Great. I’ll create an in-depth, SEO-optimized blog post on “Test Automation Frameworks” tailored for software developers and beginners in testing. The article will include clear explanations, keyword-rich content, relevant code examples or diagrams, and Yoast SEO-friendly structure. I’ll also focus on outranking the SmartBear page in Google SERP and making the content eligible for AI Overviews.
I’ll get started and let you know when the post is ready for your review.
Test Automation Frameworks
Test automation frameworks are the backbone of efficient software testing. They provide a structured approach to writing and running automated tests, ensuring consistency and reducing maintenance effort. In software testing automation, a framework establishes guidelines, tools, and practices that help QA teams develop, execute, and manage test scripts in a systematic way. This not only saves time but also improves the quality of testing by making tests more reliable and repeatable.
In software development, you might be familiar with web development frameworks (like React or Django) that streamline building applications. Similarly, a testing framework in automation is a set of rules and architecture for building test cases. Many newcomers ask questions like “what is a test framework in software development?” or even the oddly phrased “what is a test frame software dev?”.
Simply put, automation testing means using software to automatically run tests, and a test automation framework is the methodology that guides how those automated tests are structured and executed. It defines how to organize test code, handle test data, and report results. For example, a framework may specify coding standards, how to read input data from files, how to structure page object classes, and how to log test results. By following a framework, testing automation becomes more manageable and scalable as projects grow.
Modern teams use various tools with their frameworks. If you’re using Selenium for web testing, you might wonder “what is a framework in Selenium?” In practice, framework in Selenium refers to the design pattern or approach you use (data-driven, keyword-driven, etc.) to organize your Selenium test scripts. There are even ready-made frameworks like STAF (Software Testing Automation Framework) – an open-source platform for cross-platform test automation – that provide a foundation to build on. But whether you build your own or use an existing one, choosing the right framework is crucial for successful test automation in software testing.
What is a Test Framework?
A test framework is a set of guidelines or rules for creating and running tests. In the context of automation, it’s often called a test automation framework or automated testing framework. It’s not a single tool but rather a combination of practices, coding standards, and tools that work together to support automated testing. The framework defines how testers write test scripts, how those scripts are executed, and how results are handled.
For example, a framework might dictate how to structure your test files and folders, how to name your test functions, how to handle test data (external files or databases), and how to generate test reports. It may include components like:
- Driver scripts that initiate test execution
- Reusable libraries (common functions for repetitive tasks)
- Test data sources (like Excel or CSV files for inputs)
- Object repositories (to store UI element locators)
- Reporting tools (to log results and screenshots)
All these pieces work together under the framework’s guidelines. The goal is to make tests easy to write, maintain, and scale. Without a framework, automation can become chaotic – imagine every tester writing tests in their own style with no consistency. A framework brings structure and consistency, allowing teams (including beginners in testing) to collaborate and build on each other’s work. In summary, a test automation framework provides a blueprint for efficient automated testing, much like architectural frameworks guide software development.
Benefits of a Test Automation Framework
Using a test automation framework offers numerous benefits that improve the speed and quality of testing. Here are some key advantages of having a robust testing framework in place:
- Improved Test Efficiency: Automated tests run faster and more frequently. A framework optimizes this by organizing tests and eliminating redundant steps, so you can run more tests in less time.
- Lower Maintenance Costs: With structured test scripts (and no copy-paste chaos), updating tests when the application changes is easier. This reduces the effort and cost to maintain test suites over time.
- Reusability of Code: Frameworks encourage writing reusable functions or modules. Common actions (like login or navigation) can be written once and reused in many tests, avoiding duplication.
- Better Test Coverage: Because tests are easier to write and manage, teams can cover more scenarios. You can systematically approach different input data or configurations, increasing test automation coverage.
- Consistency and Standards: By following a framework, all team members adhere to the same standards (naming conventions, coding style, etc.). This consistency makes tests easier to read and review, and helps new team members (or beginners in testing) get up to speed.
- Minimal Manual Intervention: A good automation framework can run unattended. For example, it might integrate with CI/CD pipelines to trigger tests automatically on each code push, and even handle reporting. This means testing automation can happen 24/7 without constant human oversight.
- Scalability and Flexibility: As projects grow, a framework makes it simpler to scale up testing. You can add new tests without collapsing under the weight of unmanaged code. Many frameworks support parallel execution, multiple platforms, and integration with different tools – making your tests more powerful.
