How to Sort an Array in Javascript?
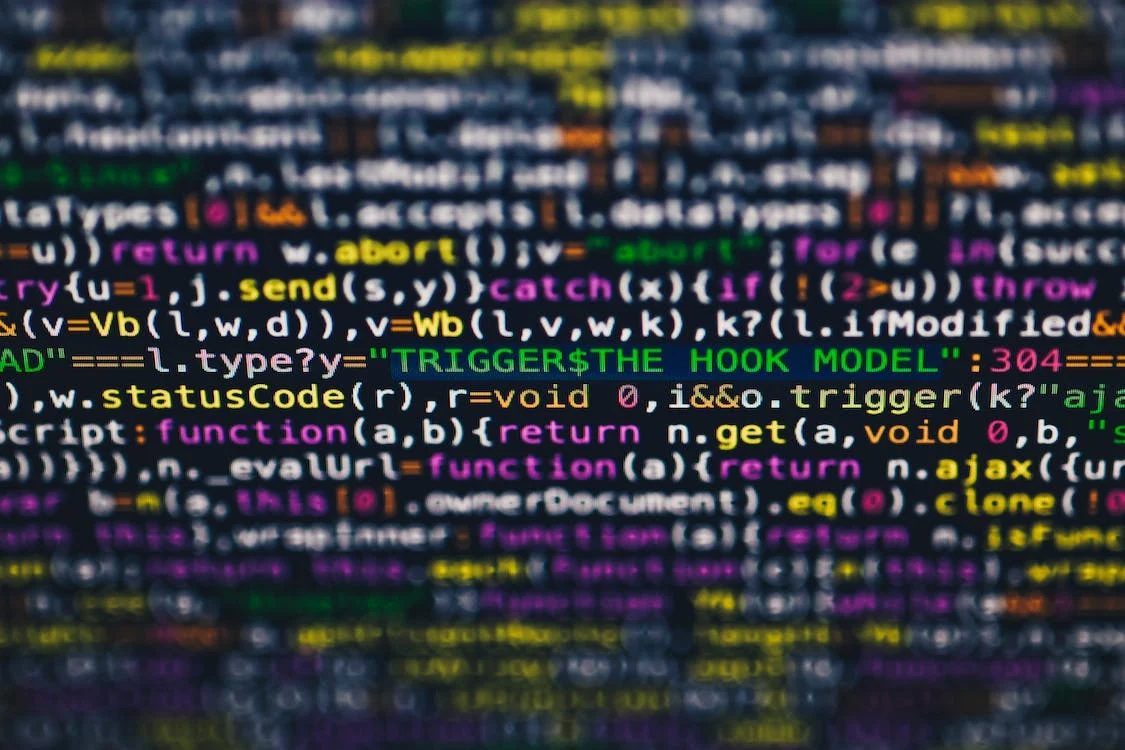
How to Sort an Array in Javascript?
Arrays are a fundamental data structure in JavaScript, allowing you to store and manage collections of elements. Sorting an array is a crucial skill for any JavaScript developer. Whether you’re arranging a list of numbers, strings, or more complex objects, mastering array sorting can greatly enhance your programming capabilities. In this guide, we’ll delve into the various techniques and methods to sort an array in JavaScript, providing you with valuable insights and practical examples.
Sorting an array in JavaScript involves arranging its elements in a specific order, which can be either ascending or descending. The default sorting order is lexicographic (alphabetical for strings and ascending for numbers). JavaScript offers several built-in methods to accomplish array sorting, each with its unique advantages and use cases.
Using the sort()
Method
The sort()
method is a versatile option for sorting arrays. It modifies the original array and arranges its elements based on their string representations. To sort an array of numbers, you can provide a comparison function that specifies the desired sorting order.
Leveraging the localeCompare()
Function
For sorting an array of strings, the localeCompare()
function is a powerful tool. It enables you to customize the sorting based on locale-specific rules, accommodating various languages and cultural contexts.
