Top 10 PHP Interview Questions and Answers
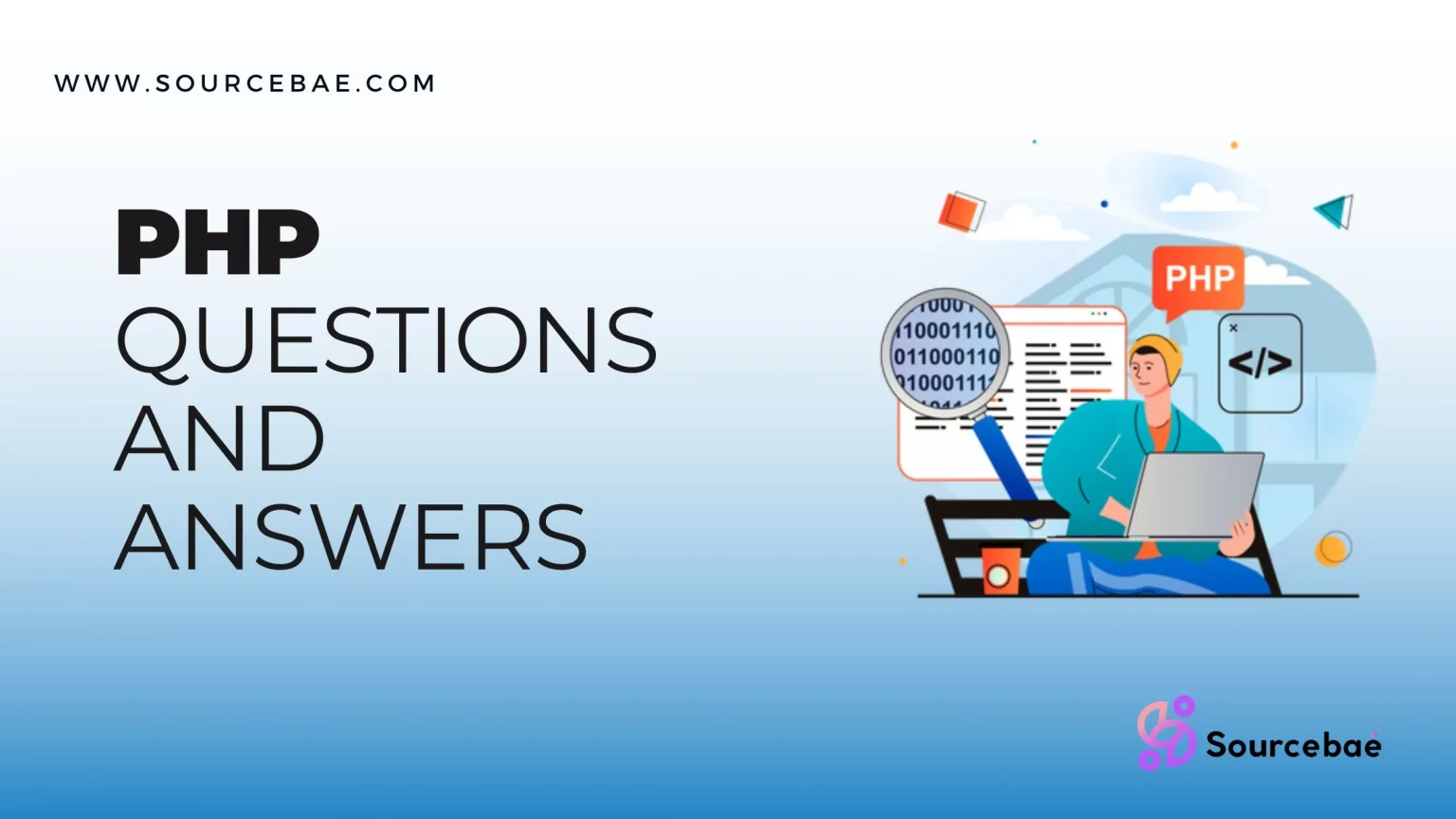
PHP is one of the most popular programming languages in the world, and it is widely used to build dynamic websites and web applications. If you’re looking for a job as a PHP developer, it’s important to be prepared for the PHP technical interview questions that you’ll likely be asked.
In PHP, variables are loosely typed, which means that you don’t need to specify the data type when defining them. However, it’s good practice to explicitly specify the data type when defining a variable.
Examples of different variable types in PHP include:
String: $name = “John”;
Integer: $age = 25;
Float: $price = 24.99;
Boolean: $is_available = true;
It’s important to follow naming conventions while naming variables in PHP, variable names should start with a letter or underscore, followed by any number of letters, numbers, or underscores.
- How does PHP handle errors and exceptions?
PHP has built-in error-handling capabilities that allow you to handle errors and exceptions in your code. Some common error-handling functions in PHP include:
die(): This function is used to stop the execution of a script and display an error message.
trigger_error(): This function is used to raise a custom error message.
set_error_handler(): This function is used to set a custom error handler function that will be called when an error occurs.
