Data Structures in Python: Exploring the Essentials
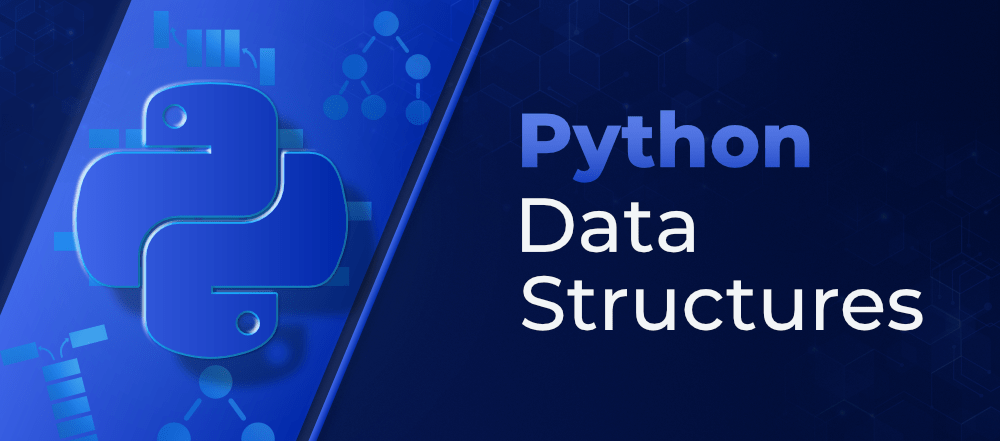
Data Structures in Python: Exploring the Essentials
Data structures are fundamental components in programming that allow us to organize and store data efficiently. In Python, a versatile and user-friendly programming language, various data structures can be employed to handle different types of data and solve a wide array of problems. This article delves into the realm of data structures in Python, providing an in-depth understanding of their concepts, implementations, and applications.
Lists: Versatile Collections
Lists are one of the most basic yet versatile data structures in Python. They allow you to store multiple values of different data types in a single variable. Lists are defined by enclosing elements within square brackets, making them an essential tool for various tasks such as iteration, sorting, and data manipulation.
Dictionaries: Key-Value Mapping
Dictionaries provide a flexible way to store data in a key-value format. Each value is associated with a unique key, enabling efficient retrieval and modification of data. This makes dictionaries a powerful choice for tasks involving data indexing, caching, and data representation.
Tuples: Immutable Sequences
Tuples are similar to lists but with one key difference: they are immutable, meaning their values cannot be changed after creation. This makes tuples suitable for scenarios where data integrity and security are paramount.
Sets: Unordered Collections
Sets are collections of unique elements with no specific order. They are useful for tasks that require checking for membership and removing duplicates from data. Sets can be combined using various set operations like union, intersection, and difference.
Arrays: Homogeneous Data Containers
Python arrays are data structures used to store elements of the same data type in contiguous memory locations. While lists can hold different data types, arrays are more efficient for tasks that require numerical computations and large datasets.
Stacks: Last-In-First-Out (LIFO) Data Structure
A stack is a linear data structure that follows the Last-In-First-Out (LIFO) principle. It’s often visualized as a stack of items, where the last item added is the first to be removed. Stacks are used for tasks like maintaining function calls, undo operations, and expression evaluation.
Queues: First-In-First-Out (FIFO) Data Structure
In contrast to stacks, queues adhere to the First-In-First-Out (FIFO) principle. They are used to manage items in a sequence, where the first item added is the first to be removed. Queues are crucial for tasks like job scheduling, breadth-first search algorithms, and task management.
Linked Lists: Dynamic Data Organization
Linked lists are dynamic data structures comprising nodes, each containing both data and a reference to the next node. Linked lists come in various forms, such as singly linked lists, doubly linked lists, and circular linked lists. They are useful for scenarios requiring constant-time insertions and deletions.
