What is Variable in JavaScript?
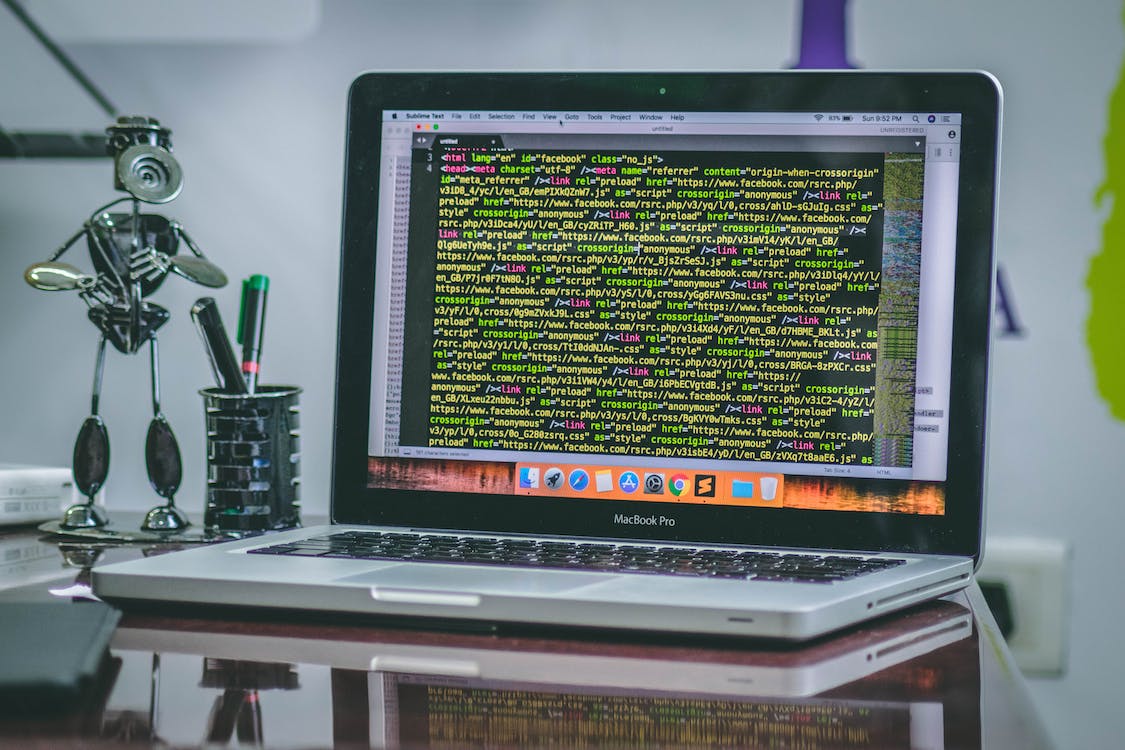
What is a Variable in JavaScript?
JavaScript, a versatile and widely-used programming language, plays a crucial role in web development. To harness its power effectively, it’s essential to grasp the foundational elements of the language. One such essential concept is variables. In this article, we’ll delve into the intricacies of variables in JavaScript, from their definition to their practical applications.
A variable in JavaScript is a symbolic name that represents a value in a program. It acts as a container for storing different types of data, such as numbers, strings, or objects. This dynamic storage capability allows developers to manipulate and work with data efficiently.
Variables enable you to store and retrieve data throughout your code, making it easier to manage complex operations and calculations. They act as placeholders, holding values that can change or remain constant depending on the needs of your program.
The Significance of Variables:
Variables serve as a fundamental building block in programming for several reasons:
- Data Storage: Variables allow you to store and manage data, making it accessible across different parts of your code.
- Data Manipulation: By assigning new values to variables, you can perform various operations, calculations, and manipulations on the data they hold.
- Code Readability: Meaningful variable names enhance the clarity of your code, making it easier for both you and other developers to understand its functionality.
- Flexibility: With variables, you can write dynamic and adaptable code that responds to changing conditions and user interactions.
Understanding Variable Declaration and Assignment:
To use a variable in JavaScript, you need to declare and assign a value to it. The declaration involves using the var
, let
, or const
keyword, followed by the variable name. Let’s explore the different ways to declare and assign variables:
- var: The
var
keyword declares a variable globally or within a function, regardless of block scope. It’s considered outdated due to its scoping behavior, which can lead to unexpected results. - let: Introduced in ECMAScript 6 (ES6), the
let
keyword declares variables with block scope. This means they’re limited to the block, statement, or expression where they’re defined. - const: Also introduced in ES6, the
const
keyword declares variables with block scope, likelet
. However, variables declared withconst
cannot be reassigned after their initial value is set.
Using Variables in JavaScript:
Variables play a vital role in a wide range of programming tasks. Here are some common ways they’re used:
- Storing User Input: When building interactive websites, you can use variables to store and process user input, such as form data.
- Performing Calculations: Variables are essential for performing mathematical calculations, tracking scores, or generating dynamic content.
- Iterating through Arrays: Variables are commonly used as index counters when iterating through arrays, enabling you to access and manipulate array elements.
- Dynamic Styling: When working with CSS in JavaScript, variables can hold style properties that you want to apply dynamically to HTML elements.
- API Requests: Variables are used to store URLs and parameters for making API requests, helping retrieve and display real-time data.
Best Practices for Using Variables:
To write clean, efficient, and maintainable code, consider these best practices when working with variables in JavaScript:
- Use Descriptive Names: Choose meaningful variable names that reflect the purpose of the data they hold.
- Declare Variables Properly: Opt for
let
orconst
overvar
to ensure consistent scoping and avoid unexpected behaviors. - Keep Scope in Mind: Be mindful of the scope in which you declare variables to prevent unintended side effects.
- Initialize Variables: Always initialize variables with an initial value to avoid undefined or null references.
- Avoid Global Variables: Minimize the use of global variables, as they can lead to naming conflicts and make code harder to maintain.
- Use Constants: If a value shouldn’t change throughout the program, use
const
to indicate its immutability.
FAQs about Variables in JavaScript:
Q: Can I change the value of a variable declared with const
?
A: No, variables declared with const
are immutable after their initial assignment. You cannot reassign them to a new value.
Q: What happens if I declare a variable without using any keyword?
A: If you declare a variable without any keyword (var
, let
, or const
), it becomes a global variable, which can lead to unintended consequences.
Q: How do I choose between let
and const
for declaring variables?
A: Use let
when the value of the variable needs to change over time. Use const
when the value should remain constant throughout the program.
Q: Can I use spaces in variable names?
A: No, variable names cannot contain spaces. Use camelCase or snake_case to improve readability.
Q: What is variable hoisting?
A: Variable hoisting is a JavaScript behavior where variable declarations are moved to the top of their scope during compilation, allowing you to use variables before they’re declared.
Q: Are variables case-sensitive in JavaScript?
A: Yes, variable names are case-sensitive. myVariable
and MyVariable
are treated as two separate variables.
Conclusion:
In the world of JavaScript programming, variables are the cornerstone of data manipulation and flexibility. By understanding how to declare, assign, and use variables effectively, you’ll unlock the ability to create dynamic, interactive, and powerful applications. So, next time you write JavaScript code, remember the vital role that variables play in shaping the functionality and behavior of your programs.
READ MORE | How to Become a React Developer?