How to Write Functions in JavaScript?
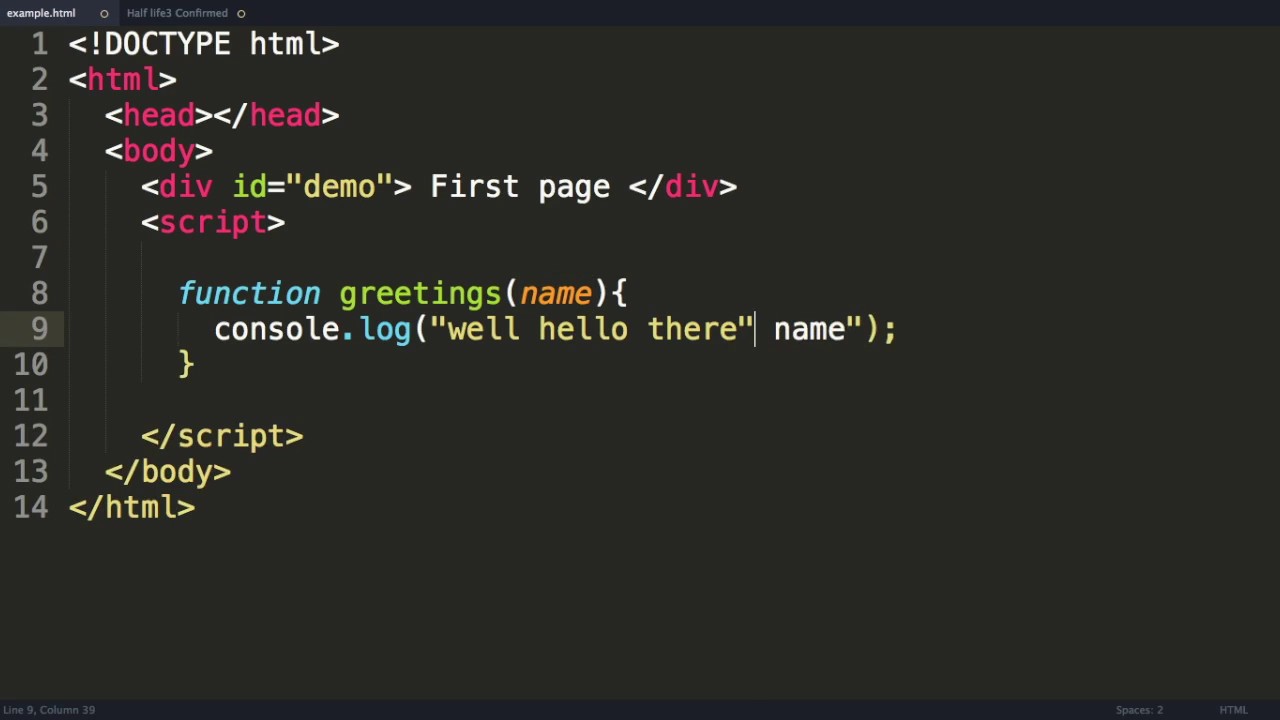
Functions can accept parameters, which are placeholders for values that the function needs to work with. When you invoke the function, you provide actual values called arguments. For example:
function greetUser(name) {
console.log(`Hello, ${name}!`);
}
greetUser("Alice"); // Outputs: Hello, Alice!
Advanced Techniques: Harnessing the Full Potential of JavaScript Functions
As you become more comfortable with the basics, it’s time to explore advanced techniques that will elevate your coding skills:
- Return Statements for Value
Functions can return values using the
return
statement. This allows you to use the result of a function call in other parts of your code:function multiply(a, b) {
return a * b;
}
const result = multiply(3, 4);
console.log(result); // Outputs: 12
- Anonymous Functions and Arrow Functions