Top Eclipse Shortcut Keys & Productivity Hacks for Java Developers
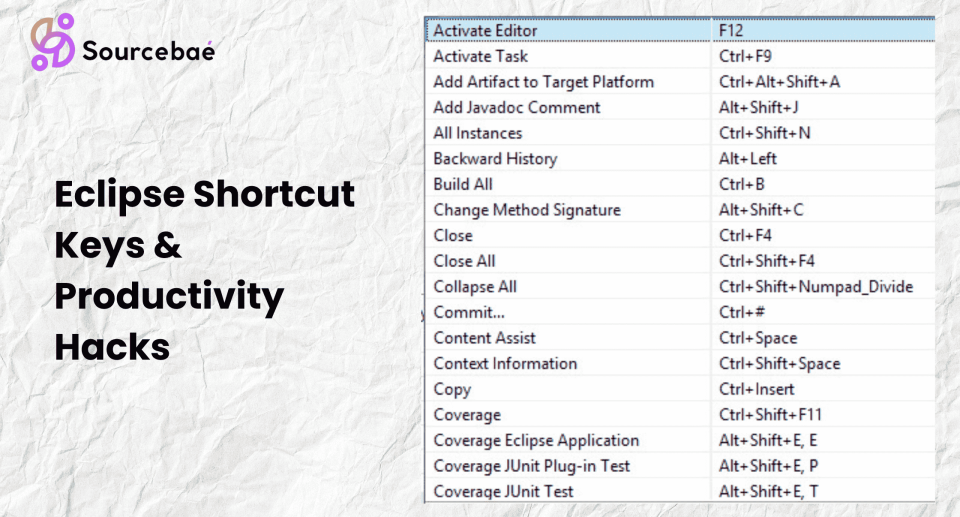
As a Java developer, your Integrated Development Environment (IDE) is more than just a tool—it’s your workspace, laboratory, and canvas. Eclipse, one of the most popular Java IDEs, offers a myriad of features and shortcuts that can significantly enhance your coding efficiency. It helps to streamline coding tasks and boost productivity. Yet, with so many options available, it’s easy to miss out on some lesser-known capabilities. In this post, we’ll explore some of the most useful eclipse shortcut keys and tips to help you code more efficiently and get the most out of Eclipse.
What is Eclipse IDE used for?
Eclipse IDE is a free, open-source development environment primarily known for Java programming but also supports a range of other languages through plugins (like C, C++, JavaScript, and more). It provides tools for writing, debugging, and testing code, as well as features like code completion, syntax highlighting, version control integrations, and a robust plugin ecosystem. Because of its modular structure, developers can customize Eclipse to fit different project requirements, whether they’re building desktop applications, enterprise systems, or web-based solutions.
How to install Eclipse IDE for Java?
1. Check System Requirements
- Operating System: Eclipse runs on Windows, macOS, and Linux.
- Java Development Kit (JDK): Make sure you have a JDK (preferably the latest LTS version, such as Java 17) installed. Eclipse will prompt you for a Java environment if none is detected.
- Memory/Storage: At least 2 GB of RAM and sufficient disk space (around 500 MB or more) are recommended.
Tip: To check if Java is installed, open a terminal or command prompt and type
java -version
orjavac -version
.
2. Download Eclipse
- Visit the Official Eclipse Website
Go to Eclipse Downloads. - Choose the Eclipse Installer or a Specific Package
- You can download the Eclipse Installer, which lets you pick and install a specific Eclipse package (e.g., “Eclipse IDE for Java Developers”).
- Alternatively, you can directly download a ready-to-run zip/tar package (e.g., “Eclipse IDE for Java Developers”) for your operating system.
3. Installation Using the Eclipse Installer
- Run the Eclipse Installer
- After downloading, double-click the installer file (*.exe on Windows, *.dmg on macOS, or *.tar.gz on Linux if you choose the installer version).
- Select ‘Eclipse IDE for Java Developers’
- In the installer window, you’ll see different Eclipse packages. Click the “Eclipse IDE for Java Developers” option.
- Choose Installation Folder and JDK
- The installer will automatically detect your installed JDK.
- Select or confirm the installation folder (where you want Eclipse to be installed).
- Begin Installation
- Click the “Install” button.
- Review and accept any license agreements if prompted.
- Once the installation completes, click “Launch” or open Eclipse from its installation directory.
4. Installation Using the Zip (or Tar) Package
- Download the Appropriate Zip/Tar File
- Look for “Eclipse IDE for Java Developers” in the list of packages for your OS (Windows, macOS, or Linux).
- Extract the Archive
- On Windows, right-click and select Extract All.
- On macOS or Linux, use the built-in archive utility or run a command like
tar -xzvf eclipse-XYZ.tar.gz
.