In essence, a framework provides a structured, efficient, and reliable approach to automated testing. It turns test automation into a true asset for the development cycle, yielding a high return on investment (ROI) by catching bugs early and freeing up testers from repetitive manual checks.
Types of Automated Testing Frameworks
There are several types of automation testing frameworks, each with its own architecture, advantages, and use cases. Choosing the right one depends on your team’s skill set and the project needs. Below we’ll explore the most common framework types:
Linear Automation Framework
A Linear Automation Framework is the simplest type of framework – it’s essentially a record-and-playback approach. Tests are written in a linear sequence, performing steps one after the other exactly as recorded or scripted. In this framework, each test case is a standalone script that runs from start to finish without modular components. This approach is straightforward and often used by beginners or for quick, simple tests.
For example, if you were testing a login function in a linear style, your script would explicitly perform each step: open the browser, navigate to the page, enter username, enter password, click login, and then check the result. All data (like the username/password) is typically hard-coded in the script.
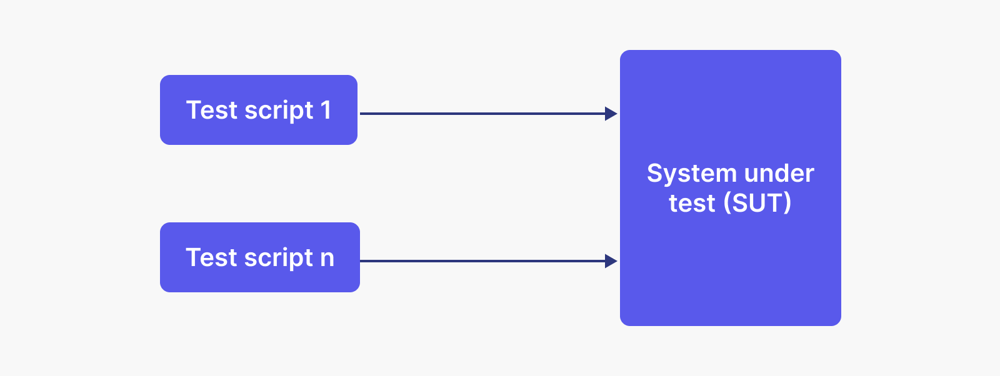
A simple depiction of a linear test automation framework. Each test script (Test script 1, Test script 2, etc.) directly interacts with the System Under Test (SUT) independently. In a linear framework, there is no reuse of code between scripts – every script contains its own actions and test data. This makes it easy to understand and create initially, but can lead to a lot of repeated code in each test case.
Advantages:
The linear framework requires no advanced programming knowledge. Testers can often use a tool’s recording feature to generate scripts quickly without writing code. It’s fast to get started – you can create automated tests in minutes by recording user actions. The sequential flow is also easy for anyone to follow, which is why it’s sometimes called “scripted testing” or “record & playback”. For a brand new automation effort (or a software dev experimenting with testing), this is the quickest way to see results.
Disadvantages:
The simplicity of linear scripts comes at a cost. The test data and flows are hard-coded, so you can’t easily rerun the same script with different data sets (no loop or parameterization). No reusability means if the same login steps are needed in 10 tests, those steps are recorded 10 times in each script. This makes maintenance a nightmare – if the application’s login process changes, you have to fix all 10 scripts individually. As the number of tests grows, a linear framework does not scale well. It’s best suited for short-term or very small projects.
Code Example – Linear Script: Here’s a quick pseudo-code example of a linear test script for a login scenario, using a Selenium-like syntax for illustration:
# Linear test script example (login test)
open_browser("https://example.com/login")
type_text("username_field", "testuser") # Enter username
type_text("password_field", "Pa$$w0rd") # Enter password
click("login_button") # Click the Login button
assert_text("welcome_message", "Welcome testuser") # Verify successful login message
close_browser()
Each step is scripted in order. This one script would only test the specific username/password given. To test different users, you’d have to create separate scripts or modify and rerun this script with new data, which isn’t efficient.
Modular Based Testing Framework
A Modular Based Testing Framework takes a more structured approach by breaking the application under test into logical modules or components. Each module (representing a part of the application or a specific functionality) has its own independent test scripts. These scripts can be combined to build larger test scenarios. Essentially, you build smaller pieces (modules) and then assemble them for complex tests, which promotes reusability.
