Reversing Arrays in JavaScript
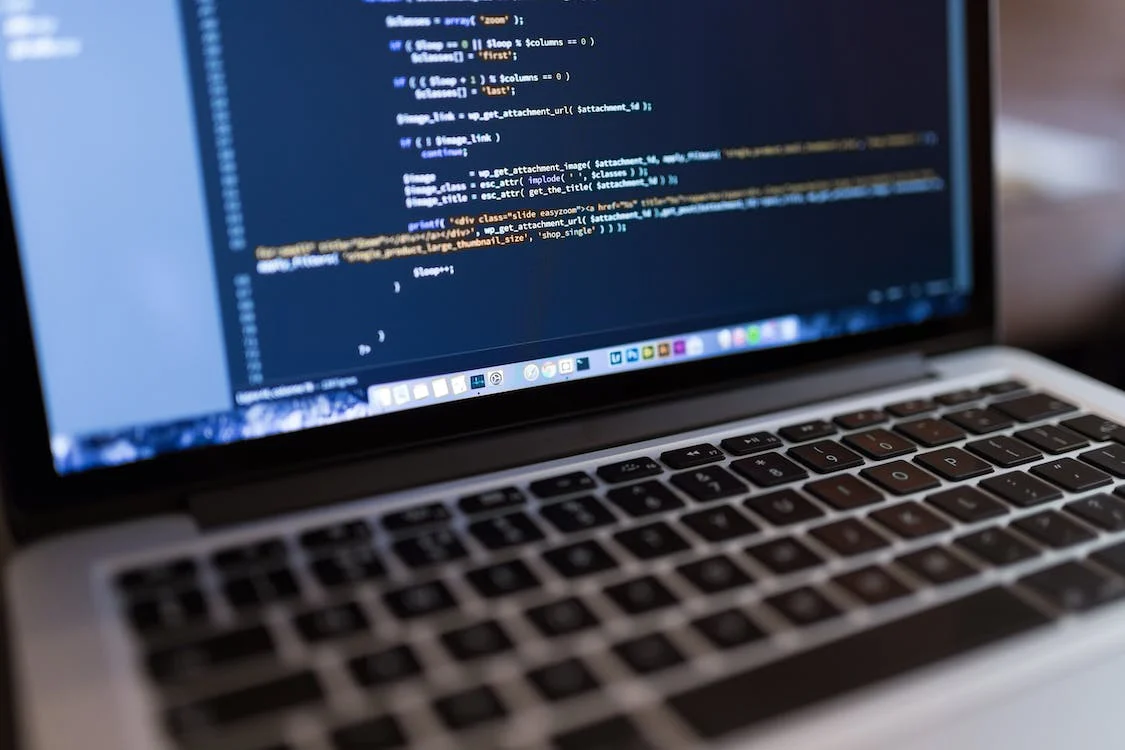
Reversing an array involves changing the order of its elements, turning the last element into the first and vice versa. JavaScript offers several methods to accomplish this, each with its advantages and use cases. Let’s explore some popular techniques:
Using the Array.prototype.reverse()
Method
The most straightforward method to reverse an array is by using the built-in reverse()
method. This method modifies the original array, reversing its elements in place. To use it, simply call the method on the array:
const originalArray = [1, 2, 3, 4, 5];
originalArray.reverse(); // The array is now [5, 4, 3, 2, 1]
Creating a Reversed Copy
In scenarios where preserving the original array is crucial, creating a reversed copy is the way to go. This approach prevents altering the original array while providing a reversed version:
const originalArray = [1, 2, 3, 4, 5];
const reversedCopy = [...originalArray].reverse(); // reversedCopy: [5, 4, 3, 2, 1], originalArray: [1, 2, 3, 4, 5]
Reversing Arrays Manually
Understanding the underlying logic of array reversal is essential for enhancing your coding skills. By manually iterating through the array, you can reverse its elements:
function reverseArrayManually(arr) {
const reversed = [];
for (let i = arr.length - 1; i >= 0; i--) {
reversed.push(arr[i]);
}
return reversed;
}
Array Spread Operator
The array spread operator offers an elegant solution for reversing arrays by spreading the elements into a new array:
const originalArray = [1, 2, 3, 4, 5];
const reversedArray = [...originalArray].reverse(); // [5, 4, 3, 2, 1]
Reversing Arrays with Recursion
Recursion is a powerful technique that can be employed to reverse arrays as well. By breaking down the problem into smaller subproblems, you can achieve array reversal:
function reverseArrayRecursively(arr) {
if (arr.length === 0) {
return [];
}
return [arr.pop()].concat(reverseArrayRecursively(arr));
}
Performance Considerations: Optimizing Your Code for Efficiency
While various techniques offer ways to reverse arrays, considering performance is crucial, especially when dealing with large datasets. The built-in reverse()
method and the array spread operator tend to be efficient due to browser optimizations. However, manual methods and recursion can lead to performance bottlenecks.
Common Mistakes to Avoid: Pitfalls and How to Sidestep Them
When working with array reversal, certain mistakes are common, often leading to unexpected outcomes. Here are some pitfalls to be aware of:
- Forgetting to Create a Copy: Modifying the original array while reversing can lead to unintended consequences. Always create a copy if preserving the original is necessary.
- Not Considering Performance: Neglecting performance considerations can result in sluggish code. Opt for efficient methods, especially with larger arrays.
- Misunderstanding Recursion: Recursion can be tricky. Ensure you understand the base case and recursive step to avoid infinite loops.
FAQs
Can I reverse an array without modifying the original?
Yes, you can create a reversed copy of the array without altering the original. Use techniques like the array spread operator or manual iteration to achieve this.
Which method is the most efficient for reversing arrays?
The built-in Array.prototype.reverse()
method and the array spread operator are generally more efficient due to browser optimizations. Consider these methods for larger arrays.
Is recursion the best approach for array reversal?
Recursion offers a unique way to reverse arrays, but it might not be the most efficient choice for large datasets. Balance your approach based on the size of the array.
How do I avoid common pitfalls while reversing arrays?
To avoid common mistakes, always create a copy of the array when needed, prioritize performance, and ensure a clear understanding of any recursive approach.
Can I use the spread operator with other array manipulation tasks?
Absolutely! The array spread operator is versatile and can be used in various array manipulation tasks beyond reversal.
What’s the benefit of mastering array reversal?
Mastering array reversal opens the door to more advanced programming tasks. It’s a fundamental skill that enhances your ability to manipulate and process data effectively.
Conclusion: Mastering Array Reversal – A Recap
In this guide, we embarked on a journey to unravel the intricacies of reversing arrays in JavaScript. We explored various methods, from the built-in reverse()
method to manual iteration and recursion. By mastering these techniques, you can confidently handle array manipulation. Consider performance, avoid common pitfalls, and tailor your approach to suit the task. With this newfound knowledge, you can excel in array manipulation and elevate your coding prowess.