In addition to these built-in functions, you can also use try-catch blocks to handle exceptions in your code. A try-catch block looks like this:
try { // code that may throw an exception } catch (Exception $e) { // code to handle the exception }
- How do you connect to a database in PHP?
To connect to a database in PHP, you need to use a database connectivity library or extensions such as MySQLi or PDO. The process of connecting to a database typically involves the following steps:
Connect to the database server using the appropriate function or method.
Select the database you want to connect to.
Authenticate using a valid username and password.
Examples of different functions and libraries used for database connectivity in PHP include:
MySQLi: mysqli_connect()
PDO: new PDO()
It’s best practice to use prepared statements while connecting to the database in PHP to prevent SQL injection attacks.
- How do you perform a query in PHP?
Once you have connected to a database in PHP, you can perform queries on the database using various functions or methods depending on the library or extension you are using.
Examples of different functions and methods used to perform queries in PHP include:
MySQLi: mysqli_query()
PDO: PDO::query()
It’s important to check for errors after performing a query to ensure that the query was successful. You can use functions such as mysqli_error() or PDO::errorInfo() to check for errors.
- How do you create a session in PHP?
In PHP, a session is a way to store data on the server that is accessible to all pages on a website. To create a session, you need to use the session_start() function. This function initializes a new session or resumes an existing one. Once a session is started, you can store data in the $_SESSION superglobal array.
For example:
1
session_start(); $_SESSION[‘username’] = ‘John’;
It’s important to note that the session_start() function must be called before any HTML or output is sent to the browser, otherwise it will not work.
- How do you perform a redirect in PHP?
In PHP, you can perform a redirect using the header() function. This function sends a raw HTTP header to the browser, which can be used to redirect the user to a different page.
For example:
header(‘Location: http://www.example.com‘);
It’s important to note that the header() function must be called before any HTML or output is sent to the browser, otherwise it will not work.
- How do you handle file uploads in PHP?
Handling file uploads in PHP can be done using the $_FILES superglobal array. This array is populated with information about the uploaded files, such as the file name, type, and size. To handle file uploads, you need to make sure that the form that the user is uploading the file from has the attribute “enctype” set to “multipart/form-data”.
For example:
1
Once the form is submitted, you can access the uploaded file using the $_FILES array. You can also use the move_uploaded_file() function to move the uploaded file to a specific location on the server.
- How do you encrypt and decrypt data in PHP?
PHP provides several functions for encrypting and decrypting data, such as hash(), md5(), sha1(), and crypt(). These functions can be used to create one-way hashes of data, which can be used to store passwords or other sensitive information in a secure manner.
For example:
1
$password = “mysecretpassword”; $hashed_password = hash(“sha256”, $password);
PHP also provides functions such as mcrypt_encrypt() and mcrypt_decrypt() for encrypting and decrypting data using symmetric encryption algorithms.
For example:
1
$plaintext = “mysecretdata”; $key = “mysecretkey”; $ciphertext = mcrypt_encrypt(“rijndael-256”, $key, $plaintext, “cbc”);
- How do you debug PHP code?
Debugging PHP code can be done by adding error reporting and debugging code to your scripts. The simplest way to do this is to add the following line of code at the top of your PHP scripts:
1
error_reporting(E_ALL); ini_set(‘display_errors’, 1);
This will display all errors and warnings that occur during the execution of your script. Additionally, you can use the var_dump() function to output the contents of a variable, and the print_r() function to output the contents of an array.
Another option for debugging PHP code is to use a debugger such as Xdebug. This is an extension for PHP that provides advanced debugging features, such as breakpoints and step-by-step execution.
Conclusion:
These are the top 10 PHP interview questions and answers that you should be prepared for when applying for a PHP developer role. Whether you are a fresher or more experienced developer with 2 years, 3 years, 5 years or even 10 years of experience, these questions will help you to showcase your knowledge and skills as a PHP developer.
In this article, we’ll take a look at the top 10 PHP interview questions and answers, whether you’re a fresher or more experienced developer with 2 years experience, 3 years experience or even 10 years of experience.
10 PHP Interview Questions and Answers:
- What is PHP and what are its advantages?
PHP stands for Hypertext Preprocessor and it is a server-side scripting language. It is used to create dynamic websites and web applications. Some of the advantages of using PHP include:
It’s open-source and free to use, which makes it accessible to developers of all levels.
It’s easy to learn, even for developers with little or no programming experience.
It’s compatible with most operating systems and web servers.
It has a large and active community, which means that there are many resources and tutorials available to help you learn and troubleshoot.
Some popular websites that use PHP include Facebook, Wikipedia, and WordPress.
- How do you define a variable in PHP?
In PHP, a variable is defined using the “$” symbol followed by the variable name. For example:
$variable_name = “value”;