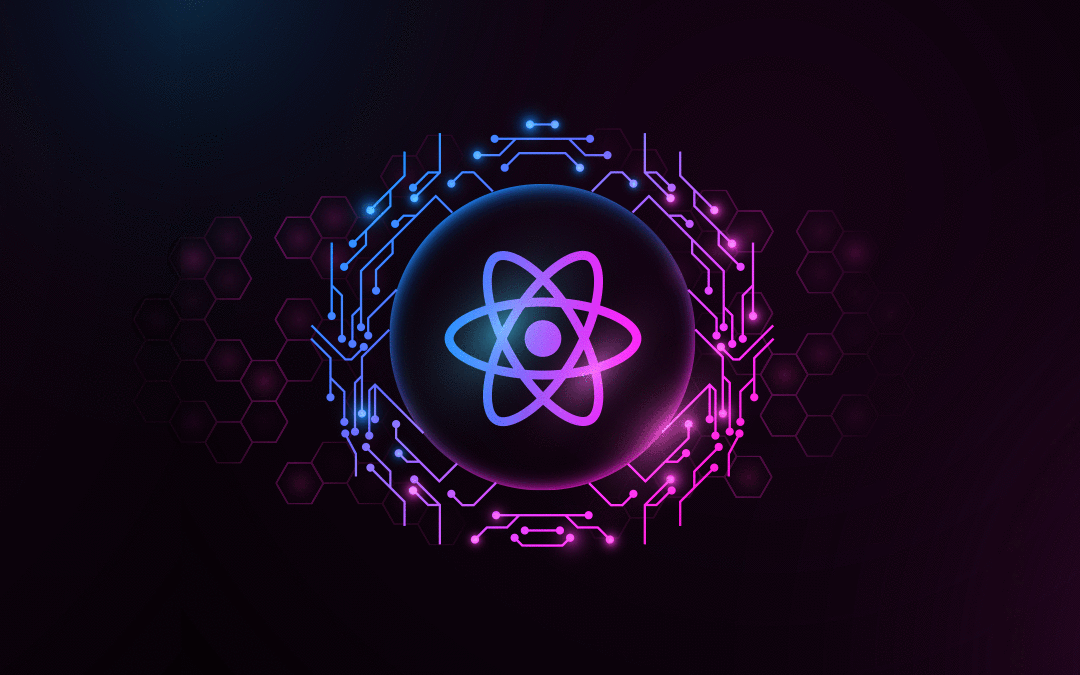
For instance, let’s integrate a Bootstrap modal in a React component:
1. Import the necessary components at the top of your React file:
import React, { useState } from ‘react’; import ‘bootstrap/dist/css/bootstrap.min.css’; import { Button, Modal } from ‘react-bootstrap’;
2. Inside your functional component, set up a state to control the modal's visibility:
const [showModal, setShowModal] = useState(false);
3. Add a button that triggers the modal:
<Button variant=”primary” onClick={() => setShowModal(true)}> Open Modal </Button>
4. Create the modal element using the 'Modal' component from Bootstrap and conditionally render it based on the 'showModal' state.
7. Enhancing Performance:
While integrating Bootstrap can greatly enhance your UI, it’s crucial to consider performance. Bootstrap’s complete library might contain features that you don’t need, leading to unnecessary bloat in your application. To optimize performance, consider using tools like ‘react-bootstrap’ that allow you to selectively import only the components you need.
FAQs:
1. Can I use a custom Bootstrap theme with React?
Absolutely! You can create a custom Bootstrap theme using SCSS variables and integrate it into your React application, giving it a unique and personalized appearance.
2. Is it possible to customize the behavior of Bootstrap components?
Yes, Bootstrap components often come with customizable options that you can modify to match your application’s requirements. This can be achieved through props or additional JavaScript configurations.
3. Can I integrate third-party libraries with Bootstrap components in React?
Certainly! Bootstrap components are React-friendly and can work seamlessly with other React libraries to enhance your application’s functionality.
4. Do I need to include all of Bootstrap’s CSS and JavaScript in my project?
No, you can optimize your project’s performance by selectively importing only the Bootstrap components and features that you need.
5. Is Bootstrap responsive by default when used with React?
Yes, Bootstrap’s responsive grid system ensures that your application’s layout adapts gracefully to different screen sizes and devices.
6. Are there alternatives to Bootstrap for styling React applications?
Yes, there are other UI frameworks like Material-UI and Semantic UI that offer unique styles and components for React applications.