How to Convert String to LocalDate in Java: A Comprehensive Guide
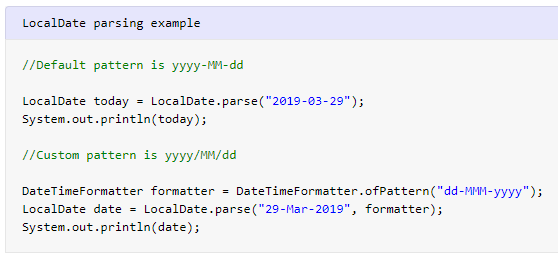
To convert a string to a LocalDate object, you’ll need to follow these steps:
- Parse the String: First, you need to parse the string that represents a date into a LocalDate object. Java provides the
LocalDate.parse()
method for this purpose.
String dateString = "2023-09-05";
LocalDate date = LocalDate.parse(dateString);
- Handling Different Date Formats: If your input string follows a specific date format other than the default ISO format (yyyy-MM-dd), you can use a
DateTimeFormatter
to specify the format explicitly.
String dateString = "05/09/2023";
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("dd/MM/yyyy");
LocalDate date = LocalDate.parse(dateString, formatter);
- Error Handling: Be mindful of potential exceptions when parsing. If the string does not conform to the specified format, a
DateTimeParseException
will be thrown. Ensure you handle this exception appropriately. - Utilize Try-Catch Blocks: To gracefully handle exceptions, enclose the parsing code in a try-catch block.
try {
LocalDate date = LocalDate.parse(dateString, formatter);
} catch (DateTimeParseException e) {
// Handle the exception
e.printStackTrace();
}
Common Challenges and Solutions
While converting strings to LocalDate is relatively straightforward, there are some common challenges you might encounter:
Handling Time Zone Differences
If your application needs to consider time zones when converting strings to LocalDate, you should use ZonedDateTime
or OffsetDateTime
to ensure proper handling of time zone offsets.
Dealing with Invalid Dates
When dealing with user input, it’s crucial to validate the input string to prevent invalid dates from being parsed. You can implement custom validation logic to ensure that the input adheres to your application’s requirements.
Leap Year Considerations
Remember that LocalDate automatically accounts for leap years, so you don’t need to worry about complex date calculations.
FAQs
Can I convert a string with a time component to LocalDate?
No, LocalDate represents a date without a time component. If your string includes a time component, consider using LocalDateTime
instead.
What should I do if the input string format varies?
You can use the DateTimeFormatter
class to specify the expected format when parsing the string. This allows you to handle different date formats gracefully.
Is LocalDate thread-safe?
Yes, LocalDate is thread-safe because it is immutable. Multiple threads can safely access and use LocalDate objects without synchronization concerns.
How can I handle date arithmetic with LocalDate?
LocalDate provides various methods for performing date arithmetic, such as adding or subtracting days, months, or years. These operations are straightforward and can be used for various date-related calculations.
Are there any performance considerations when using LocalDate?
LocalDate is designed to be efficient and is suitable for most date-related tasks. However, if you need to work with date and time together, consider using LocalDateTime
or ZonedDateTime
based on your requirements.
Can I format a LocalDate object as a string?
Yes, you can format a LocalDate object as a string using the DateTimeFormatter
class. This allows you to display the date in a specific format according to your needs.
Conclusion
In this comprehensive guide, we’ve explored how to convert a string to a LocalDate object in Java. LocalDate is a powerful class for managing date-related information in your Java applications. By following the steps outlined in this article, you can confidently handle string-to-LocalDate conversions and unlock the full potential of this versatile Java class.