Beginner’s Guide: Building an Interpreter From Scratch – Step-by-Step Tutorial
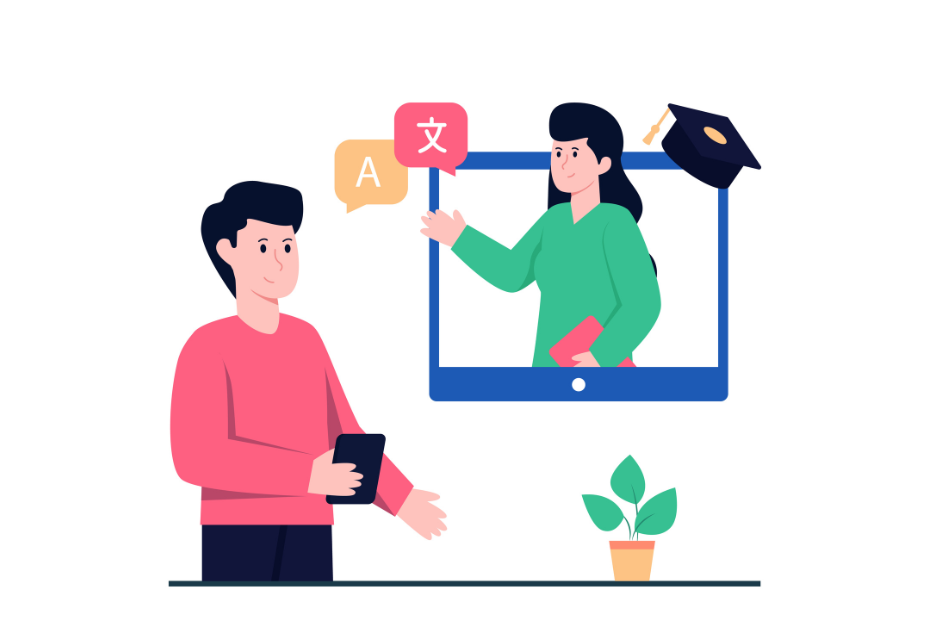
Writing an interpreter from scratch can seem like a daunting task, but with the right approach, determination, and guidance, it can be an immensely rewarding experience. An interpreter is a program that reads and executes code written in a specific programming language. Whether you’re a seasoned developer looking to deepen your understanding or an aspiring programmer taking your first steps, this article will provide you with the knowledge and tools to approach writing an interpreter from scratch confidently.
How to Approach Writing an Interpreter From Scratch?
When embarking on the journey of creating an interpreter from scratch, it’s essential to follow a well-structured approach. Here’s a step-by-step outline to guide you through the process:
Step | Description |
---|---|
Define the Purpose | Clearly understand the purpose and scope of the interpreter you wish to create. Identify the target language and the specific features it should support. |
Gather Requirements | List down the functionalities and capabilities your interpreter must have. Consider factors like input methods, error handling, and performance optimization. |
Research Existing Interpreters | Study other interpreters for the target language or similar languages. Analyze their design and implementation to gain insights and ideas. |
Design the Interpreter | Plan the overall architecture of your interpreter. Determine the data structures, algorithms, and modules needed for smooth execution. |
Lexical Analysis | Implement the lexical analyzer or tokenizer to break the input code into meaningful tokens. |
Syntactic Analysis | Create the parser to form a syntax tree from the tokens generated by the lexer. |
Semantic Analysis | Add semantic checks to ensure that the code adheres to the language rules and identify any errors. |
Intermediate Representation | Develop an intermediate representation to translate the high-level code into a lower-level form for execution. |
Code Generation | Write code to generate machine-level instructions or bytecode from the intermediate representation. |
Implement Built-in Functions | Incorporate essential built-in functions and libraries to provide useful functionalities to the users. |
Error Handling | Implement robust error handling mechanisms to provide informative and helpful error messages to the users. |
Testing | Conduct extensive testing at each stage of development to ensure the interpreter’s correctness and reliability. |
Performance Optimization | Fine-tune your interpreter to enhance its performance and reduce execution time. |
Documentation | Document your code thoroughly to make it easier for others to understand and contribute. |
Developing a Comprehensive Lexer
The lexer is the first crucial component of an interpreter as it is responsible for converting the source code into a stream of tokens. Tokens are the smallest units of code, such as keywords, identifiers, and literals. A well-designed lexer is essential for accurate parsing of the code. Consider these tips when developing your lexer:
- Use Regular Expressions: Regular expressions are powerful tools for pattern matching. Leverage them to define the structure of various tokens in the language.
- Handle Whitespace and Comments: Ensure that your lexer can skip irrelevant whitespace and properly handle comments in the code.
- Error Reporting: Implement meaningful error reporting in the lexer to indicate invalid input or unrecognized symbols.
