How do I Practice Java for Selenium? Mastering the Art of Selenium Automation Testing
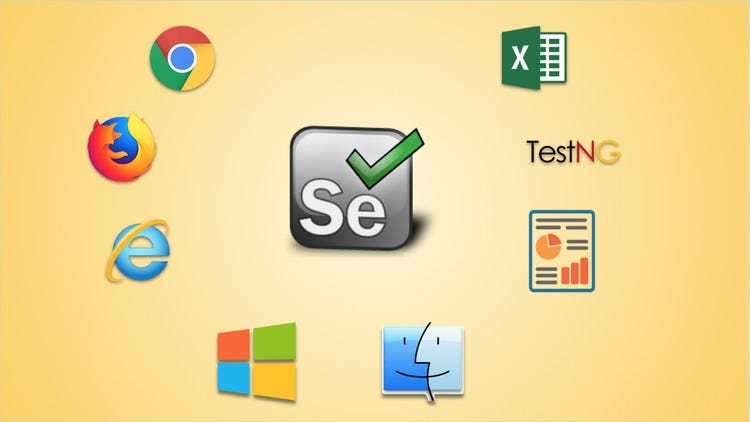
How do I Practice Java for Selenium?
Are you looking to enhance your skills in Selenium automation testing, specifically in the realm of Java? If so, you’ve come to the right place. In this comprehensive guide, we’ll take you through the ins and outs of practicing Java for Selenium, helping you become a proficient Selenium automation tester. Whether you’re a novice or an experienced tester looking to brush up on your skills, this guide has something for everyone.
Getting Started with Java for Selenium
Understanding the Basics
To embark on your journey to master Selenium automation testing with Java, it’s essential to have a solid grasp of the fundamentals. Java is a versatile and widely used programming language that’s perfectly suited for Selenium automation. Here are the key concepts to start with:
- Variables and Data Types: Get comfortable with declaring variables and using different data types in Java.
- Conditional Statements: Learn how to create if-else conditions to make decisions in your Selenium scripts.
- Loops: Master loops like for, while, and do-while, which are crucial for repetitive tasks in automation testing.
- Functions and Methods: Understand how to create and call functions, as well as work with predefined methods.
- Exception Handling: Learn to handle exceptions gracefully to prevent script crashes.
Setting Up Your Environment
Before you dive into practicing Java for Selenium, you need to set up your development environment. Here’s a brief overview of the necessary steps:
- Install Java Development Kit (JDK): Download and install the latest JDK version compatible with Selenium.
- Install Integrated Development Environment (IDE): Choose an IDE like Eclipse or IntelliJ IDEA to write and run your Java code efficiently.
- Selenium WebDriver: Download the Selenium WebDriver and set it up in your project.
- Browser Drivers: Depending on your target browser (Chrome, Firefox, etc.), download and configure the respective driver.
Writing Your First Selenium Script in Java
Now that your environment is ready, let’s write your first Selenium script in Java. This script will open a web page and interact with elements on it. Here’s a simple example:
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
public class FirstSeleniumTest {
public static void main(String[] args) {
// Set the path to your ChromeDriver executable
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver.exe");
// Initialize the WebDriver
WebDriver driver = new ChromeDriver();
// Open a website
driver.get("https://www.example.com");
// Find an element by its ID
WebElement element = driver.findElement(By.id("exampleElement"));
// Perform actions on the element
element.click();
// Close the browser
driver.quit();
}
}
This script opens the “example.com” website, locates an element by its ID, and clicks on it. This is just a starting point; Selenium offers a plethora of functions to interact with web elements and automate various tasks.
Best Practices for Practicing Java with Selenium
Code Reusability
One of the essential practices in Selenium automation testing is code reusability. Create functions and methods for common actions or interactions with web elements. This ensures cleaner, maintainable code.
Handling Dynamic Elements
Websites often have dynamic elements with changing IDs or attributes. To handle such scenarios, use Xpath or CSS selectors intelligently to locate elements.
Synchronization
Selenium executes scripts faster than web pages load. Implement explicit waits to ensure your script waits for elements to become available before interacting with them.
Test Frameworks
Consider using test frameworks like TestNG or JUnit to structure your Selenium tests, manage test data, and generate test reports.
FAQs
Is Java the best programming language for Selenium?
Java is one of the most popular programming languages for Selenium due to its compatibility and extensive community support. However, you can use other languages like Python and C# as well.
How do I handle pop-up windows in Selenium using Java?
You can use the getWindowHandles()
method to switch between pop-up windows in Selenium with Java. Ensure you identify the window using appropriate criteria.
What is the difference between Implicit and Explicit waits in Selenium?
Implicit waits wait for a specified amount of time for an element to be available, while explicit waits wait for a specific condition to be met before proceeding.
Can I run Selenium tests in parallel with Java?
Yes, you can run Selenium tests in parallel using Java. TestNG provides built-in support for parallel test execution.
Are there any good online resources to learn Java for Selenium?
Yes, there are plenty of online courses and tutorials that can help you learn Java for Selenium. Websites like Udemy, Coursera, and the official Selenium website are excellent starting points.
What is the future of Selenium automation testing?
Selenium continues to evolve and remains a crucial tool for web automation testing. Its future looks promising, with ongoing development and support from the Selenium community.
Conclusion
Practicing Java for Selenium is an invaluable skill for anyone in the field of automation testing. With the right foundation in Java and Selenium, you can create powerful automation scripts to test web applications efficiently. Remember to stay updated with the latest Selenium updates and best practices, and keep honing your skills.
So, what are you waiting for? Start your journey to becoming a Selenium automation testing expert today!
READ MORE: Why is HTML Important for Websites?