In a modular framework, you might have a login module, a search module, a checkout module, etc., each implemented as a function or script. Your tests then call these module scripts in sequence to form a complete test case. This is similar to the concept of functions in programming – write once, reuse many times.
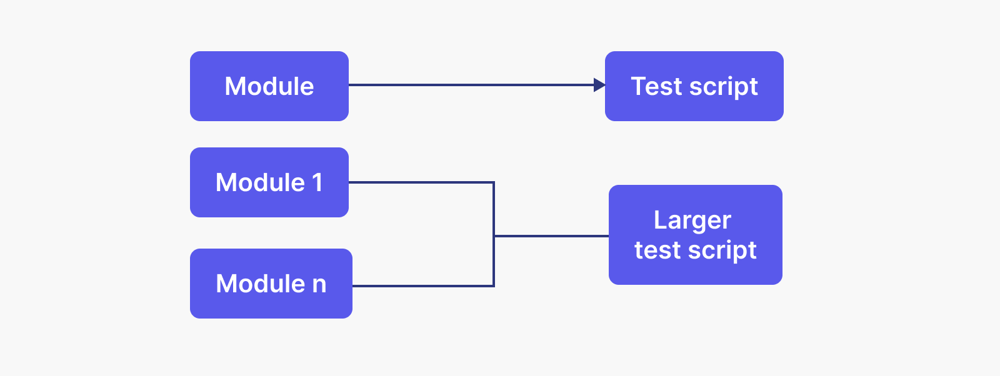
A modular test automation framework illustrated. The application is divided into modules (Module 1, Module 2, …, Module n), each with its own script. These module scripts are then assembled into larger test scripts. For example, a “Place Order” test might combine the login module, search module, and checkout module scripts into one flow. This hierarchical structure means changes in one module (say the login process) require updating only that module’s script, not every test that logs in.
Advantages:
If something changes in the application, you only need to update the corresponding module’s script. For instance, if the login page UI changes, you update the login module function, and all tests that use that login function automatically use the updated logic. This isolation makes maintenance easier and reduces duplication of code. Test creation becomes faster over time because you can reuse existing modules to create new tests (less writing from scratch). In short, modular frameworks introduce abstraction – testers don’t always rewrite low-level steps, they just call the high-level module.
Disadvantages:
Modular frameworks do require some programming skill to set up. Testers need to identify useful modules and implement them (often writing functions or methods in code). Also, at this basic modular stage, test data is often still hard-coded within each module’s script. So while code is reusable, the inability to run the same module with multiple data sets is a limitation (this is addressed by the next frameworks like data-driven). There is an upfront effort to design modules properly, which means a bit more planning than the linear approach. However, this effort pays off as the test suite grows.
Code Example – Modular Approach: To illustrate modular design, here’s a pseudo-code snippet. We create a reusable login(user, pass)
function as a module, then use it in a test case:
# Define the login module (reusable function)
def login(username, password):
open_browser("https://example.com/login")
type_text("username_field", username)
type_text("password_field", password)
click("login_button")
# Define another module for verification (just as an example)
def verify_welcome(expected_user):
assert_text("welcome_message", f"Welcome {expected_user}")
# Test case using the modules
login("testuser", "Pa$$w0rd") # reuse the login module with specific data
verify_welcome("testuser") # reuse verification module
logout() # (assume logout is another module defined elsewhere)
In this example, if we need to create a new test for a different user, we can call login("newuser", "NewPass")
without rewriting the login steps. The modules make it easy to construct tests flexibly.
Library Architecture Testing Framework
The Library Architecture Testing Framework is an extension of the modular approach. It identifies common tasks within your test scripts and groups those into libraries of reusable functions. The difference from basic modular frameworks is a greater focus on building a shared library that all tests can use. It’s as if you’re creating your own custom automation toolbox (functions library) for your application or testing needs.
In practice, after writing a few modular scripts, you might notice several scripts perform similar sequences (e.g., many tests log in at some point, or many tests add a product to the cart). With library architecture, you abstract these sequences into common library functions. For example, you might create a library file with functions like login(user, pass)
, addProductToCart(product)
, checkoutOrder()
and so on. Tests then call these library functions, sometimes in combination with module scripts, to accomplish test cases.