Sorting Numbers with the sort()
Method
To sort an array of numbers using the sort()
method, you need to provide a comparison function. This function takes two arguments and returns a value indicating their relative order. By manipulating the comparison logic, you can achieve both ascending and descending sorts.
Sorting Complex Objects
Sorting arrays of complex objects requires a bit more effort. You’ll need to provide a custom comparison function that specifies how to compare the objects’ properties. This allows you to sort objects based on different criteria, such as names or ages.
The reverse()
Method for Descending Order
While the default sorting order is ascending, you can easily reverse the order of a sorted array using the reverse()
method. This is particularly useful when you need a descending sort.
Array Sorting Techniques
Sorting algorithms play a crucial role in the efficiency of array sorting. JavaScript employs a stable sorting algorithm known as Timsort, which combines merge sort and insertion sort. Understanding the underlying techniques can help you optimize your code for different scenarios.
Timsort: A Glimpse into Efficiency
Timsort, the algorithm behind JavaScript’s built-in sort()
method, is designed for optimal performance on real-world data. It divides the array into small chunks, sorts them, and then merges them back together. This approach ensures efficiency while maintaining stability in sorting.
Quicksort: A Divide and Conquer Strategy
Quicksort is another popular sorting algorithm often used for its simplicity and efficiency. It works by selecting a “pivot” element and partitioning the array into two sub-arrays – elements less than the pivot and elements greater than the pivot. This process is repeated recursively, resulting in a sorted array.
Mergesort: Balancing Performance and Predictability
Mergesort is a stable, divide-and-conquer algorithm that works well for large datasets. It divides the array into smaller sub-arrays, sorts them, and then merges them to produce a fully sorted array. Its predictable time complexity makes it a reliable choice for various sorting needs.
Practical Examples
Let’s explore practical examples to solidify your understanding of array sorting in JavaScript.
Sorting Names Alphanumerically
Suppose you have an array of names and want to sort them alphanumerically. Using the sort()
method with a comparison function tailored for strings will yield the desired result.
Sorting Numeric Values
Sorting an array of numbers is a common task. By providing a custom comparison function to the sort()
method, you can achieve ascending or descending sorts based on your requirements.
Sorting Objects by Property
Imagine you have an array of objects, each representing a person with a name and age. To sort the array by age, you can implement a comparison function that accesses the “age” property of each object.
FAQs
How does the localeCompare()
function work in array sorting?
The localeCompare()
function compares strings based on their locale-specific order. It considers language-specific rules, ensuring accurate sorting for different languages and regions.
Can I sort an array of complex objects without a custom comparison function?
No, sorting complex objects requires a custom comparison function. This function defines how the objects should be compared and sorted based on specific properties.
Is Timsort suitable for sorting large datasets?
Yes, Timsort’s divide-and-conquer approach, coupled with its efficient merging strategy, makes it suitable for sorting large datasets while maintaining stability.
What’s the advantage of using the reverse()
method after sorting?
The reverse()
method provides a convenient way to switch the sorting order from ascending to descending without needing a separate comparison function.
Are there built-in functions to sort arrays in descending order?
JavaScript’s built-in sort()
method arranges elements in ascending order by default. To achieve descending order, you can sort the array in ascending order and then use the reverse()
method.
How can I choose the most appropriate sorting algorithm for my data?
The choice of sorting algorithm depends on factors such as the size of the data, its initial order, and any performance requirements. Consider the characteristics of different algorithms to make an informed decision.
Conclusion
Mastering the art of sorting arrays in JavaScript is essential for efficient data manipulation and organization. With the techniques and methods discussed in this guide, you’re well-equipped to tackle array sorting tasks of varying complexities. From sorting numbers to arranging objects based on custom properties, JavaScript offers a range of tools to streamline your development process. By understanding the algorithms and applying the appropriate techniques, you’ll be able to create more efficient and robust programs.
READ MORE | How to Become a React Developer?