How Dynamic Routing Works in Express.js?
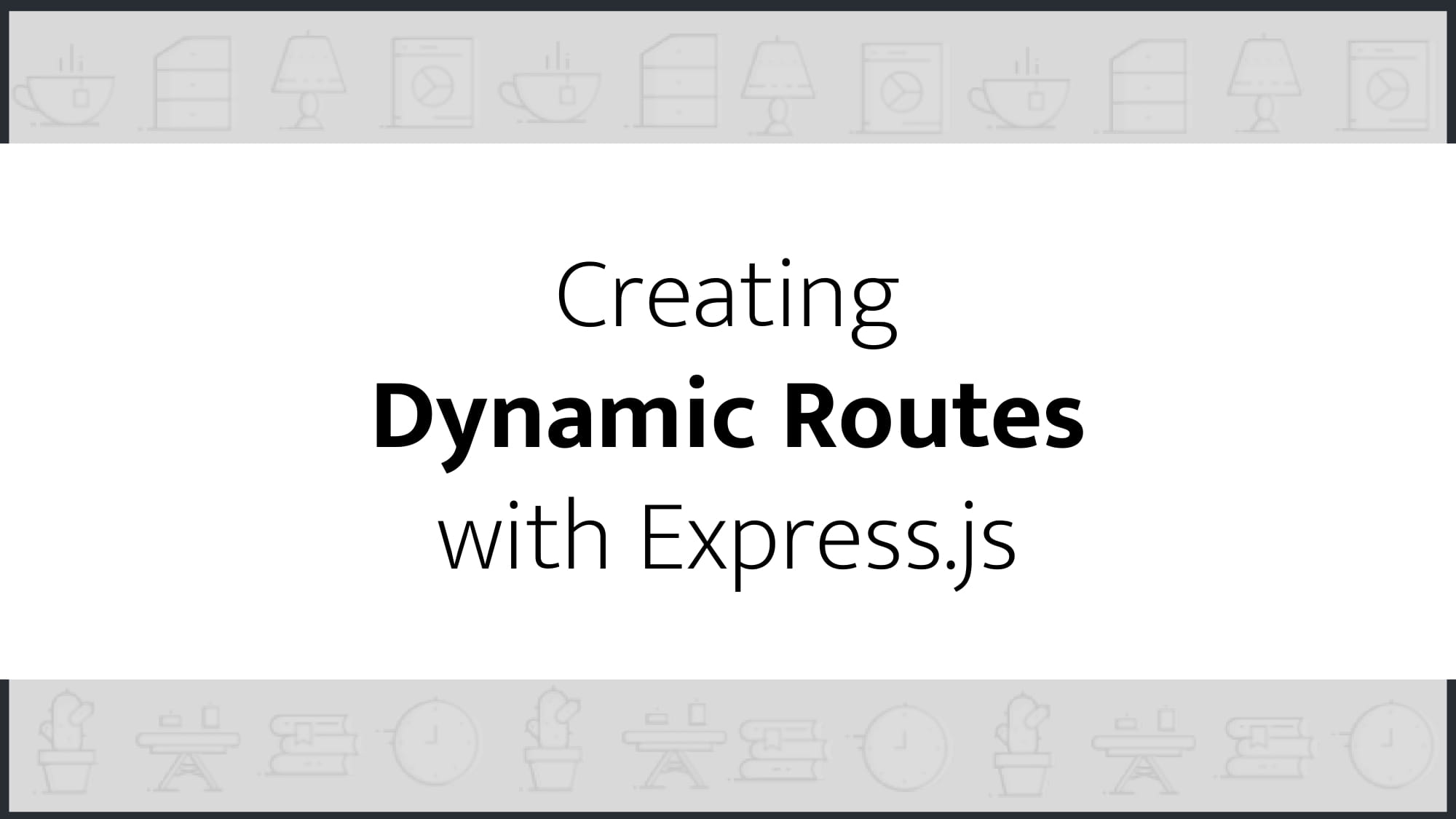
How Dynamic Routing Works in Express.js?
Dynamic routing in Express.js allows for creating flexible and customizable routes by incorporating parameters into the route paths. These parameters are placeholders in the route, and their values can vary, enabling the server to handle requests based on different input values.