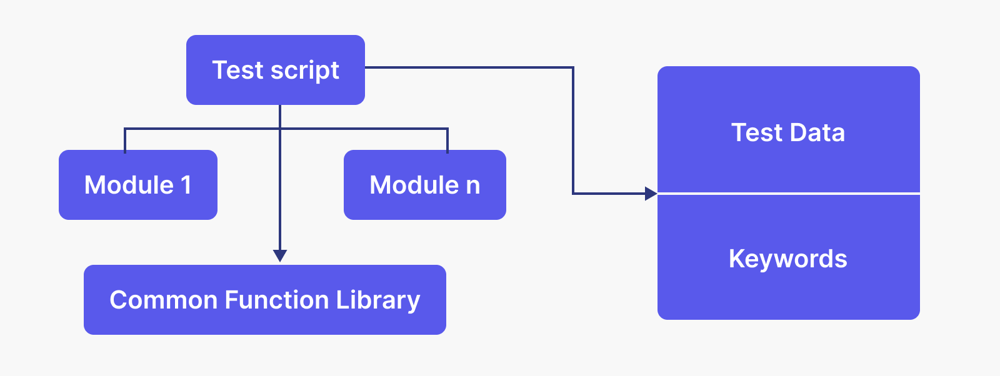
Illustration of a library architecture framework. Here, common function libraries are created for shared tasks. The test script uses those library functions (and can also utilize smaller modules) instead of containing the detailed steps each time. This design maximizes code reusability and maintainability – if something changes, you update the function in the library. The diagram also shows that library frameworks can still incorporate external test data and keyword tables for flexibility, making it a powerful hybrid-like structure.
Advantages:
High reusability and maintainability are the hallmarks of the library architecture. Since every common action resides in one place (the library), any update is done once. All tests benefit from the fix immediately. This framework also encourages a clean separation of concerns: your test cases become short and readable, since they call well-named functions like login()
or searchProduct()
rather than dozens of low-level steps. It’s easier to scale the test suite because adding a new test often just means writing a few lines that combine existing library calls in a new order. Overall, this approach yields a very structured framework that can handle large test suites.
Disadvantages:
The initial setup can be time-consuming. Building a robust library requires skilled test automation engineers who can identify patterns and implement functions for them. It’s essentially a bit of development work (creating a mini software framework for your tests). If not designed properly, there’s a risk of creating too many or too few library functions. Also, team members need to learn how to use the libraries. However, once the investment is made, the long-term payoff in reduced maintenance is significant.
Note: The line between “modular” and “library architecture” frameworks can blur. Often, people consider the library approach as just a more refined modular framework. The key distinction is the emphasis on a common function library accessible to all tests.
Also read: Different Parts of a Test Automation Framework
Data-Driven Framework
A Data-Driven Testing Framework separates test logic from test data. This means the steps of the test script are fixed, but the input values (and expected outcomes) are pulled from an external data source – such as Excel spreadsheets, CSV files, databases, or JSON files. Instead of hard-coding usernames, passwords, and other test inputs in your script, you read them from these external files. This allows the same test script to run multiple times with different data sets (hence covering different scenarios with one script).
This framework is extremely useful when you have test scenarios that need to be repeated with various inputs. For example, consider a login functionality: you want to test login with multiple sets of credentials – valid ones, invalid ones, empty fields, etc. With a data-driven approach, you can write one login test script and supply it with a list of username/password combinations to try. The script will iterate through the data, executing the same steps for each combination.
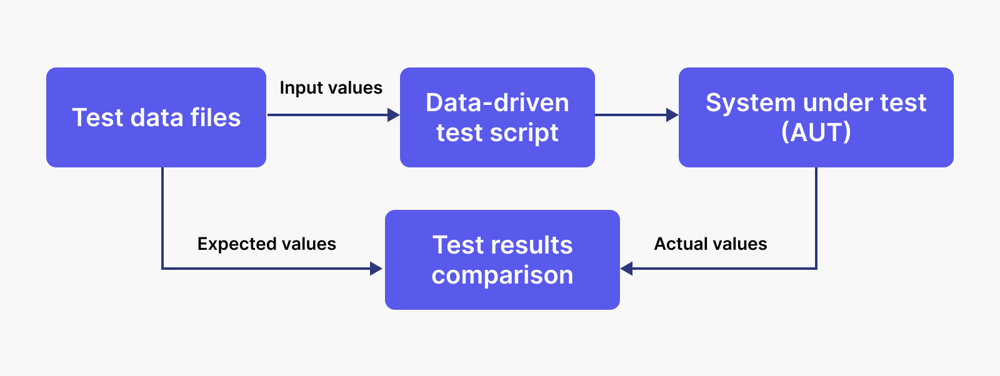
A conceptual diagram of a data-driven framework. Test data files (on the left) provide input values to the data-driven test script, which interacts with the System Under Test (AUT). The outcomes are then evaluated in a test results comparison against expected values (which may also come from the data files). By isolating data from the test script, you can quickly adjust or add new test cases by simply updating the external data – without changing the code of the test script.
