The Ultimate Guide to the ReactJs Framework
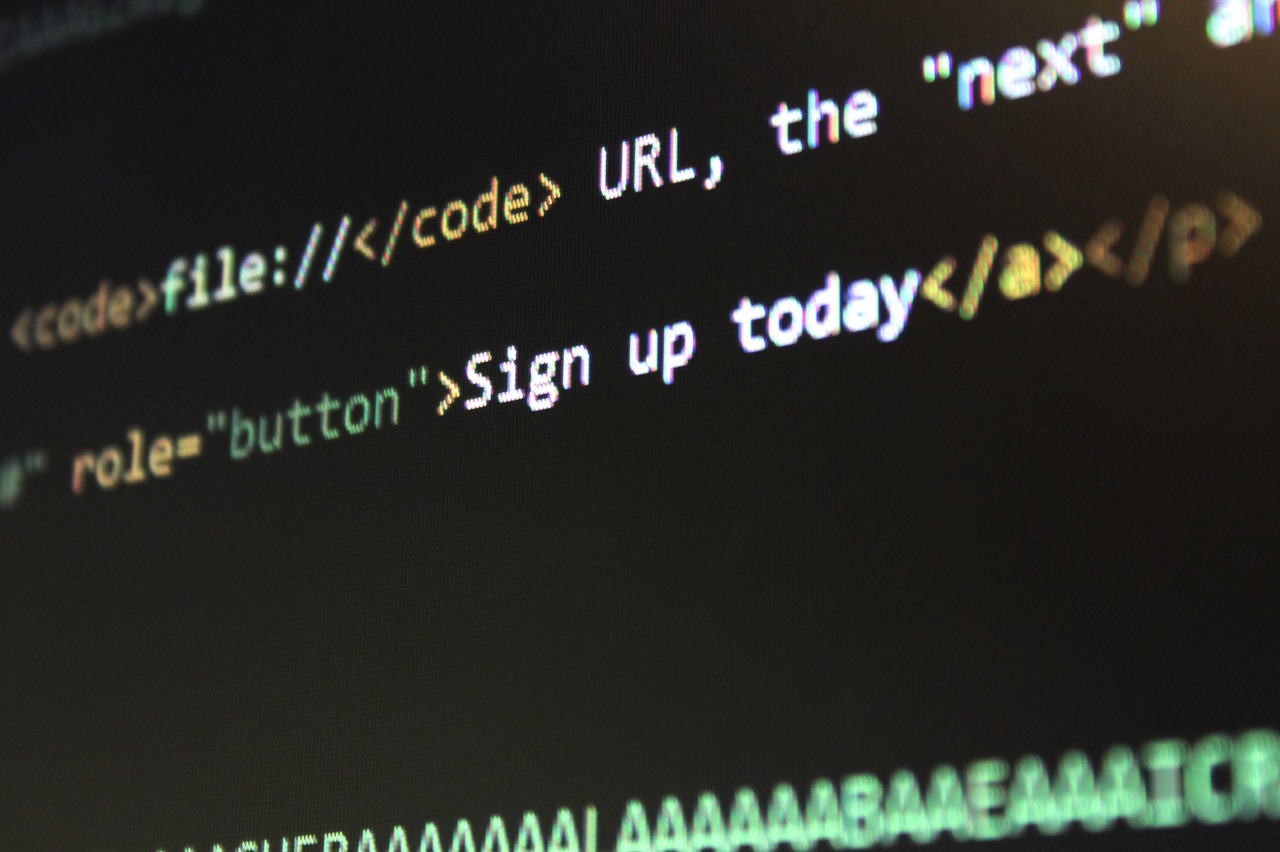
When it comes to web development, the ReactJs Framework stands as a shining star in the realm of front-end technologies. Its ability to create dynamic, responsive, and interactive user interfaces has revolutionized the way developers approach building web applications.
In this guide, we’ll dive deep into the ReactJs Framework, exploring its core concepts, advantages, use cases, and more. So, whether you’re a seasoned developer looking to expand your skill set or a beginner eager to understand the world of web development, this guide has you covered.
ReactJs Framework: Unveiling the Basics
The ReactJs Framework, often simply referred to as React, is an open-source JavaScript library developed by Facebook. It focuses on building user interfaces (UIs) for web applications, providing developers with a robust set of tools and components that make the creation of dynamic UIs seamless and efficient. React operates on a component-based architecture, allowing developers to create reusable UI elements and manage their state and behavior effectively.