- Optimize Performance: Lexer performance is critical, especially for large codebases. Employ efficient algorithms and data structures to minimize processing time.
Creating an Efficient Parser
The parser takes the stream of tokens generated by the lexer and converts it into a hierarchical structure, typically an abstract syntax tree (AST). A well-designed parser ensures that the code adheres to the grammar rules of the language. Consider the following when creating your parser:
- Grammar Design: Design a clear and unambiguous grammar for your target language. Use tools like BNF (Backus-Naur Form) or EBNF (Extended Backus-Naur Form) to represent the grammar.
- Recursive Descent Parsing: Consider using recursive descent parsing, which is a top-down parsing technique. Each non-terminal rule in the grammar corresponds to a specific parsing function.
- Error Recovery: Implement error recovery strategies to gracefully handle syntax errors in the code and continue parsing.
- AST Generation: Construct an abstract syntax tree that represents the hierarchical structure of the code. This tree will be used for semantic analysis and code generation.
Implementing Semantic Analysis
Semantic analysis ensures that the code’s meaning is correct according to the language’s rules. It catches errors that can’t be identified during lexical and syntactic analysis. When implementing semantic analysis, keep the following in mind:
- Type Checking: Perform type checking to ensure that operations are performed on compatible data types.
- Variable Scope: Keep track of variable scopes to prevent naming conflicts and enforce proper variable access rules.
- Function Resolution: Resolve function calls and ensure the correct number and types of arguments are passed.
- Constant Folding: Optimize the code by evaluating constant expressions during the compilation phase.
Generating Intermediate Representation
The intermediate representation is an intermediate step between the high-level code and the final machine code or bytecode. It simplifies the translation process and enables optimizations. When generating the intermediate representation:
- Choose a Representation Format: Decide on the format of the intermediate representation, whether it’s stack-based, register-based, or another format.
- Map Language Constructs: Define how high-level language constructs are translated into the intermediate representation.
- Optimize the IR: Apply optimization techniques to the intermediate representation to improve code efficiency.
Code Generation and Execution
Code generation is the process of converting the intermediate representation into machine code or bytecode. Depending on the target platform, the generated code can be executed directly by the computer’s hardware or interpreted by a virtual machine. Key considerations for code generation:
- Target Platform: Understand the target platform and its architecture to generate compatible machine code.
- Optimization: Apply optimization techniques to the generated code to enhance performance.
- Interpretation vs. Compilation: Decide whether your interpreter will directly execute the bytecode or generate machine code for the host system.
Incorporating Built-in Functions and Libraries
To make your interpreter more useful, consider adding built-in functions and libraries that provide commonly used functionalities. These functions can include mathematical operations, string manipulations, file I/O, and more. Ensure that the built-in functions are well-documented and easy to use.
Error Handling and Debugging
Comprehensive error handling is crucial for any interpreter. Ensure that error messages are clear, informative, and point to the source of the error. Additionally, implement debugging features to aid users in understanding and fixing issues in their code.
Extensive Testing and Quality Assurance
Thoroughly test your interpreter to ensure it works correctly under various scenarios. Write unit tests, integration tests, and functional tests to cover different aspects of the interpreter’s functionality. Continuously improve and update the tests as you add new features and make changes.
Conclusion
Writing an interpreter from scratch is a challenging yet immensely rewarding endeavor. With a well-structured approach, a solid understanding of language concepts, and a passion for problem-solving, you can create a powerful interpreter that unlocks the potential of your chosen programming language. Embrace the journey, learn from the process, and enjoy the satisfaction of building something truly remarkable.
============================================
FAQs
Q: What programming languages can I create an interpreter for?
A: You can create an interpreter for almost any programming language, ranging from simple scripting languages to complex high-level languages. The key is to understand the language’s syntax and semantics thoroughly.
Q: Do I need to be an expert programmer to write an interpreter from scratch?
A: While prior programming experience is beneficial, it’s not a strict requirement. Beginners can approach this project with dedication, perseverance, and willingness to learn.
Q: Is writing an interpreter a time-consuming task?
A: Yes, creating an interpreter from scratch is a time-consuming process, particularly for complex languages. However, the experience gained and the insights into language design are invaluable.
Q: Can I use existing libraries or tools to simplify the process?
A: Yes, you can use various tools and libraries that can help with lexing, parsing, and code generation. However, building the core components from scratch offers a deeper understanding of the process.
Q: Are there any resources or tutorials available to help me get started?
A: Yes, there are several online tutorials, books, and open-source projects that provide guidance on creating interpreters. Explore resources specific to your target language for better insights.
Q: Can I build an interpreter for a domain-specific language (DSL)?
A: Absolutely! Interpreters are commonly used for DSLs to define custom syntax and semantics tailored to specific problem domains. It’s an excellent way to simplify complex tasks.
READ MORE: TypeScript vs. JavaScript