Advantages:
Data-driven frameworks dramatically increase test coverage without multiplying the number of scripts. You can test numerous input combinations by driving them through one script. This saves time (both in writing and execution) and ensures consistency across test runs. Hard-coding of data is eliminated, so if the source data changes (say you update the spreadsheet), you don’t need to modify the script – it will pick up new data on the next run. This also reduces manual errors; testers aren’t manually typing in values each time, the framework feeds them in automatically. In short, it’s efficient for testing multiple scenarios quickly and is especially popular in scenarios like form validations, calculations, or any input-heavy application.
Disadvantages:
Setting up a data-driven framework requires more technical skill. You need to write code to read from external sources and loop through data sets. If using tools like TestNG (for Java) or PyTest (for Python), you would use features like data providers or parameterized tests. It also takes time to organize the data and keep it up-to-date (maintaining the external data files becomes part of test maintenance). Another consideration: debugging failures can be a bit more complex, since one script runs many iterations – you have to pinpoint which data set caused an issue. Despite these challenges, the ROI is high for applications that must be tested with many different inputs.
Code Example – Data-Driven: Below is a pseudo-code snippet demonstrating a data-driven approach for a login test. It uses a list of tuples as dummy data inputs:
# Sample data sets for testing (could be from a file or database in a real scenario)
test_data = [
{"username": "validUser1", "password": "Correct#1", "expected": "Login Success"},
{"username": "validUser2", "password": "WrongPass", "expected": "Login Failed"},
{"username": "", "password": "NoUser", "expected": "Login Failed"}, # empty username
]
for data in test_data:
open_browser("https://example.com/login")
type_text("username_field", data["username"])
type_text("password_field", data["password"])
click("login_button")
result = get_text("status_message")
assert result == data["expected"] # check if outcome matches expected result
close_browser()
In a real test, test_data
might be loaded from an external file. The loop runs the same sequence for each set of data. This one script effectively conducts three test cases in one go (and you can add more cases easily by adding data).
Keyword-Driven Framework
A Keyword-Driven Testing Framework (also known as table-driven or action word framework) takes the concept of separation further by abstracting the test steps into keywords. In this approach, tests are designed as tables of high-level actions (keywords) rather than code. Each keyword corresponds to a function that performs a certain action (for example, a keyword Login might internally execute all steps needed to log in, or a keyword Click might perform a click action on whatever element is specified).
Testers using a keyword-driven framework typically create a spreadsheet or table where each row represents a test step. The columns might include the Keyword to execute, the target element (or data) for that keyword, and any input value needed. The test execution engine reads this table row by row and triggers the corresponding actions in the automation tool.
For instance, a login test case in a keyword table might look like this (simplified):
Step | Keyword | Object (Target) | Value |
---|---|---|---|
1 | OpenBrowser | (browser) | Chrome |
2 | NavigateTo | URL | https://example.com |
3 | Click | login_link | (none) |
4 | TypeText | username_field | testuser |
5 | TypeText | password_field | Pa$$w0rd |
6 | Click | login_button | (none) |
7 | VerifyText | welcome_message | Welcome testuser |
In this table, “OpenBrowser”, “NavigateTo”, “Click”, “TypeText”, and “VerifyText” are all keywords. The framework would have functions implemented for each of these keywords. The tester doesn’t need to code those each time—just use the keywords with appropriate parameters.
