How to Create REST API in Java: A Comprehensive Guide
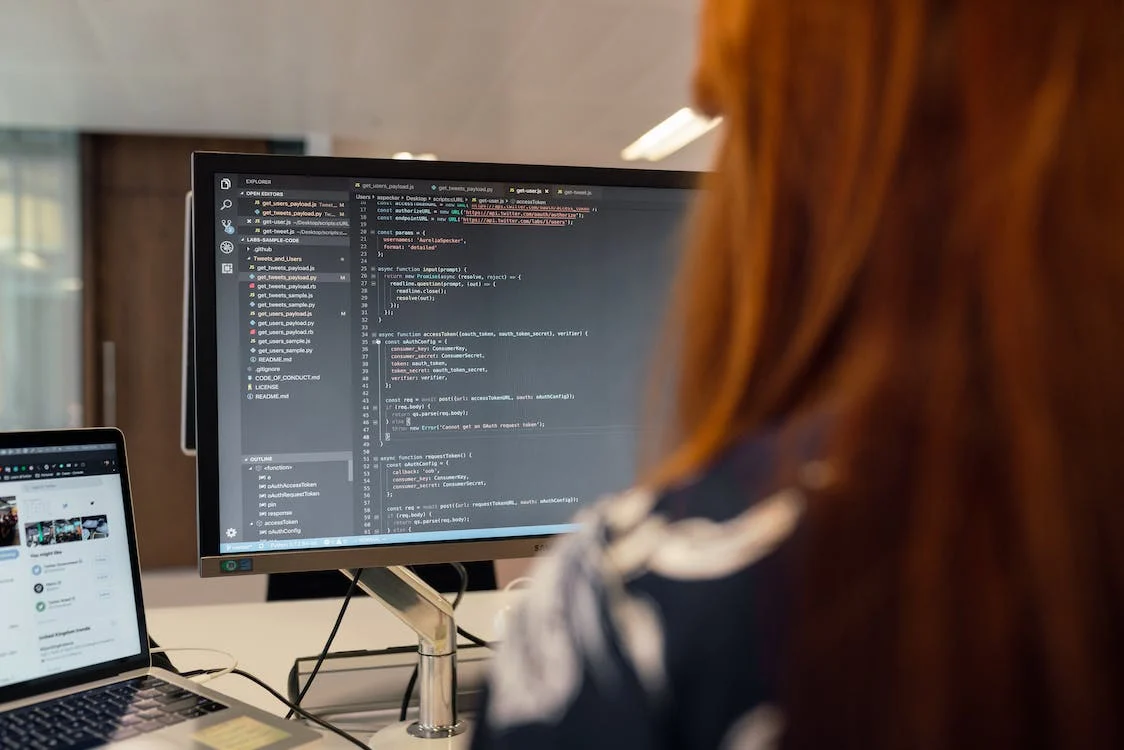
How to Create REST API in Java: A Comprehensive Guide
In today’s fast-paced digital world, creating efficient and reliable APIs is essential for seamless communication between applications. Representational State Transfer (REST) has become the standard architectural style for designing networked applications. This guide will walk you through the process of creating a REST API in Java, from the fundamental concepts to hands-on implementation.
1. Understanding REST Architecture
REST architecture serves as the foundation for creating RESTful APIs. It emphasizes the use of stateless interactions, making APIs scalable and easily maintainable.
2. Setting Up Your Development Environment
Before you dive into coding, you need to set up your development environment. Install Java Development Kit (JDK) and an Integrated Development Environment (IDE) like Eclipse or IntelliJ IDEA.
3. Choosing a Java Framework
Selecting the right Java framework can greatly simplify the API development process. Spring Boot, for instance, offers powerful tools for building REST APIs effortlessly.
4. Defining Your API’s Purpose
Clearly define the purpose of your API. What resources will it expose? What actions can be performed? Mapping out these details is crucial for a well-structured API.
5. Designing API Endpoints
Design your API endpoints following the principles of REST. Use meaningful and intuitive URLs that represent the resources and actions.
6. Handling HTTP Methods
HTTP methods such as GET, POST, PUT, and DELETE are essential for defining the operations that can be performed on your resources.
7. Request and Response Formats
Understand JSON and XML formats for structuring request and response data. Choose the format that best suits your API’s requirements.
8. Implementing CRUD Operations
CRUD (Create, Read, Update, Delete) operations are the core of most APIs. Learn how to implement these operations effectively.
9. Data Persistence
Explore various options for data storage and persistence, such as relational databases or NoSQL solutions.
10. Error Handling and Validation
Implement robust error handling mechanisms and input validation to ensure the security and reliability of your API.
11. Authentication and Authorization
Secure your API by implementing authentication and authorization mechanisms. Use tokens or API keys to control access.
12. Versioning Your API
As your API evolves, maintaining backward compatibility is crucial. Learn how to version your API effectively.
13. Testing Your API
Thoroughly test your API using tools like Postman to ensure all endpoints and functionalities work as expected.
14. Documentation is Key
Create comprehensive documentation for your API. Include details on endpoints, request/response formats, and usage examples.
15. Implementing Rate Limiting
Prevent abuse and ensure fair usage by implementing rate limiting on your API.
16. Implementing HATEOAS
Hypermedia as the Engine of Application State (HATEOAS) enhances the discoverability of your API by including links to related resources.
17. Handling Security Concerns
Address security vulnerabilities such as SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF).
18. Performance Optimization
Optimize your API for performance by caching responses, minimizing network requests, and using efficient algorithms.
19. Monitoring and Analytics
Implement monitoring tools and analytics to track the usage and performance of your API. This insight can guide improvements.
20. Scaling Your API
Learn strategies for scaling your API to handle increased traffic and ensure a seamless experience for users.
21. Continuous Integration and Deployment
Implement CI/CD pipelines to automate testing, deployment, and updates of your API.
22. Best Practices and Patterns
Explore best practices and design patterns for building maintainable and efficient REST APIs in Java.
23. Keeping Up with Industry Trends
Stay updated with the latest trends in API development, such as GraphQL integration and microservices architecture.
24. Troubleshooting and Debugging
Develop effective troubleshooting and debugging skills to identify and resolve issues in your API.
25. FAQs
Q: What is a REST API?
A: A REST API (Representational State Transfer Application Programming Interface) is a set of rules and conventions for building and interacting with web services using the HTTP protocol.
Q: Why should I use Java for building REST APIs?
A: Java offers a robust and mature ecosystem for building scalable and secure applications. It has a wide range of libraries and frameworks that simplify the process of creating REST APIs.
Q: Can I build a REST API without a framework?
A: While building a REST API without a framework is possible, using a framework like Spring Boot can significantly accelerate the development process and provide various tools and features out of the box.
Q: What is the role of JSON in REST API development?
A: JSON (JavaScript Object Notation) is a lightweight data interchange format. It is commonly used in REST API development for structuring request and response data due to its simplicity and readability.
Q: Is it necessary to version my API?
A: Versioning your API is essential to ensure backward compatibility as your API evolves. It allows you to make changes without disrupting existing consumers of your API.
Q: How can I ensure the security of my REST API?
A: Implement authentication and authorization mechanisms, handle input validation, and address security vulnerabilities to ensure the security of your REST API.
Conclusion
Creating a REST API in Java is an exciting journey that requires a blend of technical expertise and creative problem-solving. By following this comprehensive guide, you’ve gained a solid foundation for building robust and efficient RESTful APIs that cater to modern application needs. Remember, staying updated with industry trends and continuously improving your skills will set you on the path to becoming an API development expert.
READ MORE | How to Become a React Developer?