is logarithmic, resulting in efficient O(log n) search operations.
How do AVL trees and Red-Black trees ensure balance?
AVL trees and Red-Black trees ensure balance by implementing rotation and color-flipping operations during insertions and deletions. These operations maintain specific balance criteria, preventing the tree from becoming heavily skewed.
Are binary search trees suitable for large datasets?
While traditional binary search trees might not be optimal for large datasets, self-balancing trees like AVL, Red-Black, and B-Trees are well-suited for managing large amounts of data efficiently.
What advantages do self-balancing trees offer over regular binary search trees?
Self-balancing trees offer the advantage of maintaining balance automatically, preventing worst-case scenarios and guaranteeing efficient search operations. Regular binary search trees can become unbalanced, leading to slower searches.
Conclusion: Unlocking Efficiency with Binary Search Trees
Mastering binary search trees is a valuable skill that empowers programmers to optimize search operations and build efficient algorithms. From the foundational principles to advanced techniques, this guide has provided you with a comprehensive overview of binary search trees. As you continue your coding journey, remember that binary search trees are just one of many tools at your disposal. By combining them with your creativity and problem-solving skills, you can tackle a wide range of programming challenges with confidence and precision. So go ahead, embrace the power of binary search trees, and take your coding expertise to new heights!
SOURCEBAE: HIRE REACT DEVELOPER