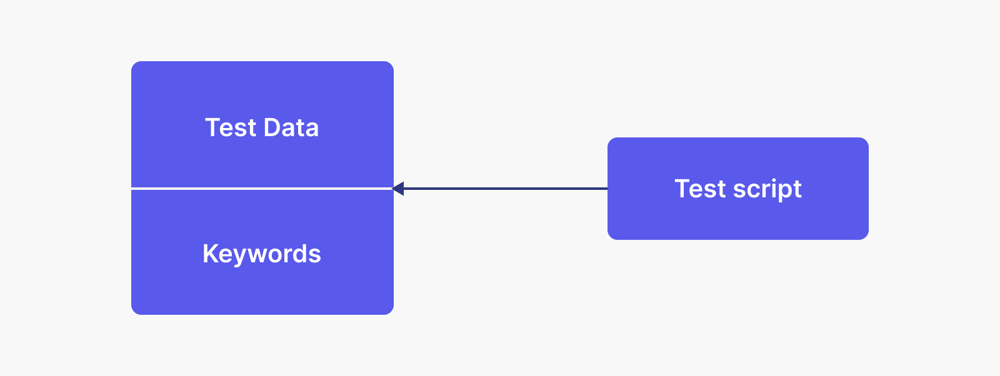
A simplified view of a keyword-driven framework. The test logic is controlled by external data: keywords and test data (often stored in spreadsheets or tables). The automation script reads the keyword instructions and executes the corresponding actions on the application. This separation means even non-programmers can create or understand test cases by reading the keyword table. Each keyword like “Click”, “TypeText”, or “VerifyText” represents a function call under the hood, enabling a form of plain English testing instructions.
Advantages:
The big benefit of keyword-driven frameworks is that they require minimal scripting knowledge to design tests. Once the automation engineers set up the underlying keyword functions, testers (or even business analysts) can write new test cases by mixing and matching keywords in a spreadsheet. It’s highly reusable because one keyword (say Login
) can be used in many test cases. Tests are also tool-agnostic to an extent – the keyword “Click” can work with any UI; if you change automation tools, you just re-implement the Click
function, but your test cases (tables) remain the same. This approach improves collaboration between non-technical test designers and automation engineers, as the former can design tests in a readable format.
Disadvantages:
The initial setup is complex and time-consuming. You need a solid framework to read keywords from files and map them to functions in the automation tool. It requires creating and maintaining potentially large keyword libraries and object repositories (for target elements). Scaling a keyword framework can be challenging – as the application grows, you keep adding new keywords and it can become difficult to manage if not organized well. Also, debugging test failures might require digging into both the test table and the underlying code, which can be tricky. It’s recommended to have good documentation for each keyword so users of the framework know how to use them properly.
Code Example – Keyword Execution: To visualize how a keyword-driven execution might look in code, consider this very simplified pseudo-code snippet:
# Define some keyword functions (normally, these would be more complex and part of the framework's library)
def OpenBrowser(browser_name):
# code to launch browser
pass
def Click(element_name):
# code to find element by name and click
pass
def TypeText(element_name, text):
# code to find element and type text
pass
def VerifyText(element_name, expected):
# code to assert text on element matches expected
pass
# Keyword mapping (could be a dictionary)
keywords = {
"OpenBrowser": OpenBrowser,
"Click": Click,
"TypeText": TypeText,
"VerifyText": VerifyText
}
# Example of executing steps from a keyword table:
test_steps = [
{"keyword": "OpenBrowser", "args": ["Chrome"]},
{"keyword": "NavigateTo", "args": ["https://example.com"]},
{"keyword": "Click", "args": ["login_link"]},
{"keyword": "TypeText", "args": ["username_field", "testuser"]},
{"keyword": "TypeText", "args": ["password_field", "Pa$$w0rd"]},
{"keyword": "Click", "args": ["login_button"]},
{"keyword": "VerifyText", "args": ["welcome_message", "Welcome testuser"]}
]
for step in test_steps:
kw = step["keyword"]
func = keywords.get(kw)
if func:
func(*step["args"])
In a real framework, NavigateTo
would be another keyword function, and we’d also handle reading this list from an external file. But this pseudo-code demonstrates the idea: each step is just data (keyword + arguments), and the test runner loops through them to execute actions.
Hybrid Test Automation Framework
As the name suggests, a Hybrid Test Automation Framework is a blend of two or more of the above frameworks, combining their strengths to suit the team’s needs. In practice, most real-world test automation frameworks end up being hybrid. For example, you might use a data-driven approach within a keyword-driven framework: keywords define the test flow, but the data for each keyword comes from an external file. Or you might combine modular and data-driven: reusable modules for actions, but feed them with external data sets.
