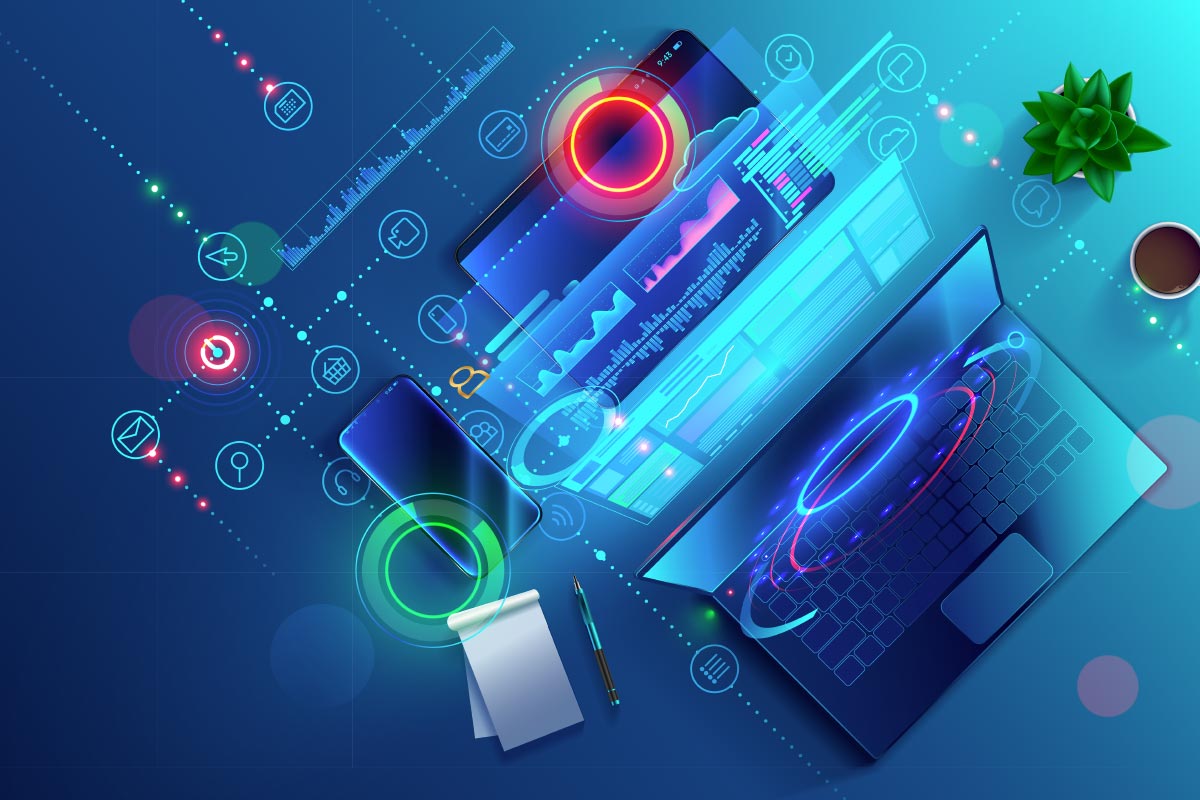
The JavaScript Fetch API is a powerful and flexible tool for making network requests in the browser. It allows you to fetch resources (such as data from a server) and manipulate them seamlessly. Unlike its predecessor, XMLHttpRequest, Fetch API offers a more modern and streamlined way to work with HTTP requests. Let’s dive into the key aspects of Fetch API:
Understanding Fetch API Basics
Fetch API simplifies making HTTP requests by providing a clean and intuitive interface. It uses promises to handle asynchronous operations, making your code more readable and maintainable. With Fetch API, you can perform various types of requests, including GET, POST, PUT, DELETE, and more.
Fetch API in Action
Let’s see Fetch API in action. Suppose you want to retrieve data from an external API:
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
In this example, Fetch API fetches data from ‘https://api.example.com/data,’ parses the response as JSON, and logs it to the console. It’s concise and elegant.
Benefits of Fetch API
Fetch API offers several advantages:
- Simplicity: Its straightforward syntax makes it easy to use.
- Promise-based: Asynchronous operations are handled cleanly with promises.
- Streaming: You can stream responses, which is great for handling large files.
- Customization: Fetch requests are highly configurable, allowing you to set headers, methods, and more.
- Cross-Origin Requests: Fetch API supports cross-origin requests, enabling you to fetch data from different domains.
Common Use Cases
Fetch API is versatile and can be used in various scenarios:
- Fetching Data: Retrieve data from RESTful APIs or other web services.
- Uploading Data: Send data to a server using POST or PUT requests.
- Handling Authentication: Include authentication tokens in headers for secure requests.
- Error Handling: Implement robust error handling to gracefully deal with network issues.
FAQs (Frequently Asked Questions)
Q: How does Fetch API differ from XMLHttpRequest?
Fetch API is more modern and user-friendly than XMLHttpRequest. It uses promises, which simplifies asynchronous code, and provides a cleaner syntax for making requests.
Q: Can I use Fetch API in all browsers?
Fetch API is supported in all modern browsers, but for older browsers, you may need to use a polyfill or consider other methods.
Q: Is Fetch API suitable for handling file uploads?
Yes, Fetch API can handle file uploads by sending data as a FormData object.
Q: Are there any security considerations when using Fetch API?
Security is a crucial aspect. Always validate and sanitize user input and use HTTPS for secure communication.
Q: How can I handle errors with Fetch API?
You can use the .catch()
method to handle errors gracefully and provide meaningful feedback to users.
Q: Can Fetch API be used for cross-origin requests?
Yes, Fetch API supports cross-origin requests, but you need to configure CORS (Cross-Origin Resource Sharing) on the server-side.
Conclusion
The JavaScript Fetch API is a game-changer in web development, offering simplicity, flexibility, and power when it comes to making network requests. Whether you’re building a dynamic web application, fetching data from an API, or handling user authentication, Fetch API has got you covered. Embrace this modern tool, stay ahead in the web development game, and unlock endless possibilities.