- Launch Eclipse
- Once extracted, open the eclipse folder.
- Double-click the eclipse (or Eclipse on macOS) executable to start the IDE.
- If prompted, select a workspace folder (where your Eclipse projects and settings will be stored).
5. First-Time Setup
- Workspace Selection
- When you launch Eclipse for the first time, you’ll be asked to select a default workspace.
- You can use the default or choose a custom directory.
- Install Additional Plugins or Tools
- Eclipse has a vast ecosystem of plugins. To explore or add new features, go to Help > Eclipse Marketplace.
- Create a New Java Project
- Go to File > New > Java Project.
- Give your project a name and start coding!
6. Verification
- Check the Installed JRE/JDK
- In Eclipse, go to Window > Preferences (macOS: Eclipse > Preferences) > Java > Installed JREs.
- Ensure the JDK is properly recognized.
- Compile and Run a Sample Program
- Right-click on your
main
class > Run As > Java Application. - Eclipse will compile and run your code, confirming that your environment is set up correctly.
- Right-click on your
Eclipse Shortcut keys:
1. Latest or Lesser-Known Eclipse Shortcuts
1.1 Quick Access (Ctrl + 3)
This is often overlooked but incredibly powerful. Press Ctrl + 3
(Windows/Linux) or Command + 3
(macOS) to quickly search for commands, settings, views, and more. It’s like a command palette that helps you jump to almost anything in Eclipse.
1.2 Show All Key Bindings (Ctrl + Shift + L)
For a quick overview or to customize shortcuts, press Ctrl + Shift + L
. Doing this once displays a tooltip of essential shortcuts. Press it again to open the Keys preferences, where you can set up or modify shortcuts.
1.3 Split Editor and Switch Editor Panes
- Split Editor: In newer Eclipse releases, you can split the editor view by right-clicking on a tab and selecting New Editor or Move to Editor.
- Switch Editor Panes: Use
Ctrl + F6
(orCtrl + Page Up/Page Down
on some OSs) to cycle through open editors and switch between split panes.
1.4 Pin or Detach Views
Recent Eclipse versions allow you to pin specific views like Package Explorer or Console so they remain in place, or detach them into separate windows. This is especially helpful if you’re working with multiple monitors.
1.5 Change Case
- Uppercase:
Ctrl + Shift + X
Select the text you want to convert, then pressCtrl + Shift + X
to transform it to uppercase. - Lowercase:
Ctrl + Shift + Y
Similarly, select your text and pressCtrl + Shift + Y
to transform it to lowercase.
If you don’t see these working, you may need to configure or enable them under Window > Preferences > General > Keys, searching for “Upper Case” and “Lower Case.”
1.6 Autocomplete
- Content Assist (Autocomplete):
Ctrl + Space
This is your main autocomplete shortcut. Eclipse will suggest class names, methods, variables, and code templates based on the current context. For example:- Start typing a class name or method, then press
Ctrl + Space
. - Type a snippet trigger (like
sysout
) and pressCtrl + Space
to expand it intoSystem.out.println()
.
- Start typing a class name or method, then press
1.7 Main Method
- Using the “main” Template
In Eclipse, typemain
and pressCtrl + Space
. Eclipse should offer an option to generate a skeleton of thepublic static void main(String[] args)
method. - Surround Existing Class
If you already have a Java class, place your cursor inside the class but outside any method, typemain
+Ctrl + Space
to insert the main method automatically.
Note: If you don’t see a completion for main
, check your code templates:
- Window > Preferences > Java > Editor > Templates
- Make sure the
main
template is enabled.
1.8 Loops
Eclipse comes with built-in templates for loops. Simply type the keyword (e.g., for
) and press Ctrl + Space
.
- For Loop:
- Type
for
+Ctrl + Space
- Choose from variations like
for - iterate over array
,for - iterate over list
, etc.
- Type
- Enhanced For Loop:
- Type
fore
+Ctrl + Space
to generate afor (ElementType element : collection) { … }
loop quickly.
- Type
- While Loop:
- Type
while
+Ctrl + Space
to generate a template for a standard while loop.
- Type
1.9 Conditional Statements
- If Statement:
- Type
if
+Ctrl + Space
. - You can select from an
if
block or anif-else
block template.
- Type
- Switch Statement:
- Type
switch
+Ctrl + Space
. - Eclipse will offer a basic skeleton of the switch statement.
- Type
- Surround Existing Code:
- If you already have a block of code that you want to place inside an
if
orwhile
, highlight that code and pressAlt + Shift + Z
(Surround with) to choose an appropriate construct.
- If you already have a block of code that you want to place inside an
1.10 Try-Catch Block
- Surround Selected Code:
- Highlight the code you want to wrap in a try-catch.
