How to Build Microservices With Node.JS
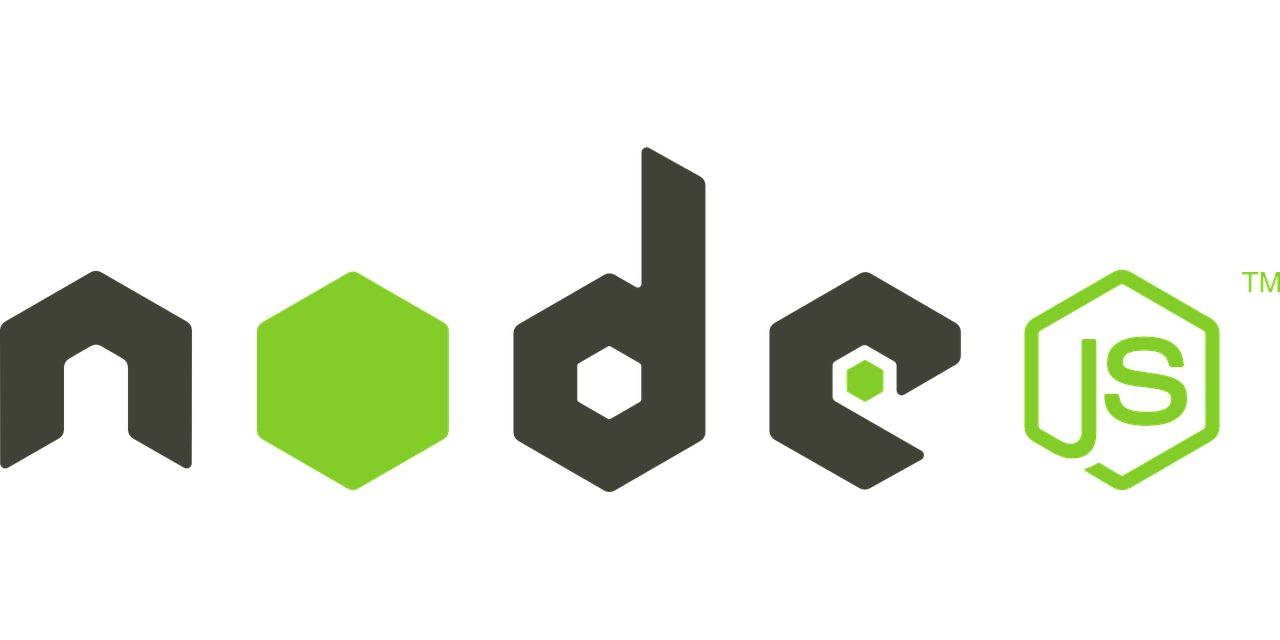
Microservices architecture has become a popular approach in designing and developing modern applications. It offers various benefits, such as scalability, flexibility, and easier maintenance.
In this article, we will explore how to build microservices using Node.js, a powerful JavaScript runtime.
1. Introduction to Microservices
Microservices are a software architectural style that structures an application as a collection of small, independent services that communicate with each other over a network. Each microservice is focused on a specific business capability and can be developed, deployed, and scaled independently. This allows for a highly modular and flexible architecture.
2. Introduction to Node.js
Node.js is a JavaScript runtime built on Chrome’s V8 JavaScript engine. It allows developers to build scalable, high-performance applications using JavaScript on both the client and server sides. Node.js is well-suited for building microservices due to its lightweight nature, event-driven architecture, and non-blocking I/O model.
3. Setting up a Node.js environment
Before we start building microservices with Node.js, we need to set up a Node.js environment. Firstly, we need to install Node.js on our machine. Visit the official Node.js website, download the installer for your operating system, and follow the installation instructions.
To work with Node.js, it’s also important to have a basic understanding of JavaScript, as it is the primary language used with Node.js.
4. Building a simple microservice with Node.js
To get started with building microservices, let’s build a simple microservice using Node.js. We’ll create a project structure, implement API endpoints, and interact with a database.
Firstly, we need to set up the project structure. Create a new directory for your project and initialize a new Node.js project by running the command npm init
. This will create a package.json
file which will contain information about your project.
Next, we can start implementing API endpoints using a web framework like Express.js. Express.js provides a simple and intuitive way to handle HTTP requests and build RESTful APIs. We can define routes, handle requests, and send responses using Express.js.
To interact with a database, we can use an ORM (Object-Relational Mapping) library like Sequelize. Sequelize provides an easy-to-use API for interacting with various databases such as MySQL, PostgreSQL, and SQLite. We can define models, perform CRUD operations, and handle database queries using Sequelize.
5. Implementing communication between microservices
In a microservices architecture, microservices often need to communicate with each other. There are two common approaches for implementing communication between microservices: using RESTful APIs and using message queues.
