How to Create Array in JavaScript?
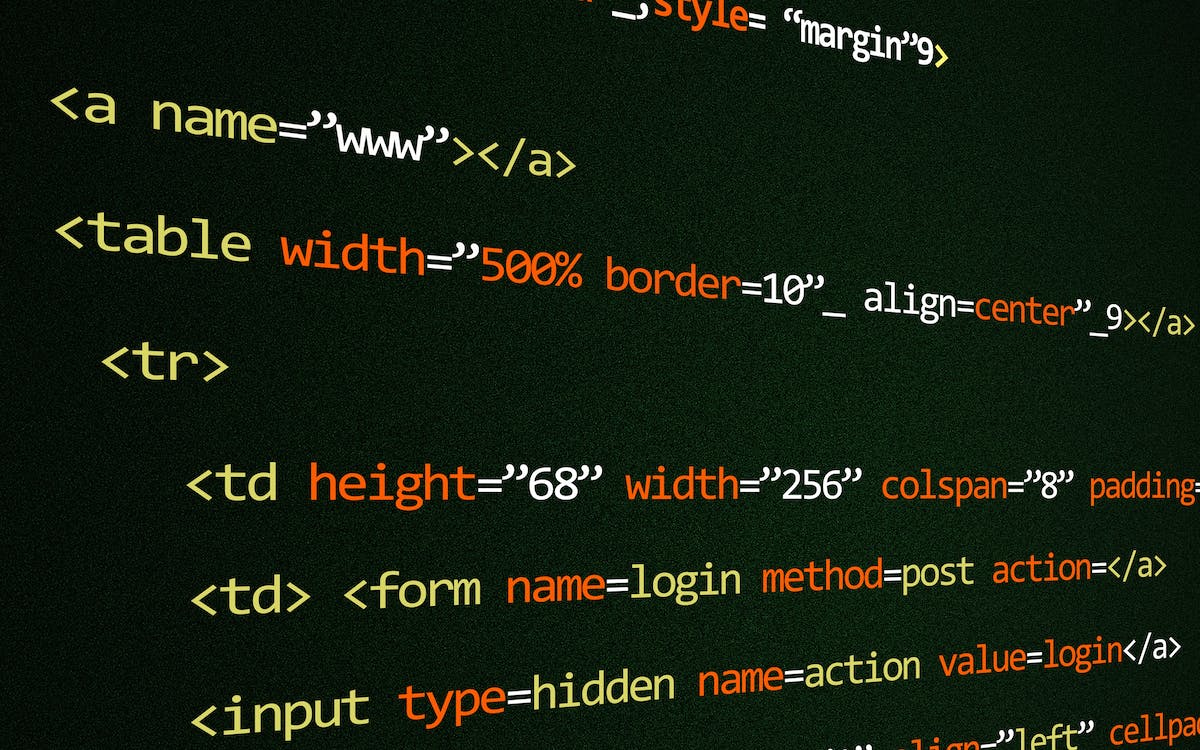
How to Create Array in JavaScript?
Arrays are fundamental data structures in JavaScript that allow you to store multiple values in a single variable. They are used extensively in programming to manage collections of data. This comprehensive guide will walk you through the process of creating arrays in JavaScript, from the basics to more advanced techniques. Whether you’re a beginner or looking to enhance your JavaScript skills, this article will provide you with the knowledge you need to efficiently create and work with arrays.
