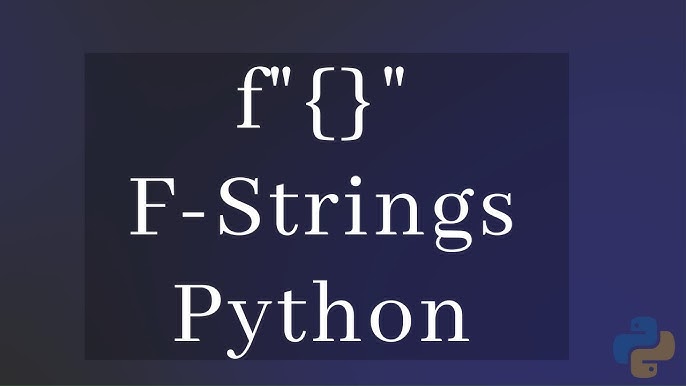
Mastering Python Strings: A Comprehensive Guide to Strings in Python
Python programming language is celebrated for its versatility and simplicity. Among its various data types, strings stand as a cornerstone, enabling developers to work with textual data efficiently. In this guide, we’ll delve into the world of Python strings, unraveling their nuances, and equipping you with the skills to wield them effectively.
Strings, in the context of programming, are sequences of characters enclosed within single (‘ ‘) or double (” “) quotes. These character sequences can encompass letters, numbers, symbols, and even whitespace. Python’s flexible approach to strings allows developers to manipulate and transform textual data seamlessly.
What Are Strings and Why Are They Important?
Strings are the building blocks of text-based data manipulation in Python. They enable us to process and present textual information, making them indispensable in various applications such as data processing, user input validation, and output formatting.
The Power of Python Strings
Python strings offer a plethora of capabilities, ranging from simple concatenation to complex manipulation. These operations empower developers to create dynamic text outputs, perform data analysis, and implement user-friendly interfaces.
Exploring Basic String Operations
Understanding fundamental string operations is pivotal for any Python programmer. Let’s explore some key techniques to harness the potential of strings.
Concatenation: Combining Strings
Concatenation involves merging multiple strings to create a single unified string. In Python, this is achieved using the +
operator. For example:
first_name = "John"
last_name = "Doe"
full_name = first_name + " " + last_name
String Formatting: Adding Variables to Strings
String formatting enables the inclusion of variables within strings for dynamic content. Python provides various methods for string formatting, with the f-string being a modern and concise approach:
name = "Alice"
age = 30
intro = f"My name is {name} and I'm {age} years old."
Length of a String: Counting Characters
To determine the length of a string, the len()
function comes in handy. It returns the total number of characters within a string, including letters, numbers, and symbols:
text = "Hello, world!"
length = len(text) # This will be 13
Essential String Methods
Python equips developers with a range of built-in string methods that simplify string manipulation. Let’s delve into some crucial methods.
Changing Case: upper()
and lower()
The upper()
method converts all characters in a string to uppercase, while lower()
converts them to lowercase. These methods are useful for consistent formatting:
text = "Python is Amazing"
uppercase_text = text.upper()
lowercase_text = text.lower()
Removing Whitespace: strip()
The strip()
method removes leading and trailing whitespace from a string, ensuring clean data input:
input_string = " User123 "
cleaned_string = input_string.strip()
Splitting Strings: split()
The split()
method divides a string into a list of substrings based on a specified delimiter. This is invaluable for parsing and processing textual data:
sentence = "Python programming is fun"
word_list = sentence.split(" ") # Returns ['Python', 'programming', 'is', 'fun']
Frequently Asked Questions (FAQs)
How do I check if a specific word is present in a string?
To check if a certain word is present in a string, you can use the in
keyword:
text = "Python is versatile"
if "versatile" in text:
print("The word 'versatile' is present.")
Can I concatenate strings of different data types?
Yes, Python allows concatenating strings with other data types using the str()
function to convert non-string data types into strings:
age = 25
message = "I am " + str(age) + " years old."
What’s the difference between single and double quotes for strings?
In Python, both single and double quotes can be used to define strings. Using one type of quote inside the other allows you to include quotes within your string without causing syntax errors:
single_quote_string = 'He said, "Hello!"'
double_quote_string = "She replied, 'Hi there!'"
How can I replace a specific substring in a string?
You can use the replace()
method to replace a specific substring with another substring:
text = "I like apples"
new_text = text.replace("apples", "bananas")
Is string manipulation in Python Unicode-aware?
Yes, Python strings are Unicode-aware, which means they can handle a wide range of characters from different languages and scripts without issues.
Can I compare strings in a case-insensitive manner?
Yes, you can achieve case-insensitive string comparison by converting both strings to either lowercase or uppercase using the lower()
or upper()
methods:
string1 = "Hello"
string2 = "hello"
if string1.lower() == string2.lower():
print("The strings are equal, ignoring case.")
Conclusion
Mastering string manipulation is pivotal for any Python developer. From basic concatenation to advanced formatting, Python strings offer a versatile toolkit to handle textual data effectively. By understanding the fundamental string operations and exploring the rich array of string methods, you’re well-equipped to tackle a wide range of programming tasks with confidence. So, dive into the world of Python strings and unleash their potential in your code!
SOURCEBAE: HIRE REACT DEVELOPER