The goal of a hybrid framework is to be flexible and comprehensive. Teams pick and choose elements that solve their problems. If you need non-programmers to contribute, you incorporate keywords; if you have lots of test data, you add data-driven capabilities; if you want reuse, you implement libraries/modules. A well-designed hybrid framework can greatly optimize testing for complex applications.
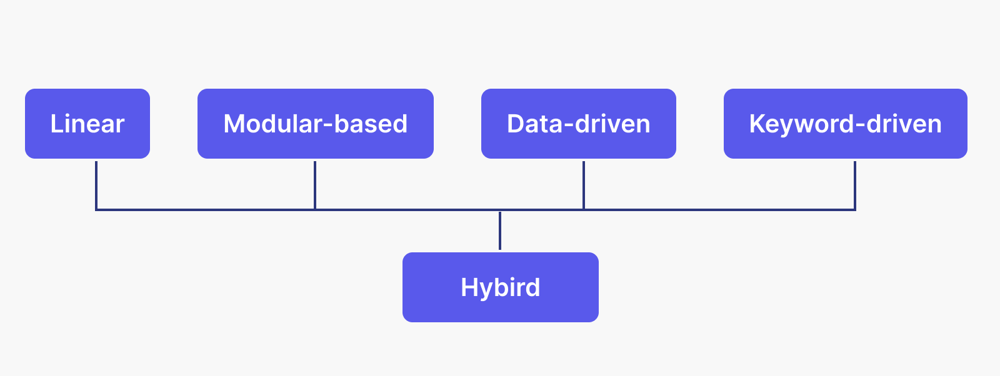
Hybrid framework concept: the diagram shows that the hybrid approach can incorporate Linear, Modular, Data-Driven, and Keyword-Driven aspects all into one framework. In a hybrid framework, you leverage the best practices from multiple approaches. For instance, you might create modular functions (library architecture), use a data-driven strategy to supply test inputs, and allow some tests to be defined by keywords for clarity. Hybrid frameworks are very popular because they can be tailored to virtually any project.
Advantages:
Maximum flexibility – the framework can handle various scenarios. It can evolve over time; you might start with modular scripts, then add a data-driven layer as the need arises, and perhaps introduce keywords for a subset of tests. This way, the framework is not constrained to one style. Higher efficiency can be achieved by mitigating the weaknesses of one approach with another’s strengths. For example, linear scripts’ maintenance nightmare is solved by the modular approach, and modular’s lack of data variation is solved by data-driven, and so on. A hybrid framework can also integrate multiple tools (e.g., using Selenium WebDriver for web actions, plus an API testing tool for back-end verifications, all under the same framework).
Disadvantages:
Designing a hybrid framework can be complex. It’s essentially building a custom solution for your organization. It requires careful planning and skilled engineers to integrate different components. There’s also a learning curve for the team to understand how the framework works (since it may have multiple layers – e.g., data sheets, keywords, function libraries, etc.). Documentation and organization are key; without them, a hybrid approach could become messy. However, when done right, the payoff is a robust framework that can handle just about anything.
Conclusion
In the world of test automation, frameworks are indispensable for achieving reliable and scalable results. We’ve discussed multiple types – linear, modular, library architecture, data-driven, keyword-driven, and hybrid – each offering a different balance of simplicity, reusability, and power. There’s no one-size-fits-all answer to “what are the frameworks in Selenium or in testing?”; in fact, many Selenium test projects use a hybrid framework that combines data-driven and keyword-driven techniques (and often the Page Object Model pattern as well) to create a maintainable test suite. The key is to choose or design a framework that fits your team’s skills and your application’s needs.
When selecting a framework, consider the application type, the size of the test suite, who will design/write the tests (automation engineers vs. manual testers), and what tools you’re using. For example, a small project might get by with a linear or modular framework, but a large enterprise application would benefit from a data-driven or hybrid approach for better coverage. Always keep in mind the benefits of test automation frameworks: they should make your life easier by organizing the chaos of automated tests into a manageable system.
In conclusion, test automation in software testing is much more effective with a well-built framework. It means the difference between a brittle set of scripts and a robust, industrial-strength testing solution. By investing time in the right framework – whether it’s implementing a popular one or creating a custom hybrid – you set the foundation for quality software releases. The result is faster testing cycles, higher confidence in software quality, and the ability to catch issues early in development. With this knowledge of frameworks, you’re better equipped to implement automation successfully and even explain to others what a test automation framework is and why it matters in today’s software development landscape.
Also read: What Does a Test Automation Architect Do?