1. Getting Started with Arrays
Arrays are containers that hold multiple values. To create an array in JavaScript, use the following syntax:
let myArray = [];
You can also initialize an array with values:
let numbers = [1, 2, 3, 4, 5];
let fruits = ['apple', 'banana', 'orange'];
2. Array Indexing
Arrays are zero-indexed, meaning the first element is at index 0, the second at index 1, and so on. Access array elements using their index:
let colors = ['red', 'green', 'blue'];
let firstColor = colors[0]; // Returns 'red'
let secondColor = colors[1]; // Returns 'green'
3. Adding Elements to an Array
You can add elements to an array using the push()
method:
let animals = ['cat', 'dog'];
animals.push('elephant'); // Adds 'elephant' to the end
4. Removing Elements from an Array
To remove elements from an array, you can use the pop()
method to remove the last element:
let fruits = ['apple', 'banana', 'orange'];
fruits.pop(); // Removes 'orange'
5. Array Length
Retrieve the number of elements in an array using the length
property:
let numbers = [10, 20, 30, 40, 50];
let length = numbers.length; // Returns 5
6. Creating Multi-dimensional ArraysJavaScript arrays can hold other arrays, creating multi-dimensional arrays:
let matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]];
let firstRow = matrix[0]; // Returns [1, 2, 3]
let element = matrix[1][2]; // Returns 6
7. Modifying Array Elements
You can change the value of an array element by directly assigning a new value:
let fruits = ['apple', 'banana', 'orange'];
fruits[1] = 'grape'; // Changes 'banana' to 'grape'
8. Iterating Through Arrays
Loop through array elements using various iteration methods like for
loops and forEach()
:
let numbers = [1, 2, 3, 4, 5];
for (let i = 0; i < numbers.length; i++) {
console.log(numbers[i]);
}
9. Array Methods for Manipulation
JavaScript provides several built-in methods for array manipulation, including splice()
, concat()
, and slice()
.
- The
splice()
method removes, replaces, or adds elements to an array. - The
concat()
method combines arrays. - The
slice()
method creates a new array by extracting a portion of an existing array.
10. Searching and Sorting Arrays
JavaScript arrays offer methods to search for elements and sort the array:
- The
indexOf()
method returns the index of the first occurrence of a specified element. - The
includes()
method checks if an element exists in the array. - The
sort()
method sorts array elements alphabetically or numerically.
11. Array Destructuring
Array destructuring allows you to extract array elements into individual variables:
let colors = ['red', 'green', 'blue'];
let [firstColor, secondColor] = colors;
12. Spread Operator for Arrays
The spread operator allows you to expand an array into individual elements:
let numbers = [1, 2, 3];
let newNumbers = [...numbers, 4, 5];
13. Creating Arrays with Array.from()
The Array.from()
method creates a new array from an iterable object:
let range = Array.from({ length: 5 }, (_, index) => index + 1);
14. Creating Arrays with Array.of()
The Array.of()
method creates a new array with the provided elements:
let values = Array.of(1, 2, 3, 4, 5);
15. Filtering Arrays
Use the filter()
method to create a new array with elements that pass a given condition:
let numbers = [1, 2, 3, 4, 5];
let evenNumbers = numbers.filter(number => number % 2 === 0);
16. Mapping Arrays
The map()
method transforms each element of an array and creates a new array:
let numbers = [1, 2, 3, 4, 5];
let doubledNumbers = numbers.map(number => number * 2);
17. Reducing Arrays
The reduce()
method combines array elements into a single value:
let numbers = [1, 2, 3, 4, 5];
let sum = numbers.reduce((accumulator, current) => accumulator + current, 0);
18. Common Mistakes to Avoid
- Forgetting the parentheses when using array methods (e.g.,
push()
instead ofpush
). - Accidentally modifying the original array while performing operations.
FAQs
How do I create an empty array in JavaScript?
To create an empty array, use the following syntax:
let emptyArray = [];
Can I change the size of an array after creating it?
Yes, you can change the size of an array by adding or removing elements using methods like push()
and pop()
.
What is the difference between push()
and unshift()
?
The push()
method adds elements to the end of an array, while the unshift()
method adds elements to the beginning.
How can I check if an element exists in an array?
You can use the includes()
method to check if an element exists in an array:
let fruits = ['apple', 'banana', 'orange'];
let exists = fruits.includes('banana'); // Returns true
What is array destructuring used for?
Array destructuring allows you to extract elements from an array and assign them to variables in a concise way.
Can I sort an array of numbers in descending order?
Yes, you can sort an array of numbers in descending order using the sort()
method with a custom comparator function.
Conclusion
Creating arrays in JavaScript is a fundamental skill for any developer. By following the techniques and methods outlined in this article, you can efficiently create arrays, manipulate their contents, and make use of various array-related functionalities. Whether you’re working on a simple script or a complex web application, mastering arrays will enhance your ability to manage and process data effectively.
READ MORE | How to Become a React Developer?