Here’s a step-by-step explanation of how dynamic routing works in Express.js:
- Define a Dynamic Route with Parameters: When defining a route in Express.js, you can include parameters by specifying a colon followed by the parameter name in the route path. For example:
app.get('/users/:userId', (req, res) => {
const userId = req.params.userId;
res.send(`User ID: ${userId}`);
});
- Accessing Parameters in the Route Handler: Inside the route handler function, you can access the parameter values using
req.params
. In the example above,req.params.userId
would contain the value of theuserId
parameter from the URL. - Requesting the Dynamic Route: To request the dynamic route, you would make a GET request to a URL that matches the defined route with the specified parameter. For example, to access the user with ID 123:
GET /users/123
- Handling the Request: When a request is made to the dynamic route, Express.js matches the URL to the defined route pattern and extracts the parameter value. In this case, it would extract the
userId
from the URL. - Using the Parameter Value: The parameter value extracted from the URL can then be used within the route handler to customize the server’s response based on the parameter value.
app.get('/users/:userId', (req, res) => {
const userId = req.params.userId;
res.send(`User ID: ${userId}`);
});
In this example, the server responds with “User ID: 123” for the request to “/users/123”.
Dynamic routing in Express.js is powerful for building APIs and web applications where different routes are needed to handle various operations and functionalities based on user input or other contextual information.
Dynamic Route Parameters
Dynamic routing allows for the creation of route parameters by placing a colon (:
) before a parameter name in the route path. These parameters act as variables and can hold different values based on the client’s request.
app.get('/users/:userId', (req, res) => {
const userId = req.params.userId;
res.send(`User ID: ${userId}`);
});
In this example, userId
is a route parameter. When a client sends a GET request to /users/123
, the req.params.userId
will be '123'
, and the server will respond with “User ID: 123”.
Multiple Dynamic Parameters
You can define routes with multiple dynamic parameters to capture more specific information.
app.get('/products/:category/:productID', (req, res) => {
const { category, productID } = req.params;
res.send(`Category: ${category}, Product ID: ${productID}`);
});
Here, the route can handle URLs like /products/electronics/456
, and req.params
will contain { category: 'electronics', productID: '456' }
.
Optional Route Parameters
You can make route parameters optional by using a question mark (?
) after the parameter name.
app.get('/posts/:year/:month?', (req, res) => {
const { year, month } = req.params;
if (month) {
res.send(`Year: ${year}, Month: ${month}`);
} else {
res.send(`Year: ${year}`);
}
});
In this example, the month
parameter is optional. Both /posts/2023
and /posts/2023/09
are valid URLs, and the server will handle them accordingly.
Regular Expressions in Route Paths
You can use regular expressions in route paths for more complex matching patterns.
app.get(/^\/users\/(\d+)$/, (req, res) => {
const userId = req.params[0]; // Access using array index
res.send(`User ID: ${userId}`);
});
This route pattern matches URLs like /users/123
where 123
is a numeric user ID. The ID is extracted using a regular expression capturing group and accessed via req.params[0]
.
Error Handling for Dynamic Routes
It’s essential to handle errors for dynamic routes, especially when expecting certain parameters.
app.get('/users/:userId', (req, res) => {
const userId = req.params.userId;
if (!userId) {
res.status(400).send('User ID is required.');
} else {
res.send(`User ID: ${userId}`);
}
});
In this case, if a request is made without a user ID, the server will respond with a 400 Bad Request status and an error message.
Dynamic routing is a fundamental feature of Express.js, allowing developers to build flexible and scalable applications by leveraging the power of route parameters to handle a wide range of requests.
Frequently Asked Questions (FAQs)
What are dynamic routes in Express.js?
Dynamic routes in Express.js involve using route parameters to create flexible route patterns. Route parameters are placeholders in the route path that allow the server to handle a variety of requests based on different input values.
How do I define a dynamic route in Express.js?
To define a dynamic route, you include a parameter placeholder (e.g., :userId
) within the route path. This parameter can hold varying values based on the client’s request.
How do I access route parameters in Express.js?
You can access route parameters using req.params
within the route handler. The parameter values are extracted from the URL and can be used to customize the server’s response.
Can I have multiple dynamic parameters in a route in Express.js?
Yes, you can define routes with multiple dynamic parameters by placing placeholders for each parameter in the route path (e.g., /category/:categoryId/product/:productId
).
Are route parameters in Express.js optional?
Yes, you can make route parameters optional by placing a question mark (?
) after the parameter name in the route path (e.g., /posts/:year/:month?
).
Conclusion
Dynamic routing is a crucial feature in Express.js that allows developers to create flexible and adaptable APIs and web applications. By utilizing route parameters, you can design routes that respond to various client requests based on specific input values. These parameters provide a powerful mechanism for building dynamic and customizable applications that can handle a wide range of use cases. Understanding how to define, access, and handle dynamic routes is essential for effectively developing Express.js applications and providing a seamless user experience.