Hash Tables: Efficient Data Retrieval
Hash tables, also known as hash maps, are data structures that enable efficient data retrieval using a hash function. They are ideal for situations where constant-time access to data is crucial, making them valuable for dictionary implementations and caching mechanisms.
Trees: Hierarchical Data Representation
Trees are hierarchical data structures composed of nodes that have parent-child relationships. Trees have diverse applications, including binary search trees for efficient data searching, heaps for priority queue implementations, and decision trees for decision-making processes.
Graphs: Complex Data Relationships
Graphs are collections of nodes connected by edges, representing complex relationships among data points. They are used in diverse domains such as social network analysis, transportation routing, and recommendation systems.
Implementing Data Structures in Python
Creating Lists
To create a list in Python, use square brackets and separate elements with commas.
my_list = [1, 2, 3, 'hello', True]
Building Dictionaries
Dictionaries can be defined using curly braces and key-value pairs.
person = {
'name': 'Alice',
'age': 30,
'city': 'New York'
}
Tuple Creation
Tuples are defined by enclosing values in parentheses.
my_tuple = (1, 2, 3, 'world')
Crafting Sets
Sets can be created using curly braces or the set()
function.
my_set = {1, 2, 3, 4, 5}
Implementing Stacks with Lists
Stacks can be implemented using Python lists. Use the append()
method to push items onto the stack and the pop()
method to remove the top item.
stack = []
stack.append(1) # Push
top_item = stack.pop() # Pop
Queue Implementation with Collections
The collections
module provides the deque
class, which can be used to implement queues efficiently.
from collections import deque
queue = deque()
queue.append(1) # Enqueue
front_item = queue.popleft() # Dequeue
Linked List Creation
Linked lists can be implemented using classes in Python, where each class represents a node containing data and a reference to the next node.
class Node:
def __init__(self, data):
self.data = data
self.next = None
Hash Table Usage
Python’s built-in dict
data type is a form of a hash table, providing quick access to values based on keys.
my_dict = {'a': 1, 'b': 2, 'c': 3}
value = my_dict['b'] # Accessing value using key
Tree Construction
Trees can be implemented using classes to define nodes with parent and child relationships.
class TreeNode:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
FAQs
How do I choose the right data structure for my task?
Selecting the appropriate data structure depends on factors such as the nature of your data, the operations you need to perform, and the efficiency you require. Lists are versatile for general data storage, while dictionaries excel at key-based data retrieval. Consider your specific use case to make an informed decision.
Can I change the size of a tuple after creation?
No, tuples are immutable, meaning their size and elements cannot be changed after creation. If you need a dynamic collection, consider using lists instead.
What is the benefit of using a hash table?
Hash tables offer constant-time average case access to data, making them efficient for scenarios where quick data retrieval is crucial. They are commonly used for implementing dictionaries and caching mechanisms.
How do linked lists differ from arrays?
Linked lists are dynamic data structures with nodes that store both data and references. Arrays are static data structures that store elements of the same type in contiguous memory. Linked lists allow for efficient insertions and deletions, while arrays provide constant-time access to elements.
What are the applications of graphs in programming?
Graphs have wide-ranging applications, including social network analysis, recommendation systems, route optimization, and more. They help represent complex relationships between data points.
Is Python the only programming language with data structures?
No, data structures are fundamental concepts in computer science and are available in various programming languages. However, Python’s simplicity and versatility make it a popular choice for implementing and using data structures.
Conclusion
Understanding data structures in Python is essential for effective programming and problem-solving. By leveraging the power of various data structures, you can optimize your code for better performance, readability, and efficiency. Whether you’re dealing with lists, dictionaries, trees, or graphs, Python offers a wide array of tools to manage and manipulate data effectively.
Remember, the choice of data structure depends on the specific task at hand. By mastering these concepts, you’ll be better equipped to tackle complex programming challenges and create elegant, efficient solutions.
SOURCEBAE: HIRE REACT DEVELOPER