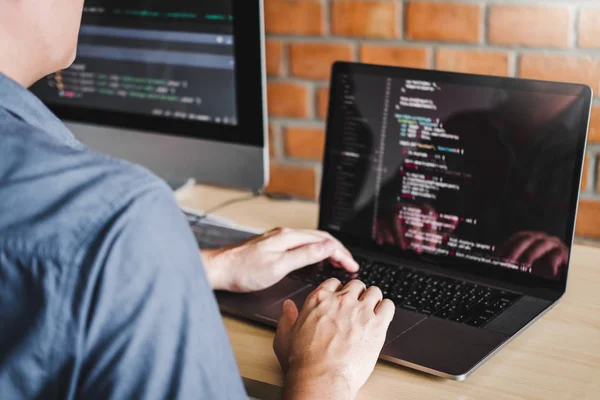
What Is Diamond Problem in Java?
Java programming, renowned for its versatility and power, is not without its quirks and challenges. One such enigma that often perplexes developers is the “Diamond Problem.” In this comprehensive guide, we will delve deep into this issue, providing you with a thorough understanding of what the Diamond Problem in Java is, its implications, and how to effectively address it. So, fasten your seatbelts as we embark on this journey into the intricate world of Java programming.
The Diamond Problem is a perplexing issue in object-oriented programming languages, especially Java. It occurs when a class inherits from two or more classes that have a common ancestor. This common ancestor creates ambiguity, leading to a situation where the compiler cannot determine which inherited method to call, resulting in a compilation error.
The Origin of Ambiguity
To understand the Diamond Problem better, let’s explore its origins. Imagine we have a class hierarchy where Class A
is the parent, and both Class B
and Class C
inherit from it. Now, a third class, Class D
, inherits from both Class B
and Class C
. This scenario forms a diamond-shaped inheritance structure.
Class A
/ \
Class B Class C
\ /
Class D
The Conflict Emerges
When Class D
inherits from both Class B
and Class C
, it inherits the methods and attributes of Class A
as well. Now, if both Class B
and Class C
override a method from Class A
, it creates a conflict for Class D
. It doesn’t know which overridden method to use, leading to ambiguity.
The Compilation Error
This ambiguity becomes evident during compilation when the Java compiler attempts to resolve which method to invoke. As a result, it throws a compilation error, preventing the program from running until the ambiguity is resolved.
Implications of the Diamond Problem
The Diamond Problem can have significant implications for Java developers and their projects:
- Compilation Errors: As mentioned earlier, the most immediate consequence of the Diamond Problem is compilation errors. These errors can be time-consuming to debug and fix.
- Code Maintenance: When a project’s codebase becomes complex, managing and resolving Diamond Problem-related issues can become a significant challenge, leading to increased development time and effort.
- Performance Overhead: In certain cases, the ambiguity resolution mechanism can introduce a performance overhead, affecting the application’s efficiency.
- Reduced Code Reusability: The Diamond Problem can limit code reusability, as developers might need to make adjustments to classes to resolve conflicts.
Handling the Diamond Problem
Using Interfaces
One effective way to tackle the Diamond Problem in Java is by using interfaces. Instead of inheriting multiple classes, Class D
can implement multiple interfaces, each defining a set of methods. This approach eliminates the ambiguity, as interfaces don’t provide method implementations.
interface InterfaceA {
void someMethod();
}
interface InterfaceB {
void anotherMethod();
}
class ClassB implements InterfaceA {
public void someMethod() {
// Implementation
}
}
class ClassC implements InterfaceB {
public void anotherMethod() {
// Implementation
}
}
class ClassD implements InterfaceA, InterfaceB {
// No ambiguity here
}
Prioritizing Classes
Another way to resolve the Diamond Problem is by explicitly specifying which superclass method to call in the subclass. This can be done using the super
keyword.
class ClassD extends ClassB {
public void someMethod() {
super.someMethod(); // Calls ClassB's method
}
}
FAQs
Q: Can the Diamond Problem occur in other programming languages besides Java?
A: Yes, the Diamond Problem is not exclusive to Java. It can also occur in other object-oriented programming languages that support multiple inheritance.
Q: Are there any real-world examples where the Diamond Problem could be encountered?
A: Yes, scenarios involving complex class hierarchies in software development can lead to the Diamond Problem. For instance, when dealing with GUI frameworks or database libraries.
Q: How can I avoid the Diamond Problem in my Java projects?
A: You can avoid the Diamond Problem by carefully designing your class hierarchies, preferring interfaces over multiple inheritance, and using the super
keyword when necessary.
Q: Does the Diamond Problem affect performance?
A: In some cases, resolving the ambiguity caused by the Diamond Problem can introduce a slight performance overhead. However, this impact is generally negligible.
Q: Are there any tools or IDEs that can help detect and resolve the Diamond Problem?
A: Yes, modern integrated development environments (IDEs) like IntelliJ IDEA and Eclipse often provide warnings and suggestions to help developers identify and address the Diamond Problem.
Q: Can the Diamond Problem lead to runtime errors?
A: No, the Diamond Problem is a compilation error. It prevents the code from being compiled, so it cannot lead to runtime errors.
Conclusion
In the intricate world of Java programming, understanding and effectively addressing the Diamond Problem is essential for writing robust and error-free code. By following best practices, such as prioritizing interfaces over multiple inheritance and using the super
keyword when needed, developers can navigate this challenge with confidence. So, go ahead, embrace the world of Java, and conquer the Diamond Problem with finesse!
READ MORE: HIRE REACT DEVELOPER