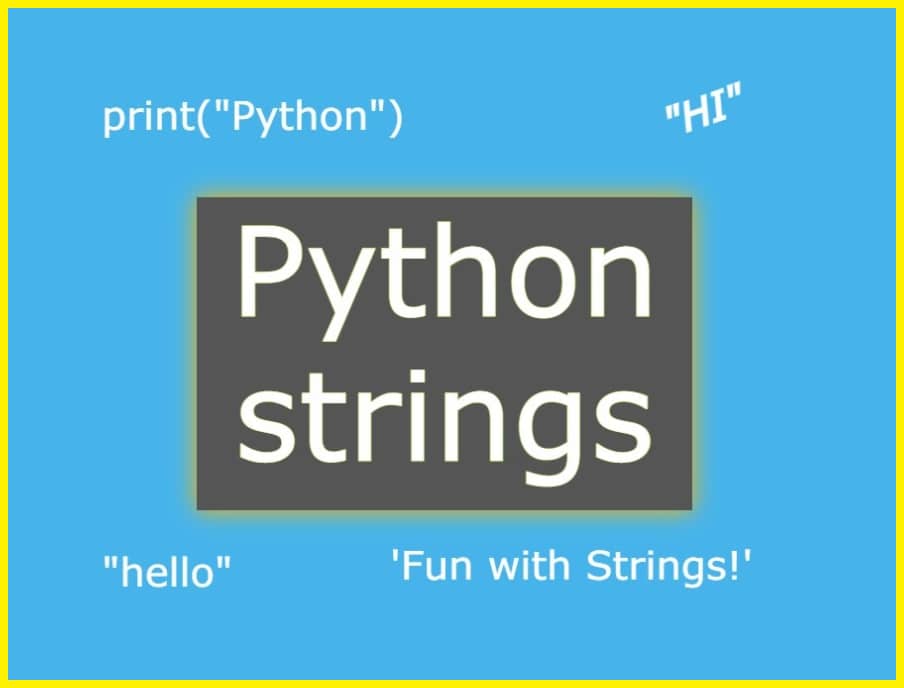
Python Strings: A Detailed Tutorial
In the realm of programming, strings are the unsung heroes that play a vital role in storing and manipulating textual data. Python, a versatile and powerful programming language, offers a plethora of tools and techniques to handle strings with finesse. Whether you’re a seasoned developer or just starting your coding journey, this comprehensive guide will take you through everything you need to know about Python strings.
Python Strings: What Are They?
At its core, a string in Python is a sequence of characters enclosed within single, double, or triple quotes. These characters can be letters, numbers, symbols, or even spaces. Strings serve as the building blocks for representing textual data in Python programs.
Creating Strings
Creating strings in Python is as simple as enclosing text within quotes. For example:
single_quoted = 'Hello, world!'
double_quoted = "Python Strings"
triple_quoted = '''Multi-line
String'''
String Operations and Methods
Strings in Python come equipped with a plethora of operations and methods to make manipulation a breeze. From concatenation to slicing, you can mold strings to your needs.
- Concatenation: Combine strings using the
+
operator. - Slicing: Extract substrings using index ranges.
- Length: Determine the length of a string with the
len()
function. - Case Conversion: Convert strings to lowercase or uppercase.
- Stripping: Remove leading and trailing whitespace with
strip()
. - Replacing: Replace specific substrings using
replace()
. - Splitting: Divide strings into lists using
split()
.
Advanced String Formatting
Python’s string formatting capabilities allow you to construct complex strings with ease. The format()
method and f-strings (formatted string literals) enable you to inject variables and values into strings dynamically.
Example:
name = "Alice"
age = 30
greeting = f"Hello, my name is {name} and I'm {age} years old."
Exploring Advanced String Techniques
Escape Characters: Adding Special Characters
In Python strings, you might encounter situations where you need to include special characters like newline (\n
) or tab (\t
). Escape characters provide a way to incorporate these characters without disrupting the string’s structure.
Example:
message = "Hello,\nPython!"
Raw Strings: Dealing with Backslashes
Sometimes, you might need to work with strings that contain backslashes. In such cases, raw strings (r'...'
) come to the rescue. They treat backslashes as literal characters, ensuring that escape sequences aren’t interpreted.
Example:
path = r'C:\Users\Username\Documents'
Common String Manipulation Challenges
Handling Unicode Characters
Unicode is a character encoding standard that encompasses a vast array of characters from various writing systems. Python’s support for Unicode allows you to handle characters from different languages and scripts seamlessly.
Example:
emoji = "😃"
Encoding and Decoding Strings
Encoding is the process of converting a Unicode string into a sequence of bytes, while decoding is the reverse process. Python provides a variety of encoding methods such as UTF-8, UTF-16, and more.
Example:
text = "Python is fun!"
encoded = text.encode('utf-8')
decoded = encoded.decode('utf-8')
FAQs
How do I find the length of a string?
The length of a string can be determined using the len()
function.
Can I concatenate strings of different data types?
No, Python doesn’t allow direct concatenation of strings and other data types. You need to convert non-string data types to strings before concatenation.
What’s the difference between single and double quotes for strings?
In Python, both single and double quotes can be used interchangeably to define strings. Using one inside the other can help avoid escape characters.
How do f-strings differ from the format()
method?
F-strings provide a more concise and readable way to format strings compared to the traditional format()
method. They are available in Python 3.6 and later versions.
Can I modify individual characters in a string?
No, strings in Python are immutable, meaning you can’t change individual characters. You need to create a new string with the desired modifications.
How do I handle case-insensitive string comparisons?
You can convert both strings to lowercase (or uppercase) using the lower()
method before comparison.
Conclusion
Strings are the fundamental components of textual data in Python, offering a wide array of manipulation techniques and advanced functionalities. By mastering the art of working with strings, you unlock the potential to craft elegant and efficient solutions for various programming challenges. From simple operations like concatenation to complex Unicode handling, Python strings empower developers to create versatile and dynamic applications. Armed with the knowledge gained from this tutorial, you’re now ready to embark on your string-handling journey with confidence. Happy coding!
SOURCEBAE: HIRE REACT DEVELOPER