Key Features of ReactJs Framework
ReactJs Framework comes packed with an array of features that empower developers to craft exceptional web applications. Some of its standout features include:
1. Virtual DOM for Enhanced Performance
The Virtual DOM, a core concept in React, enables efficient updates to a web page by minimizing direct manipulation of the actual DOM. This results in improved performance and a smoother user experience.
2. Component Reusability
React’s modular approach encourages developers to build reusable components that can be easily integrated into different parts of an application. This not only streamlines development but also enhances code maintainability.
3. JSX: Combining JavaScript and HTML
JSX, or JavaScript XML, is a syntax extension that allows developers to write HTML-like code within their JavaScript. This simplifies the process of defining UI components and their structure.
4. Unidirectional Data Flow
React follows a unidirectional data flow, ensuring that changes in the application’s state trigger predictable updates to the UI. This promotes better code organization and debugging.
Leveraging ReactJs Framework: Use Cases and Benefits
ReactJs Framework has found its way into various industries and use cases, thanks to its versatility and power. Some of the prominent applications include:
1. Building Single-Page Applications (SPAs)
React is well-suited for developing SPAs, where dynamic content is loaded seamlessly without the need for full page reloads. This leads to faster load times and a more fluid user experience.
2. Crafting User-Interactive Interfaces
The ReactJs Framework excels in creating interfaces that respond to user interactions in real time. This is particularly useful for applications that require instant updates based on user actions.
3. Developing Mobile Applications
With the advent of React Native, a framework derived from React, developers can extend their skills to build cross-platform mobile applications using familiar React concepts.
4. Collaborative User Interfaces
React’s ability to manage UI components’ state in real time makes it an ideal choice for applications that demand collaborative features, such as real-time editing and commenting.
Exploring Advanced Concepts
As you delve deeper into ReactJs Framework, you’ll encounter advanced concepts that elevate your development skills. Some of these include:
1. State Management with Redux
Redux, a popular state management library, complements React by providing a centralized store for managing application state. It’s particularly beneficial for complex applications with extensive data flows.
2. Routing with React Router
React Router enables developers to create seamless navigation experiences within their single-page applications. It allows for dynamic routing and rendering of components based on the application’s URL.
3. Testing and Debugging Strategies
Robust testing and debugging are crucial in software development. ReactJs Framework supports tools and methodologies for efficient testing and debugging of components, ensuring high-quality applications.
FAQs about ReactJs Framework
Can ReactJs Framework be used for building full-stack applications?
Yes, while ReactJs Framework is primarily focused on the front-end, it can be integrated with back-end technologies to build full-stack applications. Tools like Node.js and Express can be employed to handle server-side logic.
Is ReactJs Framework suitable for beginners?
Absolutely! React’s extensive documentation, strong community support, and a wealth of online tutorials make it accessible to beginners. Its component-based architecture also promotes code organization and learning.
What is the key difference between ReactJs and React Native?
ReactJs is tailored for building web applications, while React Native is used for developing mobile applications. React Native allows you to use React’s components and principles to create native mobile experiences.
How does ReactJs contribute to SEO optimization?
ReactJs Framework is known for its efficient rendering of components and virtual DOM. While it’s JavaScript-based, implementing server-side rendering (SSR) can improve SEO by providing search engines with pre-rendered content.
Can I migrate an existing project to ReactJs?
Yes, it’s possible to migrate an existing project to ReactJs Framework. However, the complexity of the migration process depends on the size and architecture of the project. Planning and thorough understanding of React’s concepts are essential.
What are the limitations of ReactJs?
While ReactJs Framework offers numerous benefits, it’s not without limitations. One limitation is a steeper learning curve for beginners due to its component-based structure. Additionally, integrating complex animations can sometimes be challenging.
Conclusion
In the ever-evolving landscape of web development, the ReactJs Framework continues to shine as a powerful tool for creating captivating user interfaces and seamless user experiences.
Its component-based approach, virtual DOM, and vast ecosystem of tools have cemented its position as a go-to choice for developers worldwide.
By harnessing the capabilities of ReactJs, you’re not just building web applications; you’re shaping the future of digital interaction.