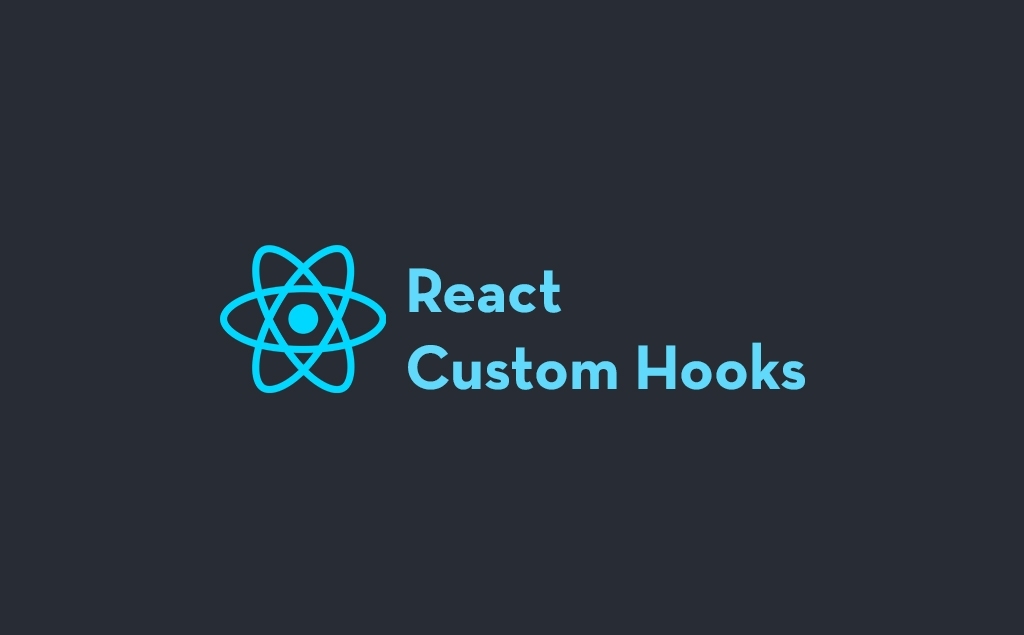
Custom React JS Hooks
React JS is a popular JavaScript library used for building user interfaces. It offers various features, such as components, props, and state management, which make it a robust choice for web developers. One of the most significant advancements in React is the introduction of Custom Hooks, which allows developers to create reusable and efficient logic that can be shared across different components. In this article, we will explore Custom React JS Hooks in detail, discussing their benefits, implementation, and real-world use cases.
What are Custom React JS Hooks?
Custom React JS Hooks are functions that allow you to extract logic from components and reuse it across different parts of your application. These hooks follow the naming convention “useSomething,” where “Something” indicates the functionality they provide. By encapsulating logic into custom hooks, developers can achieve cleaner and more organized code, leading to better maintainability and scalability of React applications.
Advantages of Custom React JS Hooks
- Code Reusability: With Custom Hooks, developers can encapsulate complex logic and share it across multiple components, reducing code duplication and promoting reusability.
- Improved Readability: By abstracting logic into hooks, the main component’s code becomes cleaner and easier to understand, making it more readable and maintainable.
- Separation of Concerns: Custom Hooks enable a clear separation of concerns between the presentation and business logic, resulting in more structured code architecture.
- Testability: Isolating logic in hooks allows for easier testing, as hooks can be unit tested independently from components.
- Enhanced Performance: Custom Hooks can optimize the rendering process and prevent unnecessary re-renders by using React’s memoization techniques.
- Shareable Logic: Custom Hooks can be published and shared with the React community, contributing to a more extensive library of reusable logic for developers.
Implementing Custom React JS Hooks
Creating a custom hook in React is a straightforward process. Custom hooks follow the rules of regular hooks but must start with the keyword “use” to be identified correctly by React. Below is an example of a simple custom hook for handling form inputs:
import { useState } from 'react';
function useFormInput(initialValue) {
const [value, setValue] = useState(initialValue);
const handleChange = (e) => {
setValue(e.target.value);
};
return {
value,
onChange: handleChange,
};
}
In this example, the useFormInput
hook encapsulates the logic for handling form inputs, abstracting away the useState
hook’s implementation details.
Common Custom React JS Hooks
- useLocalStorage: This hook allows you to synchronize and manage data in the browser’s local storage. It is useful for persisting data between sessions or caching user preferences.
- useFetch: The
useFetch
hook simplifies data fetching from APIs by handling the loading, error, and data states efficiently. - useMediaQuery: With this hook, developers can easily adapt the UI to different screen sizes and devices by tracking media query changes.
- useAnimation: The
useAnimation
hook provides control over CSS animations, making it easy to create engaging user interactions. - useDebounce: This hook helps in optimizing performance by delaying the execution of a function until a certain amount of time has passed since the last call.
- useFormValidation: Developers can use this hook to implement form validation easily, providing instant feedback to users during input.
Real-World Use Cases
Building a To-Do List App
Custom React JS Hooks can greatly simplify the development of a To-Do List app. A useTodo
hook could handle tasks like adding, deleting, and marking items as complete. The main component can focus solely on rendering the UI, while the hook manages the underlying data and logic.
Implementing Dark Mode
Creating a Dark Mode feature becomes more manageable with the help of a useDarkMode
hook. This hook could manage the state of the dark mode, handle toggling, and save the user’s preference to local storage.
Internationalization
For applications requiring multiple language support, a useIntl
hook could handle language switching, translation, and context-based content rendering.
FAQs
Can I use multiple Custom Hooks in a single component?
Yes, you can use multiple custom hooks in a single component. Custom Hooks are designed to be composable, allowing you to combine various hooks to address different aspects of your component’s functionality.
Is there any performance concern with Custom React JS Hooks?
Custom Hooks, when used correctly, can actually enhance performance by promoting reusability and memoization. However, improper usage, such as creating infinite loops or incorrect dependencies, may lead to performance issues.
Can I pass props to Custom Hooks?
Yes, you can pass props to Custom Hooks just like regular functions. This allows you to make your hooks more flexible and customizable for different use cases.
Are Custom React JS Hooks a replacement for Redux?
While Custom Hooks can handle state management at a component level, they are not a direct replacement for Redux. Redux is more suitable for managing global state and complex interactions between components.
How can I share my Custom Hooks with the React community?
To share your custom hooks, consider publishing them as separate packages on platforms like npm. Include proper documentation and examples to make them accessible and easy to use for other developers.
Are Custom React JS Hooks officially supported by React?
Yes, Custom Hooks are officially supported by React, and they follow the same rules as regular hooks. However, ensure you are using the latest version of React to access the latest features and optimizations.
Conclusion
Custom React JS Hooks are a powerful tool that can significantly improve the way you build React applications. By promoting code reusability, separation of concerns, and improved readability, these hooks contribute to a more efficient and maintainable codebase. As you dive deeper into React development, consider integrating Custom Hooks into your projects to unlock their full potential.