RESTful APIs provide a simple and standard way for microservices to communicate over HTTP. Each microservice exposes a set of API endpoints that other microservices can consume. This approach is suitable for synchronous communication.
Message queues, on the other hand, allow for asynchronous communication between microservices. Microservices can publish messages to a queue, and other microservices can consume those messages asynchronously. This approach is suitable for scenarios where real-time communication is not required or when services need to be decoupled.
6. Scaling microservices with Node.js
One of the advantages of using microservices architecture is the ability to scale individual services independently. In Node.js, we can scale microservices horizontally by running multiple instances of a service and distributing the traffic among them.
To achieve horizontal scaling, we can use a load balancer such as Nginx or HAProxy. The load balancer distributes incoming requests among the available service instances, ensuring that the workload is evenly distributed and the service remains responsive.
7. Managing data in microservices
Managing data in a microservices architecture can be challenging due to the distributed nature of the system. When building microservices with Node.js, it’s important to choose a suitable database technology that can handle the requirements of your application.
There are various database options available for Node.js, such as relational databases (e.g., MySQL, PostgreSQL), NoSQL databases (e.g., MongoDB, Redis), and graph databases (e.g., Neo4j). Each database technology has its own strengths and weaknesses, so it’s important to choose the one that fits your application’s needs.
Additionally, when using multiple databases in a microservices architecture, data synchronization becomes an important consideration. Different services may have their own databases, and it’s necessary to keep the data in sync between them to ensure consistency.
8. Securing microservices with Node.js
Security is a crucial aspect when building microservices. In a distributed system, it’s important to implement authentication and authorization mechanisms to ensure that only authorized users and services can access the microservices.
In Node.js, we can implement authentication and authorization using libraries like Passport.js and JSON Web Tokens (JWT). Passport.js provides a flexible authentication middleware and supports various authentication strategies, such as username/password, social login, and OAuth. JWT can be used to securely transmit user information between services.
In addition to authentication and authorization, it’s also important to implement other security measures such as input validation, rate limiting, and protecting against common web vulnerabilities like cross-site scripting (XSS) and SQL injection.
9. Testing and deploying microservices
Testing is an essential part of the development lifecycle. In Node.js, we can write unit tests using a testing framework like Mocha or Jest. These frameworks provide a rich set of features for writing and running tests, including assertions, test runners, and mocking utilities. By writing comprehensive unit tests, we can ensure that our microservices behave as expected and catch any potential bugs or regressions.
Deploying microservices built with Node.js can be done using various deployment strategies. We can deploy our microservices to a cloud platform like AWS, Azure, or Google Cloud Platform. These platforms provide infrastructure-as-a-service (IaaS) and platform-as-a-service (PaaS) solutions that simplify the deployment and management of microservices.
Alternatively, we can use containerization technologies like Docker and container orchestration platforms like Kubernetes to deploy and manage our microservices. Containerization allows us to package our microservices into lightweight, portable containers that can run consistently across different environments.
10. Best practices and considerations for building microservices with Node.js
When building microservices with Node.js, there are several best practices and considerations to keep in mind:
- Code organization and module separation: Properly organizing your codebase and separating modules will make it easier to understand and maintain your microservices.
- Monitoring and logging: Implementing monitoring and logging mechanisms will help you identify and troubleshoot issues in your microservices.
- Error handling and resilience: Your microservices should handle errors gracefully and have mechanisms in place to handle failures and recover from them.
11. Conclusion
In this article, we explored the process of building microservices with Node.js. We discussed the benefits of using microservices architecture, the suitability of Node.js for building microservices, and various aspects of building microservices, including communication, scaling, data management, security, testing, and deploying.
By following best practices and leveraging the features of Node.js, you can build robust and scalable microservices that are well-suited for modern application development.
FAQs
1. Can microservices be built using other programming languages?
Yes, microservices can be built using various programming languages. Node.js is just one of the many options available. Other popular choices include Python, Java, and Go.
2. Is Node.js the best choice for building microservices?
Node.js is a popular choice for building microservices due to its lightweight nature and non-blocking I/O model. However, the choice of programming language depends on various factors, such as the requirements of your application, familiarity with the language, and the ecosystem of libraries and frameworks available.
3. How do microservices improve scalability?
Microservices architecture allows for individual services to be scaled independently based on demand. This granular scaling ensures that only the necessary services are scaled, resulting in better resource utilization and improved overall scalability.
4. Can microservices communicate with each other in real-time?
Yes, microservices can communicate with each other in real-time using technologies such as WebSockets or message queues. Real-time communication is useful for scenarios where services need to push notifications or update data in real-time.
5. Can microservices be deployed on-premises?
Yes, microservices can be deployed on-premises, in addition to being deployed on cloud platforms. On-premises deployment allows organizations to have complete control over their infrastructure and data. However, it requires additional effort in terms of managing and maintaining the infrastructure.