- Press
Ctrl + 1
(Quick Fix). - Select Surround with try/catch.
- Template:
- Type
try
+Ctrl + Space
. - Eclipse will offer to insert a try-catch or try-finally block automatically.
- For Java 7 and above, you can also generate a “try-with-resources” structure if the code involves resources like streams.
- Type
2. Eclipse Shortcut keys for Code Editing
2.1 Content Assist (Ctrl + Space)
This is your basic autocomplete and suggestion tool. Eclipse will suggest class names, methods, fields, and even templates based on your context.
2.2 Quick Fix (Ctrl + 1)
When you see an error or warning squiggle, place your cursor there and press Ctrl + 1
. Eclipse suggests potential fixes or refactoring’s like importing missing classes or correcting the method signature.
2.3 Surround With Block (Alt + Shift + Z)
If you want to surround a block of code with if
, while
, try/catch
, or other structures, select the lines, press Alt + Shift + Z
, and pick the appropriate option from the list.
2.4 Rename (Alt + Shift + R)
A must-use for refactoring. Whether it’s a variable, method, or class, Eclipse automatically updates all references when you rename.
2.5 Extract Local Variable or Method
- Extract Local Variable:
Alt + Shift + L
- Extract Method:
Alt + Shift + M
These shortcuts streamline your refactoring by pulling out repeated code or large chunks of logic into reusable methods or variables.
2.6 Organize Imports (Ctrl + Shift + O)
Automatically adds missing imports and removes unused ones, keeping your code tidy.
2.7 Auto-Format (Ctrl + Shift + F)
Formats your code according to the style settings configured in Window > Preferences > Java > Code Style.
3. Shortcuts for Quick Navigation
3.1 Open Resource (Ctrl + Shift + R)
Jump to any file in your workspace by typing a partial name. This works for Java files, XML configs, properties files, and more.
3.2 Open Type (Ctrl + Shift + T)
Specifically designed for classes and interfaces. Even if you don’t remember the package name, a partial match will do.
3.3 Quick Outline (Ctrl + O)
Inside an open file, press Ctrl + O
to see a structured outline of the fields and methods. Press Ctrl + O
again for inherited members. This shortcut is great for navigating large classes.
3.4 Go to Declaration (F3 or Ctrl + Click)
Hover over a class, method, or variable and press F3
(or Ctrl + Click
on Windows/Linux). Eclipse will open the file and jump straight to its declaration.
3.5 Navigate Back/Forward (Alt + Left / Alt + Right)
Similar to a web browser, you can jump back to previously viewed locations in the code with Alt + Left Arrow
and go forward with Alt + Right Arrow
.
3.6 Open Call Hierarchy (Ctrl + Alt + H)
View the call hierarchy of a method to see who calls it (and who calls those callers, and so on). This is extremely useful for understanding code flow.
4. Eclipse Shortcut keys for Debugging
4.1 Toggle Breakpoint (Ctrl + Shift + B)
Set or remove a breakpoint on the current line. Alternatively, double-click in the left margin next to the line number.
4.2 Step Through Code (F5 / F6 / F7 / F8)
- Step Into (F5): Enter the method call on the current line.
- Step Over (F6): Move to the next line, skipping the method details.
- Step Return (F7): Exit the current method and go back to the caller.
- Resume (F8): Continue execution until the next breakpoint or the end of the program.
4.3 Inspect/Watch Variables
- Inspect (Ctrl + Shift + I): Highlight a variable or expression in the Debug view, press this shortcut to evaluate it.
- Watch: Right-click an expression/variable and select Watch. Eclipse keeps track of its value as you step through code.
4.4 Display (Ctrl + Shift + D)
While debugging, highlight an expression and press Ctrl + Shift + D
to see its result in the Expressions or Debug console without changing your code.
4.5 Breakpoints View
Open the Breakpoints view (via Window > Show View > Breakpoints) to enable, disable, or remove breakpoints in bulk. You can also set conditions on breakpoints (like stopping only when a variable has a certain value).
Bonus Tip: Customize Your Shortcuts
Eclipse is highly customizable. If a default shortcut conflicts with your muscle memory or personal preference:
- Go to Window > Preferences (macOS: Eclipse > Preferences).
- Expand General and select Keys.
- Search for the command you want to change.
- Assign your own keystroke, press OK, and you’re done!
Final Thoughts
Mastering Eclipse shortcut keys can significantly speed up your workflow, reduce repetitive tasks, and help you maintain focus on solving problems rather than hunting through menus. Start with a few of these shortcuts, gradually incorporate more, and soon you’ll be navigating and editing code with lightning speed.
Got a favorite Eclipse shortcut keys or a hidden gem we didn’t mention? Let us know in the comments! Happy coding!
Read more: Hire Java Developers